揭秘Python Requests库:从入门到精通的实战宝典
发布时间: 2024-06-23 07:27:52 阅读量: 70 订阅数: 52 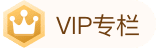
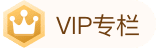
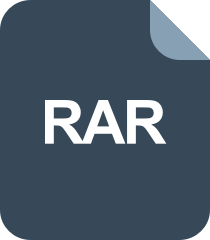
python学习教程(超级详细版)入门到精通
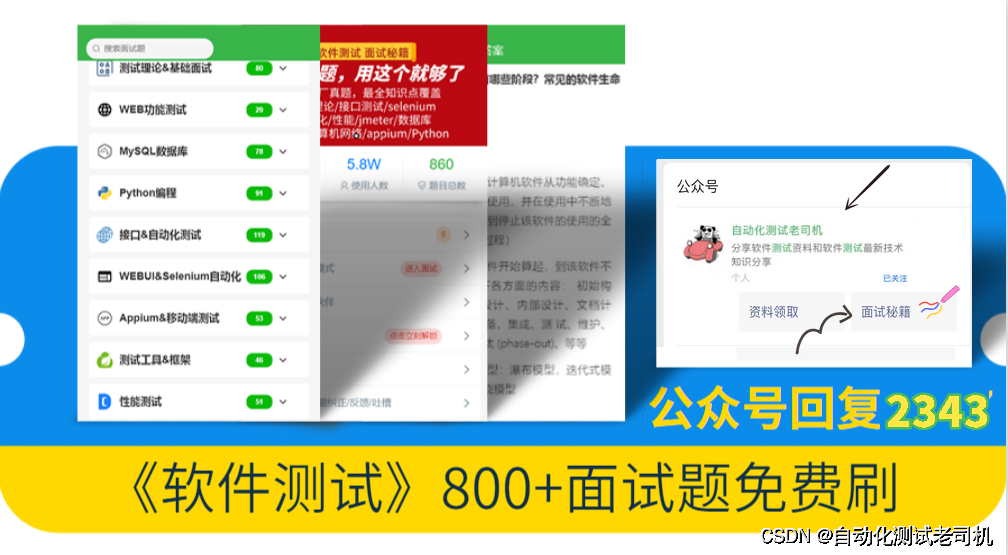
# 1. Python Requests库简介**
Requests库是Python中一个用于发送HTTP请求的强大且易于使用的库。它简化了HTTP请求和响应处理,为开发人员提供了与Web服务交互的便捷方式。Requests库具有以下主要特性:
- **易于使用:**Requests库提供了简洁且直观的API,使发送HTTP请求变得轻而易举。
- **功能丰富:**Requests库支持各种HTTP方法,包括GET、POST、PUT、DELETE等,并提供了对Cookie、认证、代理和超时等功能的全面支持。
- **可扩展性:**Requests库允许开发人员通过扩展和适配器轻松地扩展其功能,以满足特定的需求。
# 2. Requests库基础
### 2.1 HTTP请求与响应
#### 2.1.1 HTTP请求方法
HTTP请求方法用于指定客户端对服务器资源执行的操作。Requests库支持以下HTTP请求方法:
| 方法 | 描述 |
|---|---|
| GET | 从服务器获取资源 |
| POST | 向服务器创建或更新资源 |
| PUT | 更新服务器上的现有资源 |
| DELETE | 从服务器删除资源 |
| HEAD | 获取资源的元数据(如头信息) |
| OPTIONS | 获取服务器支持的HTTP方法 |
#### 2.1.2 HTTP响应状态码
HTTP响应状态码由服务器返回,表示请求处理的结果。Requests库支持以下常见的HTTP响应状态码:
| 状态码 | 描述 |
|---|---|
| 200 | 请求成功 |
| 301 | 永久重定向 |
| 302 | 临时重定向 |
| 400 | 请求语法错误 |
| 401 | 未授权 |
| 403 | 禁止 |
| 404 | 未找到 |
| 500 | 服务器内部错误 |
### 2.2 Requests库的基本用法
#### 2.2.1 GET和POST请求
Requests库可以通过`get()`和`post()`方法发送HTTP请求。`get()`方法用于发送GET请求,而`post()`方法用于发送POST请求。
```python
# 发送GET请求
response = requests.get('https://example.com')
# 发送POST请求
response = requests.post('https://example.com', data={'name': 'John Doe'})
```
#### 2.2.2 参数和数据传递
Requests库支持通过以下方式传递参数和数据:
* **查询参数:**附加到URL末尾,以`?`开头,参数之间用`&`分隔。
* **表单数据:**通过`data`参数传递,以键值对形式组织。
* **JSON数据:**通过`json`参数传递,必须是字典或列表。
```python
# 使用查询参数
response = requests.get('https://example.com?q=python')
# 使用表单数据
response = requests.post('https://example.com', data={'username': 'admin', 'password': 'secret'})
# 使用JSON数据
response = requests.post('https://example.com', json={'name': 'John Doe'})
```
### 2.3 Requests库的会话管理
#### 2.3.1 会话的概念
会话是Requests库中用于维护请求之间状态的对象。它允许在多个请求之间保持cookie、头信息和其他会话数据。
#### 2.3.2 会话的创建和使用
要创建会话,请使用`Session()`类:
```python
session = requests.Session()
```
然后,可以使用会话对象发送请求:
```python
response = session.get('https://example.com')
```
会话对象将自动维护会话数据,并在后续请求中使用它。
# 3. Requests库高级用法
### 3.1 Cookie和认证
#### 3.1.1 Cookie的处理
Cookie是服务器发送给客户端的小型文本文件,用于在客户端和服务器之间存储会话信息。Requests库提供了便捷的方法来处理Cookie。
**获取Cookie**
```python
import requests
# 发送请求
response = requests.get("https://example.com")
# 获取响应中的Cookie
cookies = response.cookies
```
**设置Cookie**
```python
# 创建一个Cookie对象
cookie = requests.cookies.Cookie(
name="my_cookie",
value="my_value",
domain="example.com",
path="/",
expires=datetime.datetime.now() + datetime.timedelta(days=1)
