Android中的自定义控件与界面优化
发布时间: 2024-01-31 22:58:38 阅读量: 45 订阅数: 41 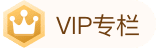
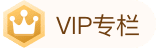
# 1. Android自定义控件概述
## 1.1 自定义控件的概念与作用
在Android开发中,自定义控件是指根据项目需求自行编写的UI组件,能够实现特定的功能或者具有特殊的外观效果。自定义控件可以丰富界面表现形式,提升用户体验,同时也能提高开发效率和代码的可重用性。
## 1.2 Android中自定义控件的分类
在Android中,自定义控件可以分为两类:自定义View和自定义ViewGroup。前者主要用于实现特定功能的UI组件,如按钮、进度条等;后者则用于实现复杂的布局,例如自定义的列表控件、表格布局等。
## 1.3 自定义控件的优势与应用场景
自定义控件能够满足各种个性化的UI需求,使得应用界面更加丰富多彩;同时,通过封装业务逻辑和界面样式,可以提高代码的可维护性和可复用性。自定义控件常应用于产品差异化展示、UI定制化需求等场景中。
接下来将深入探讨如何在Android应用中实现自定义控件,并结合性能优化与用户体验等方面展开讨论。
# 2. 自定义控件的实现与开发
在Android开发中,通过自定义控件可以实现更加灵活和多样化的界面展示。本章将介绍如何使用自定义View来实现简单控件,以及如何使用自定义ViewGroup来实现复杂布局。
### 2.1 使用自定义View实现简单控件
在Android中,自定义View是通过继承View或其子类来实现的。下面以绘制一个自定义的圆形ImageView为例,来介绍自定义View的实现步骤。
首先,创建一个名为CircleImageView的类,继承自ImageView:
```java
public class CircleImageView extends ImageView {
private Paint mPaint;
public CircleImageView(Context context) {
super(context);
init();
}
public CircleImageView(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
public CircleImageView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
private void init() {
mPaint = new Paint();
mPaint.setAntiAlias(true);
mPaint.setDither(true);
}
@Override
protected void onDraw(Canvas canvas) {
Drawable drawable = getDrawable();
if (drawable == null) {
super.onDraw(canvas);
return;
}
Bitmap bitmap = ((BitmapDrawable) drawable).getBitmap();
Bitmap circleBitmap = getCircleBitmap(bitmap);
canvas.drawBitmap(circleBitmap, 0, 0, mPaint);
}
private Bitmap getCircleBitmap(Bitmap bitmap) {
Bitmap output = Bitmap.createBitmap(bitmap.getWidth(), bitmap.getHeight(), Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(output);
final Rect rect = new Rect(0, 0, bitmap.getWidth(), bitmap.getHeight());
final RectF rectF = new RectF(rect);
final float roundRadius = Math.max(bitmap.getWidth(), bitmap.getHeight()) / 2.0f;
mPaint.setShader(new BitmapShader(bitmap, Shader.TileMode.CLAMP, Shader.TileMode.CLAMP));
canvas.drawRoundRect(rectF, roundRadius, roundRadius, mPaint);
return output;
}
}
```
在CircleImageView类中,我们重写了onDraw方法,在该方法内部对View进行绘制操作。在本例中,我们将ImageView的图片绘制为一个圆形。
代码解释:
- 在构造方法和初始化方法中,我们初始化了一个Paint对象,用于绘制圆形图片。
- 在onDraw方法中,我们首先获取ImageView的Drawable对象,判断是否存在。如果不存在,直接调用super方法绘制。
- 接下来,我们将Drawable转换为Bitmap,并使用getCircleBitmap方法将其绘制为圆形后,再调用canvas的drawBitmap方法绘制到View上。
### 2.2 自定义ViewGroup实现复杂布局
除了自定义View,我们还可以通过自定义ViewGroup来实现复杂的布局。下面以一个自定义的流式布局FlowLayout为例,来介绍自定义ViewGroup的实现步骤。
首先,创建一个名为FlowLayout的类,继承自ViewGroup:
```java
public class FlowLayout extends ViewGroup {
public FlowLayout(Context context) {
super(context);
}
public FlowLayout(Context context, AttributeSet attrs) {
super(context, attrs);
}
public FlowLayout(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
int maxWidth = MeasureSpec.getSize(widthMeasureSpec) - getPaddingLeft() - getPaddingRight();
int maxHeight = MeasureSpec.getSize(heightMeasureSpec) - getPaddingTop() - getPaddingBottom();
int childCount = getChildCount();
int lineWidth = 0;
int lineHeight = 0;
int width = 0;
int height = 0;
for (int i = 0; i < childCount; i++) {
View child =
```
0
0
相关推荐
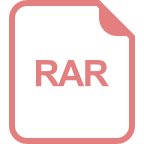
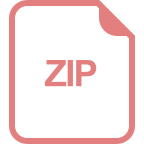
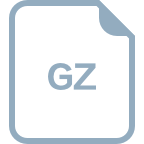
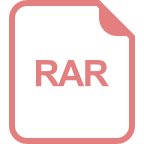
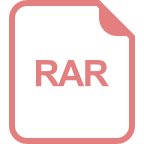
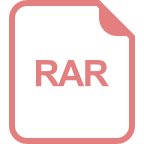