Notepad++ Plugin Recommendations: Expanding Functionality for a Personalized Text Editor
发布时间: 2024-09-14 05:06:15 阅读量: 45 订阅数: 42 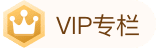
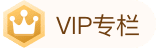
# 1. Introduction and Installation of Notepad++ Plugins
Notepad++ is a lightweight, open-source text editor widely used in programming, web development, and text processing. Its powerful plugin system allows for functionality expansion to meet specific needs.
### Installing Plugins
There are two primary methods for installing Notepad++ plugins:
- **Via the Plugin Manager:** In Notepad++, navigate to the "Plugins" menu and select "Plugin Manager." Enter the plugin name in the search bar and then click the "Install" button.
- **Manual Installation:** Download the plugin's .dll file, copy it to Notepad++'s plugin directory (typically "C:\Program Files (x86)\Notepad++\plugins"). Restart Notepad++ to load the plugin.
# 2. Practical Functions of Notepad++ Plugins
Notepad++ plugins offer a wide range of practical features that greatly enhance its capabilities as a text editor. These features cover various aspects such as code editing, file management, code analysis, and debugging.
### 2.1 Enhanced Code Editing
#### 2.1.1 Syntax Highlighting and Auto-Completion
Syntax highlighting is one of the most basic enhancements provided by Notepad++ plugins. It uses different colors and styles to highlight syntax elements in the code, such as keywords, identifiers, and comments, based on different programming languages or file types. This significantly improves the readability and maintainability of the code.
Auto-completion further enhances the code editing experience. When typing code, Notepad++ plugins suggest code snippets or functions based on the context. This not only saves time but also reduces typing errors.
```cpp
// C++ code example
#include <iostream>
int main() {
// Syntax highlighting will highlight keywords and function names
std::cout << "Hello, world!" << std::endl;
// Auto-completion will suggest cout and endl functions
std::c<TAB>
}
```
#### 2.1.2 Code Folding and Indentation
Code folding allows code blocks to be collapsed, hiding irrelevant details. This is very useful when dealing with large code files, as it can improve the readability and navigability of the code.
The indentation feature automatically formats the code to conform to specific indentation rules. This ensures the neatness and consistency of the code, making it easier to read and understand.
```cpp
// Code folding example
// Click the fold icon to hide code blocks
+ int main() {
| std::cout << "Hello, world!" << std::endl;
|
| // More code
| }
// Indentation example
// Auto-indent code to improve readability
if (condition) {
// Code block 1
} else {
// Code block 2
}
```
### 2.2 Optimized File Management
#### 2.2.1 File Browser and Tab Management
Notepad++ plugins provide an integrated file browser, allowing users to easily browse and open files. The tab management feature enables multiple files to be opened simultaneously and switched between easily, improving work efficiency.
```cpp
// File browser example
// Use the file browser to browse and open files
File > Open > File Browser
// Tab management example
// Use tabs to open multiple files at once
File > New > New Tab
```
#### 2.2.2 File Comparison and Merging
The file comparison plugin allows users to compare the differences between two files. This is very useful during code reviews, version control, or merging different versions of files.
The file merging plugin allows users to merge the contents of two or more files into a new file. This is useful when integrating code or text from different sources.
```cpp
// File comparison example
// Use the file comparison plugin to compare two files
Plugins > Compare > Compare Two Files
// File merging example
// Use the file merging plugin to merge multiple files
Plugins > Merge > Merge Files
```
### 2.3 Code Analysis and Debugging
#### 2.3.1 Static Code Analysis and Error Checking
The static code analysis plugin can scan code and identify potential errors, warnings, and code quality issues. This helps to find problems before running the code, improving code reliability and maintainability.
```cpp
// Static code analysis example
// Use the static code analysis plugin to scan code
Plugins > Code Analysis > Run Analysis
// Error checking example
// Use the error checking plugin to identify errors in the code
Plugins > Error Checker > Check Document
```
#### 2.3.2 Debugging Tools and Breakpoint Settings
Debugging tools plugins allow users to set breakpoints in the code and step through the code execution, checking variable values and code flow. This helps diagnose problems in the code and understand its behavior.
```cpp
// Debugging tools example
// Use the debugging tools plugin to set breakpoints
Debug > Toggle Breakpoint
// Breakpoint settings example
// Set a breakpoint on a specific line
Debug > Break at Line
```
# 3. Customizing Notepad++ Plugins
### 3.1 Customizing the Interface
#### 3.1.1 Themes and Color Schemes
Notepad++ offers a variety of built-in themes and color schemes that users can choose from based on their preferences. These themes and color schemes can change the overall appearance of the editor, including background color, font color, and syntax highlighting colors.
To change the theme, go to "Settings" > "Preferences" > "Style Configurator". In the "Theme" tab, you can preview and select different themes.
To change the color scheme, go to "Settings" > "Preferences" > "Style Configurator". In the "Color Scheme" tab, you can preview and select different color schemes.
#### 3.1.2 Toolbar and Shortcut Keys Customization
Notepad++ allows users to customize toolbars and shortcut keys to improve work efficiency.
To customize the toolbar, right-click the toolbar and select "Customize". In the "Customize Toolbar" dialog, you can add, remove, or rearrange toolbar buttons.
To customize shortcut keys, go to "Settings" > "Preferences" > "Shortcut Mapper". In the "Shortcut Mapper" dialog, you can view and modify existing shortcut keys or create new ones.