)
# 发送请求时带上Cookie
response = requests.get("https://example.com", cookies=cookie)
```
**清除Cookie**
```python
# 清除所有Cookie
requests.delete("https://example.com", allow_redirects=False)
# 清除特定Cookie
response = requests.get("https://example.com", cookies={"my_cookie": ""})
```
#### 3.1.2 认证机制
Requests库支持多种认证机制,包括基本认证、摘要认证和令牌认证。
**基本认证**
```python
# 发送请求时带上用户名和密码
response = requests.get("https://example.com", auth=("username", "password"))
```
**摘要认证**
```python
# 发送请求时带上用户名和密码
response = requests.get("https://example.com", auth=("username", "password"), auth_type=requests.auth.HTTPBasicAuth)
```
**令牌认证**
```python
# 发送请求时带上令牌
response = requests.get("https://example.com", headers={"Authorization": "Bearer my_token"})
```
### 3.2 代理和超时
#### 3.2.1 代理的使用
代理服务器可以作为客户端和目标服务器之间的中介,用于隐藏客户端的真实IP地址或绕过防火墙。Requests库支持使用代理服务器。
```python
# 使用HTTP代理
proxy = {"http": "http://proxy.example.com:8080"}
response = requests.get("https://example.com", proxies=proxy)
# 使用SOCKS代理
proxy = {"socks5": "socks5://proxy.example.com:1080"}
response = requests.get("https://example.com", proxies=proxy)
```
#### 3.2.2 超时设置
Requests库允许设置请求和响应的超时时间。
```python
# 设置请求超时时间(以秒为单位)
timeout = (3.05, 10)
response = requests.get("https://example.com", timeout=timeout)
```
### 3.3 请求和响应的定制
#### 3.3.1 请求头和请求体
Requests库允许定制请求头和请求体。
**请求头**
```python
# 设置请求头
headers = {"Content-Type": "application/json"}
response = requests.get("https://example.com", headers=headers)
```
**请求体**
```python
# 设置请求体
data = {"username": "my_username", "password": "my_password"}
response = requests.post("https://example.com", data=data)
```
#### 3.3.2 响应头和响应体
Requests库提供了访问响应头和响应体的方法。
**响应头**
```python
# 获取响应头
headers = response.headers
```
**响应体**
```python
# 获取响应体
content = response.content
# 获取响应体文本
text = response.text
# 获取响应体JSON数据
json_data = response.json()
```
# 4. Requests库实战应用
### 4.1 数据抓取
#### 4.1.1 网页抓取
**代码块:**
```python
import requests
from bs4 import BeautifulSoup
# GET请求网页
url = "https://www.example.com"
response = requests.get(url)
# 解析HTML
soup = BeautifulSoup(response.text, "html.parser")
# 提取网页标题
title = soup.find("title").text
print(title)
```
**逻辑分析:**
* 使用`requests.get()`方法发送GET请求到目标网址。
* 将响应内容存储在`response`对象中。
* 使用`BeautifulSoup`库解析HTML内容。
* 通过`find()`方法找到`<title>`标签并提取其文本内容。
#### 4.1.2 API调用
**代码块:**
```python
import requests
# POST请求API
url = "https://api.example.com/endpoint"
data = {"key": "value"}
response = requests.post(url, data=data)
# 解析JSON响应
json_data = response.json()
print(json_data)
```
