Python Requests库:深入剖析HTTP请求处理的秘密
发布时间: 2024-06-23 07:29:44 阅读量: 84 订阅数: 52 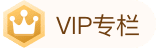
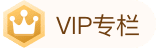
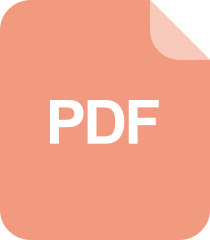
深入Web请求过程

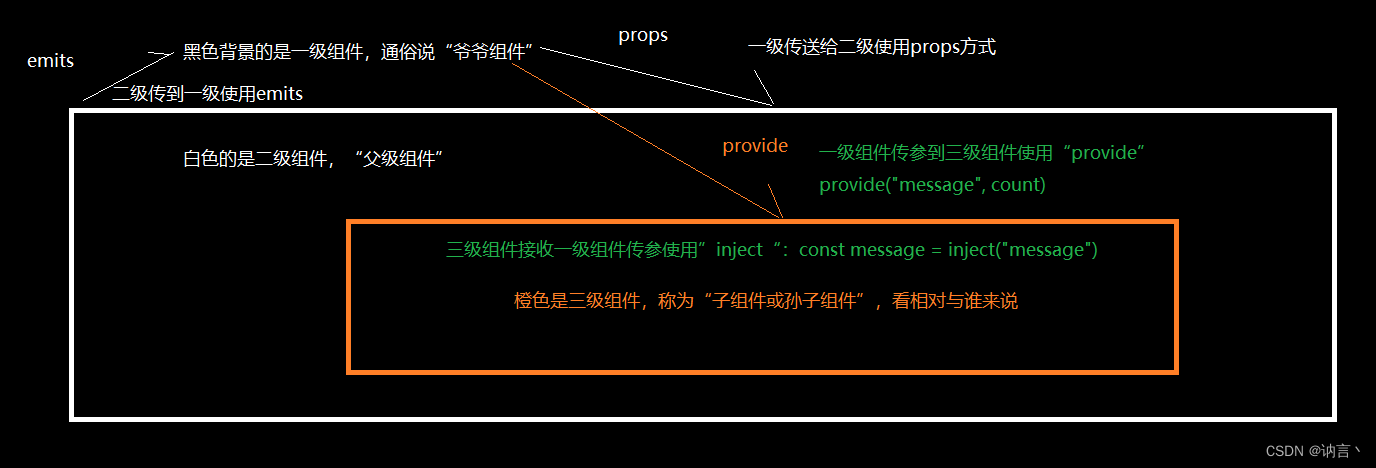
# 1. Python Requests库简介**
Requests库是一个用于Python中发送HTTP请求的强大库。它提供了简洁易用的API,可以轻松地发送各种类型的HTTP请求,包括GET、POST、PUT和DELETE。Requests库还提供了对请求头、请求体和响应的处理,以及对Cookies、会话管理和SSL证书验证的支持。
Requests库的设计目的是使HTTP请求处理变得简单而高效。它具有直观的语法,允许开发人员使用一行代码发送HTTP请求。此外,Requests库还提供了一系列高级特性,如并行请求、异步处理和自定义适配器,使开发人员能够根据自己的需要定制请求处理行为。
# 2. Requests库的基本用法
### 2.1 GET和POST请求
Requests库提供了两种基本类型的HTTP请求:GET和POST。
**GET请求**
GET请求用于从服务器获取数据,其语法如下:
```python
response = requests.get(url, params=None, **kwargs)
```
其中:
- `url`:请求的URL地址
- `params`:一个字典,包含要作为查询参数发送的数据
- `**kwargs`:其他可选参数,如超时、代理等
**POST请求**
POST请求用于向服务器发送数据,其语法如下:
```python
response = requests.post(url, data=None, json=None, **kwargs)
```
其中:
- `url`:请求的URL地址
- `data`:一个字典或字符串,包含要作为表单数据发送的数据
- `json`:一个字典或对象,包含要作为JSON数据发送的数据
- `**kwargs`:其他可选参数,如超时、代理等
### 2.2 请求头和请求体
**请求头**
请求头包含有关请求的信息,如:
- `User-Agent`:客户端的标识符
- `Content-Type`:请求正文的类型
- `Accept`:客户端可以接受的响应类型
**请求体**
请求体包含请求的数据,可以是表单数据、JSON数据或其他类型的数据。
### 2.3 响应处理和错误处理
Requests库提供了多种方法来处理响应和错误:
**响应处理**
- `response.status_code`:响应的状态码
- `response.headers`:响应头
- `response.text`:响应正文(字符串)
- `response.json()`:响应正文(JSON对象)
**错误处理**
- `requests.exceptions.RequestException`:基类异常
- `requests.exceptions.HTTPError`:HTTP错误异常
- `requests.exceptions.ConnectionError`:连接错误异常
- `requests.exceptions.Timeout`:超时异常
**代码示例**
```python
# GET请求
response = requests.get("https://example.com")
print(response.status_code) # 200
print(response.headers) # {'Content-Type': 'text/html; charset=utf-8'}
print(response.text) # HTML文档
# POST请求
data = {"username": "admin", "password": "password"}
response = requests.post("https://example.com/login", data=data)
print(response.status_code) # 200
print(response.json()) # {'success': True, 'token': 'eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9...'}
```
# 3. Requests库的高级特性
### 3.1 Cookies和会话管理
Cookies是服务器发送给客户端的小型数据块,用于在客户端和服务器之间保存状态信息。Requests库提供了对Cookies的全面支持,允许开发者轻松地管理和使用Cookies。
#### Cookies的使用
要使用Cookies,首先需要获取Cookies,可以通过以下方式:
```python
import requests
# 发送GET请求,获取Cookies
response = requests.get("https://example.com")
# 获取响应中的Cookies
cookies = response.cookies
```
获取Cookies后,可以通过以下方式使用:
```python
# 在后续请求中添加Cookies
requests.get("https://example.com", cookies=cookies)
```
#### 会话管理
会话管理是维护客户端和服务器之间会话状态的过程。Requests库通过`Session`对象提供了会话管理功能,允许开发者在多个请求中保持相同的会话状态。
