使用Python进行自动文摘提取:掌握算法与实践,提升工作效率
发布时间: 2024-08-31 12:14:56 阅读量: 37 订阅数: 53 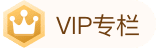
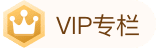
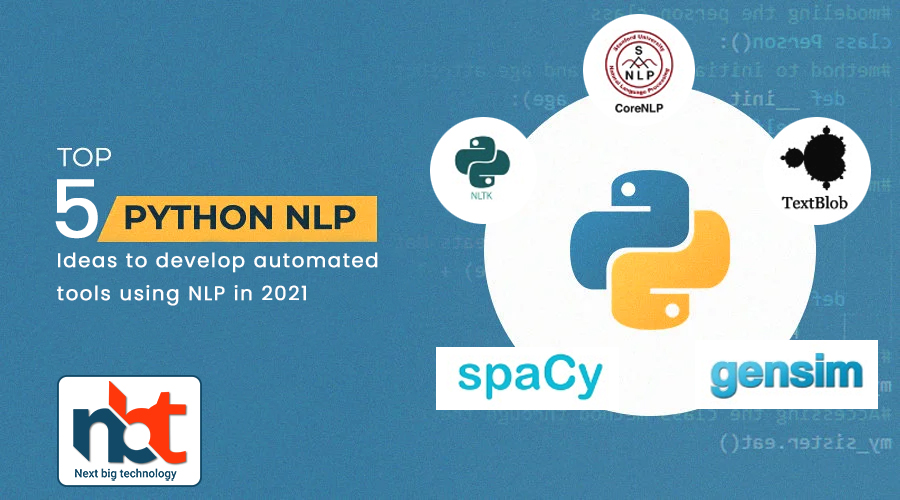
# 1. 自动文摘提取概述
在信息爆炸的时代,自动文摘提取技术已成为IT领域的重要研究方向之一。它允许从大量文本中快速生成简洁、连贯的摘要,极大地提高了信息处理的效率。本章将介绍自动文摘技术的基础概念、发展历程以及它的应用领域。随后,我们将探讨自动文摘系统的主要组成部分,并对文摘提取中的关键技术——文本预处理和特征提取进行概述。通过这些讨论,为读者建立起对自动文摘提取这一技术的初步认识。
# 2. 文本预处理与特征提取
在自动文摘提取的过程中,文本预处理和特征提取是至关重要的步骤。它们为后续的算法分析和模式识别打下基础,确保数据的质量和分析的准确性。本章将详细介绍清洗文本数据、分词和词性标注的方法,以及如何运用特征提取技术如词袋模型、TF-IDF、N-gram模型、主题模型、词嵌入技术和句子嵌入技术来提取文本的关键特征。
### 2.1 文本预处理基础
文本预处理是自然语言处理(NLP)中的一个关键步骤,包括清洗文本数据、分词、词性标注等。这些预处理步骤是去除文本噪声、标准化文本数据,使之成为适合进一步分析的格式。
#### 2.1.1 清洗文本数据
在获取原始文本数据之后,首先要做的是清洗文本数据。这一步骤需要删除无关的信息,如HTML标签、特殊字符、停用词等,以及纠正拼写错误等。清洗过程可以帮助去除数据中的噪声,提高后续分析的准确性。
清洗文本数据的一般步骤包括:
1. 去除HTML标签和特殊字符,如`<br/>`、`&`、`<`等。
2. 移除数字和标点符号,如果它们对于文摘提取不是重要信息。
3. 规范化文本,例如统一为小写,以减少词汇的多样性。
4. 移除或替换掉停用词,即那些在文本中频繁出现但对理解文本内容没有太大帮助的词汇。
5. 分词,如果是中文文本,需要通过中文分词工具进行分词处理。
```python
# 示例代码:清洗文本数据(假设文本是英文)
import re
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
# 定义停用词集合
stop_words = set(stopwords.words('english'))
# 清洗函数
def clean_text(text):
# 移除HTML标签
text = re.sub(r'<.*?>', '', text)
# 移除数字和标点符号
text = re.sub(r'[^\w\s]', '', text)
# 将文本转换为小写
text = text.lower()
# 移除停用词
words = text.split()
words = [word for word in words if word not in stop_words]
return ' '.join(words)
# 示例文本
example_text = "This is a sample text with HTML tags, numbers 123, and punctuation!"