### Plugin Management
#### 3.2.1 Installing and Updating Plugins
Notepad++ supports the installation of third-party plugins to extend its functionality. To install a plugin, go to "Plugins" > "Plugin Manager". In the "Plugin Manager", you can search for, install, and update plugins.
#### 3.2.2 Configuring and Disabling Plugins
After installing a plugin, you can configure it by going to "Plugins" > "Plugin Configurator". In the "Plugin Configurator" dialog, you can enable or disable plugins or configure plugin settings.
To disable a plugin, uncheck the box next to the plugin in the "Plugin Manager". To re-enable a plugin, check the box.
### Code Examples
**Customize Toolbar**
```cpp
// Right-click the toolbar and select "Customize"
// In the "Customize Toolbar" dialog, add the "Save" button
toolbar.addButton("Save", "Save", "Ctrl+S")
```
**Customize Shortcut Keys**
```cpp
// Go to "Settings" > "Preferences" > "Shortcut Mapper"
// In the "Shortcut Mapper" dialog, create a new shortcut
shortcuts.add("Toggle Word Wrap", "Ctrl+W")
```
**Configure Plugin**
```cpp
// Go to "Plugins" > "Plugin Configurator"
// In the "Plugin Configurator" dialog, configure the settings for the "TextFX" plugin
textFX.config.enableAutoIndent = true
```
# 4. Practical Application of Notepad++ Plugins
### 4.1 Improving Code Development Efficiency
#### 4.1.1 Code Generation and Templates
Notepad++ offers various code generation and template features, which can significantly improve code development efficiency.
- **Code Generator (Generate):** This plugin allows users to quickly generate code snippets through templates. Users can create their own templates or use predefined template libraries.
```cpp
// Code Generator example
#include <iostream>
int main() {
std::cout << "Hello, world!" << std::endl;
return 0;
}
```
- **Code Templates (Templates):** This plugin allows users to create and manage code templates and insert them effortlessly when needed. Templates can contain code snippets, variables, and comments.
```cpp
// Code Templates example
// Function template
template <typename T>
T max(T a, T b) {
return (a > b) ? a : b;
}
```
#### 4.1.2 Code Refactoring and Optimization
Notepad++ also offers code refactoring and optimization features to help users improve code quality.
- **Code Refactoring (Refactor):** This plugin allows users to rename variables, methods, and classes, extract code blocks, and perform other refactoring operations.
```cpp
// Code Refactoring example
// Rename variable
int old_name = 10;
int new_name = old_name;
```
- **Code Optimization (Optimize):** This plugin can analyze code and suggest optimizations, such as removing unused code, optimizing loops, and reducing memory usage.
```cpp
// Code Optimization example
// Optimize loop
for (int i = 0; i < 10; i++) {
// Perform operation
}
// Optimized version
for (int i = 0; i < 10; ++i) {
// Perform operation
}
```
### 4.2 Automating Text Processing
#### 4.2.1 Text Search and Replace
Notepad++ provides powerful text search and replace functionality, helping users quickly process large amounts of text.
- **Find and Replace:** This plugin allows users to search for and replace specific text strings in the text. It supports regular expressions and advanced search options.
```
// Find and Replace example
Find: Notepad++
Replace: Notepad++ IDE
```
- **Multiline Find and Replace:** This plugin allows users to perform search and replace operations across multiple lines of text.
```
// Multiline Find and Replace example
Find:
```
if (a > 0) {
// Perform operation
}
```
Replace:
```
if (a > 0) {
// Perform operation
} else {
// Perform other operations
}
```
#### 4.2.2 Text Transformation and Formatting
Notepad++ also offers text transformation and formatting features to help users process and organize text data.
- **Text Transformation (Convert Text):** This plugin allows users to convert text into different encodings, line breaks, and file formats.
```
// Text Transformation example
Convert: UTF-8 -> ASCII
```
- **Text Formatting (Format Text):** This plugin can format text, such as indentation, alignment, and removing excessive spaces.
```
// Text Formatting example
Indentation: 4 spaces
```
# 5.1 Basic Plugin Development
### 5.1.1 Plugin Structure and Syntax
Notepad++ plugins are usually provided as `.dll` files, and their internal structure includes:
- **Plugin Header File (plugin.h):** Defines the interface and functionality of the plugin.
- **Plugin Source File (plugin.cpp):** Implements the logic and functionality of the plugin.
- **Resource File (plugin.rc):** Defines resources such as the plugin's icon, menu, and dialog boxes.
The plugin's syntax is based on C++, following this basic structure:
```cpp
#include <npp.h>
// Plugin entry function
BOOL WINAPI DllMain(HINSTANCE hinstDLL, DWORD fdwReason, LPVOID lpvReserved) {
// Plugin initialization code
return TRUE;
}
// Plugin command handling function
BOOL WINAPI CommandFunc(int nCommand, void *nppData, LPARAM lParam) {
// Plugin command handling logic
return TRUE;
}
```
### 5.1.2 Plugin Event Handling and Message Passing
Notepad++ plugins can interact with Notepad++ via event handling and message passing.
**Event Handling:**
Plugins can register event handling functions to respond to events triggered by Notepad++, such as:
```cpp
void OnNotepadppInit() {
// Notepad++ initialization event handling logic
}
```
**Message Passing:**
Plugins can also communicate with Notepad++ by sending and receiving messages, such as:
```cpp
SendMessage(nppData->hwnd, NPPN_GETCURRENTSCINTILLA, 0, 0);
```
By mastering the basics of plugin development, developers can create customized Notepad++ plugins, expanding its functionality and adapting to various needs.
0
0
相关推荐








