MySQL JSON数据类型详解:深入理解JSON数据存储机制
发布时间: 2024-07-27 19:11:31 阅读量: 27 订阅数: 35 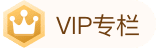
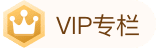
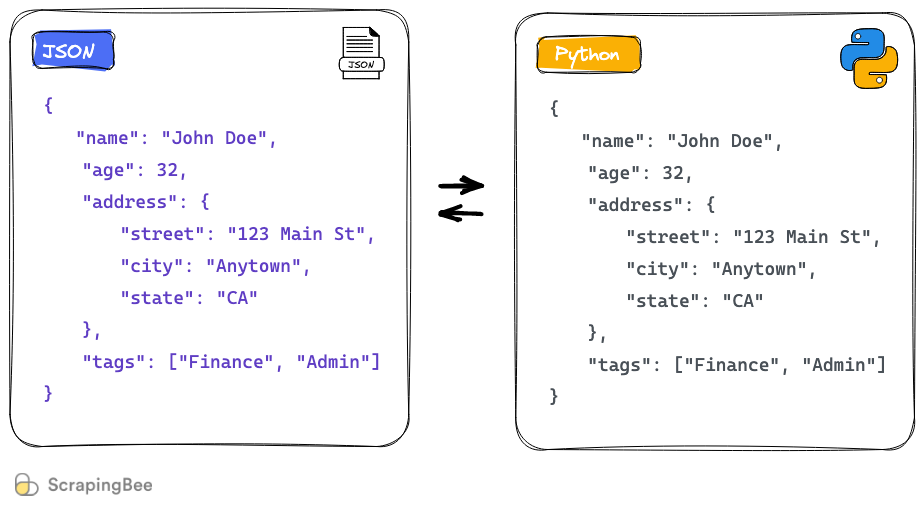
# 1. MySQL JSON数据类型简介**
MySQL JSON数据类型是一种用于存储和管理JSON(JavaScript Object Notation)数据的原生数据类型。JSON是一种轻量级的数据格式,广泛用于Web应用程序和数据交换。MySQL JSON数据类型提供了对JSON数据的强大支持,允许用户以结构化的方式存储和操作复杂的数据。
# 2. JSON数据存储机制**
**2.1 JSON数据结构**
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,它采用文本格式表示数据,具有良好的可读性和可写性。JSON数据结构主要分为两种:
**2.1.1 JSON对象**
JSON对象是一个无序的键值对集合,键是字符串,值可以是任何JSON数据类型,包括字符串、数字、布尔值、数组或其他对象。JSON对象使用花括号`{}`表示,键和值之间使用冒号`:`分隔,键值对之间使用逗号`,`分隔。
```json
{
"name": "John Doe",
"age": 30,
"is_active": true,
"address": {
"street": "123 Main Street",
"city": "Anytown",
"state": "CA",
"zip": "12345"
}
}
```
**2.1.2 JSON数组**
JSON数组是一个有序的值集合,值可以是任何JSON数据类型。JSON数组使用方括号`[]`表示,值之间使用逗号`,`分隔。
```json
[
"John Doe",
30,
true,
{
"street": "123 Main Street",
"city": "Anytown",
"state": "CA",
"zip": "12345"
}
]
```
**2.2 JSON数据存储原理**
MySQL支持两种JSON数据存储机制:文本存储和二进制存储。
**2.2.1 文本存储**
文本存储将JSON数据作为字符串存储在数据库中。这种存储方式简单易用,但查询效率较低,因为MySQL需要对字符串进行解析才能提取数据。
**2.2.2 二进制存储**
二进制存储将JSON数据以二进制格式存储在数据库中。这种存储方式比文本存储更复杂,但查询效率更高,因为MySQL可以直接访问二进制数据。
MySQL默认使用文本存储JSON数据。如果需要使用二进制存储,则需要在创建表时指定`JSON_BINARY`属性。
```sql
CREATE TABLE json_data (
id INT NOT NULL AUTO_INCREMENT,
json_data JSON_BINARY NOT NULL,
PRIMARY KEY (id)
);
```
**存储机制比较**
| 特性 | 文本存储 | 二进制存储 |
|---|---|---|
| 存储方式 | 字符串 | 二进制 |
| 查询效率 | 较低 | 较高 |
| 存储空间 | 较大 | 较小 |
| 复杂性 | 较简单 | 较复杂 |
# 3.1 JSON数据插入
#### 3.1.1 使用JSON_SET()函数
**语法:**
```sql
JSON_SET(json_document, json_path, json_value)
```
**参数说明:**
* `json_document`: 要更新的JSON文档。
* `json_path`: 要更新的JSON路径。
* `json_value`: 要设置的新值。
**代码示例:**
```sql
-- 创建一个JSON文档
INSERT INTO table_name (json_data) VALUES ('{"name": "John", "age": 30}');
-- 使用JSON_SET()函数更新JSON文档
UPDATE table_name SET json_data = JSON_SET(json_data, '$.age', 31);
-- 查询更新后的JSON文档
SELECT json_data FROM table_name;
```
**逻辑分析:**
该代码示例演示了如何使用`JSON_SET()`函数更新JSON文档中的`age`值。`JSON_SET()`函数接受三个参数:要更新的JSON文档、要更新的JSON路径和要设置的新值。在示例中,`json_data`列中的JSON文档被更新,`$.age`路径下的值被设置为31。
#### 3.1.2 使用JSON_INSERT()函数
**语法:**
```sql
JSON_INSERT(json_document, json_path, json_value)
```
**参数说明:**
* `json_document`: 要更新的JSON文档。
* `json_path`: 要插入的新值的位置。
* `json_value`: 要插入的新值。
**代码示例:**
```sql
-- 创建一个JSON文档
INSERT INTO table_name (json_data) VALUES ('{"name": "John", "age": 30}');
-- 使用JSON_INSERT()函数在JSON文档中插入一个新的键值对
