【JSON数据库入门速成指南】:从零基础到实战应用
发布时间: 2024-08-04 19:08:40 阅读量: 23 订阅数: 29 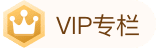
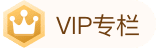
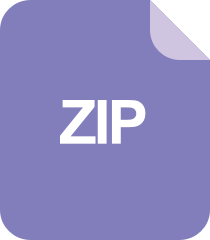
Android Studio开发实战:从零基础到App上线

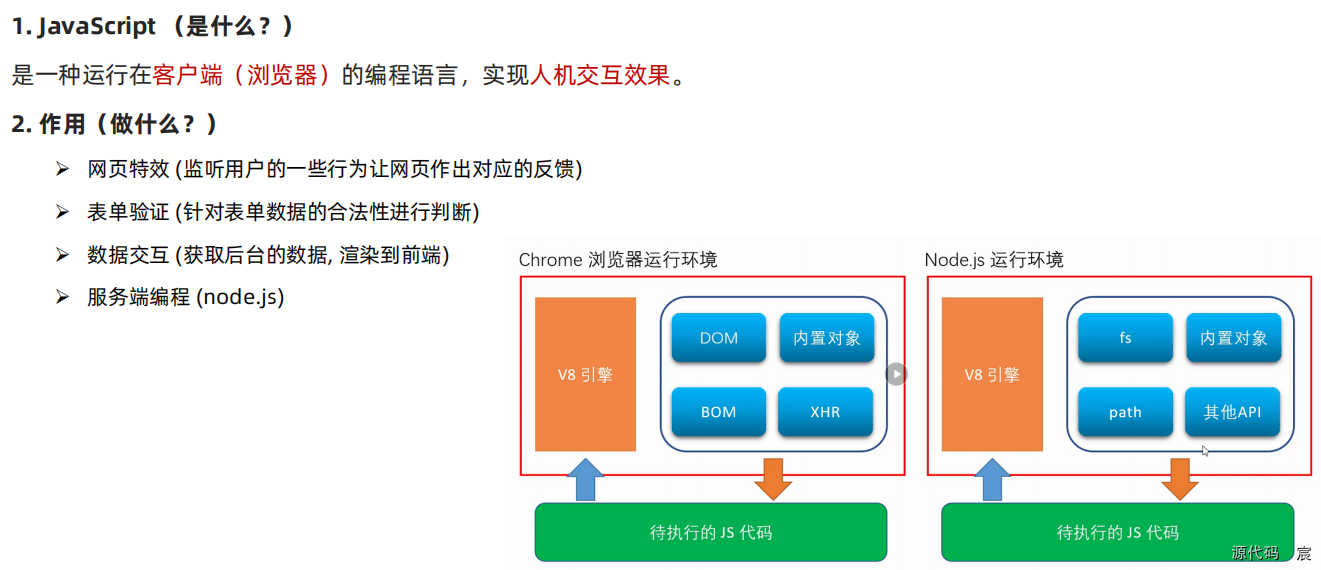
# 1. JSON数据库简介
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,广泛用于Web开发和数据存储。它以文本形式表示数据,并遵循一种类似于JavaScript对象的结构。JSON数据库是一种基于JSON格式存储数据的数据库系统。
与传统关系型数据库不同,JSON数据库采用非结构化数据模型,允许存储灵活且可扩展的数据。这种非结构化特性使得JSON数据库特别适合于处理复杂和多变的数据,例如文档、日志和社交媒体数据。
# 2. JSON数据结构与操作
### 2.1 JSON数据结构概述
#### 2.1.1 JSON对象和数组
JSON数据结构由两种基本类型组成:对象和数组。
- **对象**:由键值对组成,键名是字符串,值可以是任何JSON数据类型。对象用大括号({})表示。
- **数组**:由有序元素列表组成,每个元素可以是任何JSON数据类型。数组用方括号([])表示。
#### 2.1.2 JSON数据类型
JSON支持以下数据类型:
- **字符串**:由双引号包围的文本序列。
- **数字**:整数或浮点数。
- **布尔值**:true或false。
- **null**:表示空值。
- **对象**:如上所述。
- **数组**:如上所述。
### 2.2 JSON数据操作
#### 2.2.1 JSON解析和生成
**解析**将JSON字符串转换为JavaScript对象或数组。**生成**将JavaScript对象或数组转换为JSON字符串。
**解析示例:**
```javascript
const jsonObject = JSON.parse('{ "name": "John Doe", "age": 30 }');
```
**生成示例:**
```javascript
const jsonString = JSON.stringify({ name: "John Doe", age: 30 });
```
#### 2.2.2 JSON数据查询和修改
**查询**:使用点号(.)运算符或方括号([])运算符访问JSON对象或数组中的属性或元素。
**修改**:通过赋值运算符(=)修改JSON对象或数组中的属性或元素。
**查询示例:**
```javascript
console.log(jsonObject.name); // 输出:"John Doe"
```
**修改示例:**
```javascript
jsonObject.age = 31;
```
### 代码块示例
**JSON解析示例:**
```javascript
const jsonString = '{ "name": "John Doe", "age": 30 }';
const jsonObject = JSON.parse(jsonString);
// 逻辑分析:
// JSON.parse() 函数将 JSON 字符串解析为 JavaScript 对象。
// jsonObject 变量现在包含一个具有 name 和 age 属性的对象。
```
### 表格示例
**JSON数据类型对比:**
| 数据类型 | 描述 |
|---|---|
| 字符串 | 由双引号包围的文本序列 |
| 数字 | 整数或浮点数 |
| 布尔值 | true 或 false |
| null | 表示空值 |
| 对象 | 由键值对组成 |
| 数组 | 由有序元素列表组成 |
### mermaid流程图示例
**JSON数据解析流程:**
```mermaid
sequenceDiagram
participant JSONString