# 调用清洗函数
cleaned_text = clean_text(example_text)
print(cleaned_text)
```
这段代码展示了如何使用Python对文本进行基本的清洗操作。需要注意的是,不同的应用场景可能需要额外的预处理步骤。
#### 2.1.2 分词和词性标注
分词和词性标注是文本预处理的另外两个关键步骤。对于中文文本,分词技术尤为关键,因为中文没有单词间的空格分隔。词性标注则是将每个单词赋予一个词性标签,如名词、动词等,这有助于后续的文本分析。
分词和词性标注的常用工具有jieba(中文分词)、NLTK(自然语言处理工具包)等。
```python
# 示例代码:使用jieba进行中文分词,使用NLTK进行词性标注(假设文本是中文)
import jieba
import nltk
from nltk import pos_tag
nltk.download('averaged_perceptron_tagger')
# 中文文本示例
chinese_text = "自然语言处理是计算机科学和人工智能领域与语言学领域中的一个交汇领域。"
# 使用jieba进行分词
words = jieba.lcut(chinese_text)
# 输出分词结果
print(words)
# 将分词结果转换为英文,然后进行词性标注
english_version = " ".join(words)
tagged_words = pos_tag(english_version.split())
# 输出词性标注结果
print(tagged_words)
```
以上代码展示了如何使用jieba对中文文本进行分词,并将分词结果转换为英文进行词性标注。词性标注结果对于文本的进一步分析非常有用。
### 2.2 特征提取方法
在文本预处理之后,下一步就是从文本数据中提取特征。特征提取是从原始数据中抽取信息的过程,这些信息可作为机器学习模型的输入。常用的特征提取方法包括词袋模型(Bag of Words)、TF-IDF、N-gram模型和主题模型。
#### 2.2.1 词袋模型和TF-IDF
词袋模型(Bag of Words, BoW)是一种将文本转换为数值特征向量的表示方式。在这种表示中,文本被假设为一个“词袋”,其中顺序和上下文被忽略。每个唯一的词对应于特征向量中的一个维度,文档中的每个词都会增加该维度的权重。
TF-IDF(Term Frequency-Inverse Document Frequency)是一种用于信息检索和文本挖掘的常用加权技术。其主要思想是:如果某个词在一篇文章中频繁出现,而在其他文章中很少出现,则认为这个词具有很好的类别区分能力,应该给它较高的权重。
```python
from sklearn.feature_extraction.text import CountVectorizer, TfidfTransformer
# 示例文本集合
documents = [
"The sky is blue.",
"The sun is bright.",
"The sun in the sky is bright.",
"We can see the shining sun, the bright sun."
]
# 创建词袋模型
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(documents)
# 应用TF-IDF转换
tfidf = TfidfTransformer()
X_tfidf = tfidf.fit_transform(X)
# 查看结果
print(X_tfidf.toarray())
```
这段代码展示了如何使用`sklearn`库中的`CountVectorizer`和`TfidfTransformer`类来进行词袋模型表示和TF-IDF加权。
#### 2.2.2 N-gram模型和主题模型
N-gram模型是一种基于统计学的文本特征提取方法。在N-gram模型中,文本被表示为一系列连续的n个词汇的组合。这种方法通常用于捕捉词序信息,有助于提高文本分析的精确度。
主题模型,如潜在语义分析(Latent Semantic Analysis, LSA)和潜在狄利克雷分配(Latent Dirichlet Allocation, LDA),是另一种提取文本数据中隐藏主题的方法。这些模型可以帮助分析大量文本数据中的模式和主题。
```python
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.decomposition import NMF
# 示例文本集合
documents = [
"The sky is blue.",
"The sun is bright.",
"The sun in the sky is bright.",
"We can see the shining sun, the bright sun."
]
# 创建N-gram模型
vectorizer = TfidfVectorizer(ngram_range=(1,2))
X = vectorizer.fit_transform(documents)
# 应用NMF(非负矩阵分解)进行主题建模
nmf_model = NMF(n_components=2, random_state=1)
nmf_transformed = nmf_model.fit_transform(X)
# 查看NMF变换后的特征权重
print(nmf_transformed)
```
在这段代码中,我们使用了`sklearn`的`TfidfVectorizer`来创建一个N-gram特征向量,然后使用非负矩阵分解(NMF)算法对文档进行主题建模,得到每篇文档的主题分布。
### 2.3 高级文本分析技术
随着深度学习的发展,文本分析领域出现了更高级的技术,如词嵌入和句子嵌入。这些方法能够捕捉文本中的语义信息,有助于提高文摘提取的准确性。
#### 2.3.1 词嵌入与Word2Vec
词嵌入是一种将
0
0
相关推荐
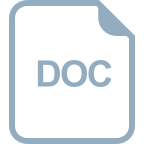
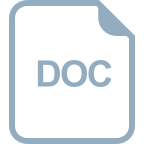
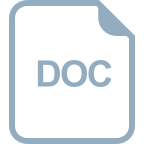
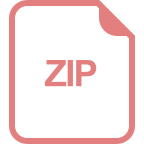
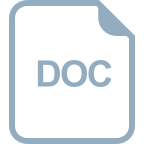
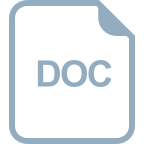
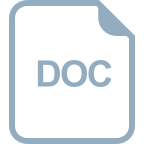
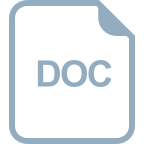
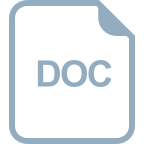