UPDATE table_name SET json_data = JSON_INSERT(json_data, '$.hobbies', '["coding", "reading"]');
-- 查询更新后的JSON文档
SELECT json_data FROM table_name;
```
**逻辑分析:**
该代码示例演示了如何使用`JSON_INSERT()`函数在JSON文档中插入一个新的键值对。`JSON_INSERT()`函数接受三个参数:要更新的JSON文档、要插入新值的位置和要插入的新值。在示例中,`json_data`列中的JSON文档被更新,在`$.hobbies`路径下插入了一个新的键值对,值为`["coding", "reading"]`。
# 4. JSON数据索引和优化
### 4.1 JSON索引
**4.1.1 创建JSON索引**
MySQL 5.7及更高版本支持对JSON数据创建索引。创建JSON索引的语法如下:
```sql
CREATE INDEX index_name ON table_name(json_column) USING GIN(json_path)
```
其中:
* `index_name`:索引的名称
* `table_name`:包含JSON列的表名
* `json_column`:要创建索引的JSON列
* `json_path`:JSON路径,指定要索引的JSON对象或数组中的键或元素
例如,创建一个名为 `idx_product_details` 的索引,对 `products` 表中的 `details` JSON列进行索引:
```sql
CREATE INDEX idx_product_details ON products(details) USING GIN(name)
```
**4.1.2 使用JSON索引**
创建JSON索引后,MySQL可以利用索引来优化对JSON数据的查询。例如,以下查询使用 `idx_product_details` 索引来快速查找名称包含 "iPhone" 的产品:
```sql
SELECT * FROM products WHERE details->"$.name" LIKE '%iPhone%'
```
### 4.2 JSON优化
**4.2.1 避免嵌套过深的JSON数据**
嵌套过深的JSON数据会降低查询性能。尽量将JSON数据结构保持扁平化,避免出现多层嵌套。
**4.2.2 使用适当的数据类型**
对于JSON数据中的数值或布尔值,使用适当的数据类型(如 `INT`、`FLOAT`、`BOOL`)可以提高查询效率。
**优化示例**
考虑以下示例:
```sql
CREATE TABLE orders (
id INT NOT NULL,
order_details JSON NOT NULL
);
```
其中,`order_details` 列包含以下JSON数据:
```json
{
"items": [
{
"product_id": 1,
"quantity": 2
},
{
"product_id": 2,
"quantity": 1
}
],
"total_price": 100.00,
"order_date": "2023-03-08"
}
```
为了优化查询,我们可以将 `total_price` 和 `order_date` 从JSON数据中提取出来,并创建单独的列:
```sql
CREATE TABLE orders (
id INT NOT NULL,
total_price FLOAT NOT NULL,
order_date DATE NOT NULL,
order_details JSON NOT NULL
);
INSERT INTO orders (id, total_price, order_date, order_details)
VALUES (1, 100.00, '2023-03-08', '{
"items": [
{
"product_id": 1,
"quantity": 2
},
{
"product_id": 2,
"quantity": 1
}
]
}');
```
通过这种优化,我们可以避免对嵌套的JSON数据进行查询,从而提高查询效率。
# 5. JSON数据案例分析
### 5.1 JSON数据在电商中的应用
JSON数据在电商领域具有广泛的应用,以下列举两个常见的应用场景:
#### 5.1.1 存储产品信息
电商平台需要存储大量产品信息,包括产品名称、描述、规格、价格等。使用JSON数据可以将这些信息以结构化的方式存储在数据库中,方便查询和管理。
```json
{
"product_id": "12345",
"product_name": "iPhone 14 Pro Max",
"description": "6.7英寸Super Retina XDR显示屏,A16仿生芯片,4800万像素主摄",
"specs": {
"storage": "1TB",
"memory": "8GB",
"battery": "4323mAh"
},
"price": 9999
}
```
#### 5.1.2 存储用户订单
电商平台还需要存储用户订单信息,包括订单号、商品列表、收货地址、支付方式等。使用JSON数据可以将这些信息以结构化的方式存储在数据库中,方便查询和处理。
```json
{
"order_id": "20230308123456",
"user_id": "10001",
"items": [
{
"product_id": "12345",
"quantity": 1
},
{
"product_id": "67890",