participant JavaScriptObject
JSONString->>JavaScriptObject: Parse
JavaScriptObject->>JSONString: Generate
```
# 3. MongoDB数据库实践
### 3.1 MongoDB安装与配置
#### 3.1.1 MongoDB安装
1. **Linux系统安装:**
```bash
sudo apt-get update
sudo apt-get install mongodb
```
2. **Windows系统安装:**
下载MongoDB安装包并执行安装程序。
3. **macOS系统安装:**
```bash
brew install mongodb
```
#### 3.1.2 MongoDB配置
1. **修改配置文件:**
编辑`/etc/mongod.conf`(Linux)或`/usr/local/etc/mongod.conf`(macOS)配置文件,修改以下参数:
```
bind_ip = 0.0.0.0
port = 27017
```
2. **启动MongoDB:**
```bash
sudo service mongod start
```
3. **创建管理员用户:**
```bash
mongo
use admin
db.createUser({user: "admin", pwd: "password", roles: ["root"]})
```
### 3.2 MongoDB数据管理
#### 3.2.1 数据库和集合创建
1. **创建数据库:**
```bash
use my_database
```
2. **创建集合:**
```bash
db.createCollection("my_collection")
```
#### 3.2.2 数据插入和查询
1. **插入数据:**
```bash
db.my_collection.insertOne({name: "John Doe", age: 30})
```
2. **查询数据:**
```bash
db.my_collection.find({name: "John Doe"})
```
### 3.3 MongoDB高级查询
#### 3.3.1 聚合管道
聚合管道允许对数据进行多阶段处理。
**示例:** 计算每个年龄段的人数
```bash
db.my_collection.aggregate([
{$group: {_id: "$age", count: {$sum: 1}}},
{$project: {_id: 0, age: "$_id", count: 1}}
])
```
#### 3.3.2 索引优化
索引可以提高查询速度。
**示例:** 在`name`字段上创建索引
```bash
db.my_collection.createIndex({name: 1})
```
**参数说明:**
- `1`:升序索引
- `-1`:降序索引
# 4. JSON与MongoDB实战应用
### 4.1 JSON数据与MongoDB交互
#### 4.1.1 JSON数据导入和导出
**导入JSON数据**
```json
mongoimport --db <database> --collection <collection> --file <json_file>
```
**参数说明:**
* `--db`: 指定要导入数据的数据库名称。
* `--collection`: 指定要导入数据的集合名称。
* `--file`: 指定要导入的JSON文件路径。
**导出JSON数据**
```json
mongoexport --db <database> --collection <collection> --out <json_file>
```
**参数说明:**
* `--db`: 指定要导出数据的数据库名称。
* `--collection`: 指定要导出数据的集合名称。
* `--out`: 指定要导出的JSON文件路径。
#### 4.1.2 MongoDB数据API操作
MongoDB提供了一系列API用于对数据进行操作,包括:
| API | 描述 |
|---|---|
| `insertOne()` | 插入一个文档 |