```python
import requests
# 创建一个会话对象
session = requests.Session()
# 在会话中发送GET请求
response = session.get("https://example.com")
# 再次发送GET请求,会话状态将被保持
response = session.get("https://example.com/profile")
```
### 3.2 SSL证书验证
SSL证书用于在客户端和服务器之间建立安全连接。Requests库支持SSL证书验证,以确保连接的安全性和数据的完整性。
#### SSL证书验证的启用
默认情况下,Requests库会验证SSL证书。如果需要禁用证书验证,可以设置`verify`参数为`False`:
```python
import requests
# 禁用SSL证书验证
response = requests.get("https://example.com", verify=False)
```
#### 自签名证书的处理
自签名证书是由组织自己颁发的证书,不受受信任的证书颁发机构(CA)的信任。Requests库可以通过设置`verify`参数为一个自签名证书文件路径来处理自签名证书:
```python
import requests
# 设置自签名证书文件路径
verify_file = "path/to/certificate.pem"
# 使用自签名证书验证SSL连接
response = requests.get("https://example.com", verify=verify_file)
```
### 3.3 代理服务器和身份验证
代理服务器是介于客户端和目标服务器之间的中间服务器。Requests库支持使用代理服务器,并提供身份验证机制。
#### 代理服务器的使用
要使用代理服务器,需要设置`proxies`参数,该参数是一个字典,键为协议(如"http"或"https"),值为代理服务器地址:
```python
import requests
# 设置代理服务器
proxies = {"http": "http://proxy.example.com:8080", "https": "https://proxy.example.com:8080"}
# 使用代理服务器发送GET请求
response = requests.get("https://example.com", proxies=proxies)
```
#### 身份验证
Requests库支持使用HTTP基本身份验证和摘要身份验证。要使用基本身份验证,需要设置`auth`参数为一个元组,第一个元素为用户名,第二个元素为密码:
```python
import requests
# 设置基本身份验证
auth = ("username", "password")
# 使用基本身份验证发送GET请求
response = requests.get("https://example.com", auth=auth)
```
要使用摘要身份验证,需要设置`auth`参数为一个`requests.auth.HTTPBasicAuth`对象:
```python
import requests
# 设置摘要身份验证
auth = requests.auth.HTTPBasicAuth("username", "password")
# 使用摘要身份验证发送GET请求
response = requests.get("https://example.com", auth=auth)
```
# 4. Requests库的实践应用
### 4.1 爬取网页数据
Requests库是爬取网页数据的有力工具。通过使用`get()`方法,我们可以轻松获取网页的HTML内容。例如:
```python
import requests
url = "https://www.example.com"
response = requests.get(url)
html_content = response.text
```
获取HTML内容后,我们可以使用BeautifulSoup或lxml等库来解析和提取所需的数据。
### 4.2 测试API接口
Requests库还非常适合测试API接口。我们可以使用`post()`方法发送请求,并检查响应状态码和内容。例如:
```python
import requests
url = "https://api.example.com/v1/users"
data = {"username": "john", "password": "doe"}
response = requests.post(url, data=data)
if response.status_code == 200:
print("API call successful")
else:
print("API call failed")
```
### 4.3 自动化任务
Requests库可以用于自动化各种任务,例如:
- 定期爬取网站并提取数据
- 监控API接口的可用性和性能
- 发送电子邮件通知或警报
以下是一个示例,展示如何使用Requests库自动化发送电子邮件:
```python
import requests
url = "https://api.example.com/v1/email"
data = {"to": "john@example.com", "subject": "Test email", "body": "This is a test email"}
response = requests.post(url, data=data)