"quantity": 2
}
],
"shipping_address": {
"name": "张三",
"phone": "13800000000",
"address": "北京市海淀区某某街道某某小区1号楼101室"
},
"payment_method": "微信支付"
}
```
### 5.2 JSON数据在日志分析中的应用
JSON数据在日志分析领域也有着广泛的应用,以下列举两个常见的应用场景:
#### 5.2.1 存储日志事件
日志系统需要记录大量的日志事件,包括事件类型、时间戳、来源、消息等。使用JSON数据可以将这些信息以结构化的方式存储在数据库中,方便查询和分析。
```json
{
"event_type": "error",
"timestamp": "2023-03-08 12:34:56",
"source": "web_server",
"message": "数据库连接失败"
}
```
#### 5.2.2 分析日志数据
日志分析系统需要对日志数据进行分析,以发现问题、优化系统性能等。使用JSON数据可以将日志数据以结构化的方式存储在数据库中,方便使用SQL或其他工具进行查询和分析。
```sql
SELECT event_type, COUNT(*) AS count
FROM logs
WHERE timestamp >= '2023-03-08 00:00:00' AND timestamp <= '2023-03-08 23:59:59'
GROUP BY event_type
ORDER BY count DESC;
```
# 6. JSON数据未来发展**
**6.1 JSON数据在NoSQL数据库中的应用**
JSON数据由于其灵活性和可扩展性,在NoSQL数据库中得到了广泛应用。NoSQL数据库不遵循传统的SQL关系模型,而是采用非结构化或半结构化的数据存储方式,非常适合存储和处理JSON数据。
**6.1.1 MongoDB**
MongoDB是一个流行的NoSQL数据库,以其文档存储模式而闻名。文档是JSON格式的数据结构,可以存储任意数量的键值对。MongoDB提供对JSON数据的强大支持,包括查询、更新和索引功能。
```json
// 创建一个 MongoDB 文档
db.collection.insertOne({
_id: "1",
name: "John Doe",
address: {
street: "123 Main Street",
city: "Anytown",
state: "CA",
zip: "12345"
}
});
// 查询 JSON 数据
db.collection.find({
"address.state": "CA"
});
```
**6.1.2 CouchDB**
CouchDB是另一个流行的NoSQL数据库,专门用于存储和处理JSON数据。它提供了一个RESTful API,允许开发者轻松地创建、读取、更新和删除JSON文档。CouchDB还支持MapReduce功能,用于对JSON数据进行复杂查询和聚合。
```json
// 创建一个 CouchDB 文档
curl -X POST http://localhost:5984/mydb/1 -d '{"name": "John Doe", "address": {"street": "123 Main Street", "city": "Anytown", "state": "CA", "zip": "12345"}}'
// 查询 JSON 数据
curl -X GET http://localhost:5984/mydb/1
```
**6.2 JSON数据在物联网中的应用**
JSON数据在物联网(IoT)中也扮演着重要的角色。物联网设备通常会生成大量传感器数据,这些数据需要以结构化和可处理的方式存储。JSON提供了将传感器数据组织成文档的理想格式,便于存储、传输和分析。
**6.2.1 传感器数据存储**
JSON数据可用于存储各种传感器数据,例如温度、湿度、位置和运动。这些数据可以存储在本地设备上或传输到云平台进行进一步处理。
```json
// 传感器数据示例
{
"device_id": "12345",
"timestamp": "2023-03-08T12:34:56Z",
"data": {
"temperature": 25.5,
"humidity": 60.0,
"location": {
"latitude": 37.422408,
"longitude": 122.084067
}
}
}
```
**6.2.2 设备配置管理**
JSON数据还可用于管理物联网设备的配置。设备配置信息,例如网络设置、安全策略和固件更新,可以存储在JSON文档中,并通过网络传输到设备。
```json
// 设备配置示例
{
"device_id": "12345",
"network": {
"ssid": "my_wifi_network",
"password": "my_wifi_password"
},
"security": {
"username": "admin",
"password": "admin123"
},
"firmware": {
"version": "1.2.3",
"url": "https://example.com/firmware.bin"
}
}
```
0
0
相关推荐
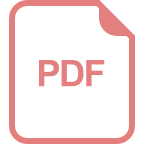
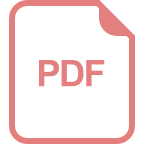
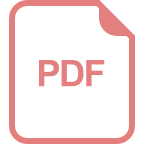