| `insertMany()` | 插入多个文档 |
| `updateOne()` | 更新一个文档 |
| `updateMany()` | 更新多个文档 |
| `deleteOne()` | 删除一个文档 |
| `deleteMany()` | 删除多个文档 |
| `find()` | 查询文档 |
| `aggregate()` | 聚合文档 |
**代码示例:**
```javascript
// 插入一个文档
db.collection.insertOne({ name: "John Doe", age: 30 });
// 查询所有文档
db.collection.find({}).toArray((err, docs) => {
console.log(docs);
});
// 聚合文档
db.collection.aggregate([
{ $match: { age: { $gt: 25 } } },
{ $group: { _id: "$name", totalAge: { $sum: "$age" } } }
]).toArray((err, results) => {
console.log(results);
});
```
### 4.2 MongoDB应用场景
MongoDB在各种应用场景中都得到了广泛应用,包括:
#### 4.2.1 文档存储和管理
MongoDB非常适合存储和管理文档数据,因为它提供了灵活的模式和强大的查询功能。文档可以包含各种数据类型,包括JSON对象、数组、字符串、数字和二进制数据。
#### 4.2.2 实时数据分析
MongoDB的聚合管道提供了强大的数据分析功能,可以对大量数据进行实时分析。聚合管道可以执行各种操作,包括过滤、分组、排序、投影和计算。
# 5.1 JSON数据标准与规范
### 5.1.1 JSON Schema
JSON Schema是一种用于定义JSON数据结构和验证的规范。它允许开发者定义JSON数据的预期格式,包括数据类型、键名、嵌套结构和约束条件。
**优点:**
- 提高数据一致性:确保JSON数据符合预定义的结构,减少数据错误和不一致。
- 简化数据验证:通过验证JSON数据是否符合Schema,简化数据处理和集成。
- 增强可读性:提供JSON数据的清晰文档,便于理解和使用。
**使用:**
1. **定义Schema:**使用JSON Schema语言定义JSON数据的结构和约束。
2. **验证数据:**使用JSON Schema验证器检查JSON数据是否符合Schema。
3. **生成文档:**使用JSON Schema生成器生成JSON数据的文档和示例。
### 5.1.2 JSON API
JSON API是一种规范,用于定义基于REST的API,使用JSON作为数据交换格式。它提供了一组标准化的约定,用于定义资源、关系和操作。
**优点:**
- 提高API可预测性:遵循JSON API规范的API具有可预测的URL结构和响应格式。
- 简化客户端开发:提供一致的API交互方式,简化客户端应用程序的开发。
- 增强可扩展性:通过使用标准化的资源和关系定义,API可以轻松地扩展和修改。
**使用:**
1. **定义资源:**使用JSON API资源定义语言定义API中的资源。
2. **定义关系:**使用JSON API关系定义语言定义资源之间的关系。
3. **定义操作:**使用JSON API操作定义语言定义API支持的操作。
0
0
相关推荐
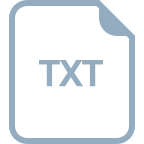
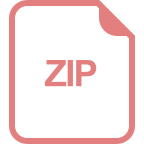
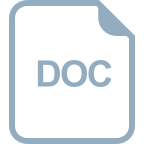
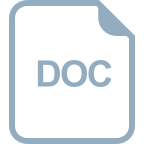
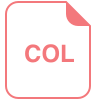
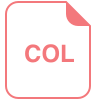
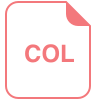
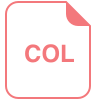