if response.status_code == 200:
print("Email sent successfully")
else:
print("Email sending failed")
```
# 5. Requests库的进阶技巧
### 5.1 并发请求和异步处理
**并发请求**
并发请求允许同时发送多个HTTP请求,从而提高效率。Requests库提供了 `concurrent.futures` 模块来实现并发请求。
```python
import concurrent.futures
def fetch_url(url):
response = requests.get(url)
return response.text
urls = ['https://example.com', 'https://example.org', 'https://example.net']
with concurrent.futures.ThreadPoolExecutor() as executor:
results = executor.map(fetch_url, urls)
for result in results:
print(result)
```
**异步处理**
异步处理允许在不阻塞主线程的情况下发送HTTP请求。Requests库提供了 `aiohttp` 模块来实现异步处理。
```python
import asyncio
async def fetch_url(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
urls = ['https://example.com', 'https://example.org', 'https://example.net']
async def main():
tasks = [fetch_url(url) for url in urls]
results = await asyncio.gather(*tasks)
for result in results:
print(result)
asyncio.run(main())
```
### 5.2 响应流处理和分块下载
**响应流处理**
Requests库提供了 `iter_content()` 方法来以流的方式处理响应内容,从而避免将整个响应内容加载到内存中。
```python
import io
response = requests.get('https://example.com/large_file.txt', stream=True)
with io.TextIOWrapper(response.iter_content()) as f:
for line in f:
print(line)
```
**分块下载**
Requests库提供了 `stream=True` 参数来启用分块下载。这允许在下载文件时逐步处理响应内容,从而避免将整个文件存储在内存中。
```python
import io
response = requests.get('https://example.com/large_file.txt', stream=True)
with io.FileIO('large_file.txt', 'w') as f:
for chunk in response.iter_content(chunk_size=1024):
f.write(chunk)
```
### 5.3 自定义适配器和中间件
**自定义适配器**
Requests库允许使用自定义适配器来连接到HTTP服务器。适配器负责建立和管理HTTP连接。
```python
import requests
class MyAdapter(requests.adapters.BaseAdapter):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
def send(self, request, **kwargs):
# 自定义连接逻辑
pass
requests.adapters.DEFAULT_POOLSIZE = 10
requests.adapters.DEFAULT_RETRIES = 5
requests.adapters.map['https://'] = MyAdapter()
```
**中间件**
Requests库提供了中间件机制,允许在发送和接收HTTP请求时拦截和修改请求和响应。
```python
import requests
class MyMiddleware:
def __init__(self, next):
self.next = next
def __call__(self, request):
# 在发送请求之前执行
request.headers['X-My-Header'] = 'My-Value'
response = self.next(request)
# 在接收响应之后执行
return response
requests.hooks.response.append(MyMiddleware)
```
0
0
相关推荐
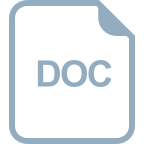
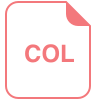
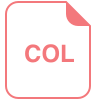
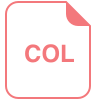
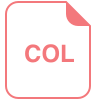
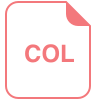