**逻辑分析:**
* 使用`requests.post()`方法发送POST请求到API端点。
* 将请求数据存储在`data`字典中。
* 将响应内容存储在`response`对象中。
* 使用`json()`方法将响应内容解析为JSON数据。
### 4.2 数据处理
#### 4.2.1 JSON解析
**代码块:**
```python
import requests
import json
# GET请求JSON数据
url = "https://api.example.com/data"
response = requests.get(url)
# 解析JSON数据
json_data = json.loads(response.text)
print(json_data)
```
**逻辑分析:**
* 使用`requests.get()`方法发送GET请求到JSON数据端点。
* 将响应内容存储在`response`对象中。
* 使用`json.loads()`方法将响应内容解析为JSON数据。
#### 4.2.2 XML解析
**代码块:**
```python
import requests
from xml.etree import ElementTree
# GET请求XML数据
url = "https://api.example.com/data.xml"
response = requests.get(url)
# 解析XML数据
root = ElementTree.fromstring(response.text)
print(root.tag)
```
**逻辑分析:**
* 使用`requests.get()`方法发送GET请求到XML数据端点。
* 将响应内容存储在`response`对象中。
* 使用`ElementTree`库解析XML内容。
* 获取根元素并打印其标签名称。
### 4.3 自动化测试
#### 4.3.1 单元测试
**代码块:**
```python
import unittest
import requests
class TestRequests(unittest.TestCase):
def test_get_request(self):
url = "https://www.example.com"
response = requests.get(url)
self.assertEqual(response.status_code, 200)
```
**逻辑分析:**
* 使用`unittest`框架创建测试用例类。
* 定义一个测试方法来测试GET请求。
* 断言响应状态码为200,表示请求成功。
#### 4.3.2 集成测试
**代码块:**
```python
import unittest
import requests
class TestRequestsIntegration(unittest.TestCase):
def test_api_call(self):
url = "https://api.example.com/endpoint"
data = {"key": "value"}
response = requests.post(url, data=data)
self.assertEqual(response.json()["status"], "success")
```
**逻辑分析:**
* 定义一个集成测试用例类。
* 定义一个测试方法来测试API调用。
* 断言响应JSON数据中的"status"字段值为"success",表示API调用成功。
# 5. Requests库进阶**
**5.1 并发和异步请求**
**5.1.1 多线程和多进程**
在某些场景下,我们需要同时处理多个请求以提高效率。Requests库支持使用多线程或多进程来实现并发请求。
**多线程**
```python
import requests
import threading
def fetch_url(url):
response = requests.get(url)
print(f"Thread {threading.current_thread().name} fetched {url}")
urls = ["https://www.google.com", "https://www.amazon.com", "https://www.facebook.com"]
threads = []
for url in urls:
thread = threading.Thread(target=fetch_url, args=(url,))
threads.append(thread)
for thread in threads:
thread.start()
for thread in threads:
thread.join()
```
**逻辑分析:**
* 创建一个 `fetch_url` 函数,该函数使用Requests库获取给定URL的响应。
* 定义一个包含要获取的URL列表。
* 创建一个空列表 `threads` 来存储线程。
* 遍历URL列表,为每个URL创建一个线程,并将其添加到 `threads` 列表中。
* 启动所有线程。
* 等待所有线程完成。
**多进程**
```python
import requests
import multiprocessing
def fetch_url(url):
response = requests.get(url)
print(f"Process {multiprocessing.current_process().name} fetched {url}")
urls = ["https://www.google.com", "https://www.amazon.com", "https://www.facebook.com"]
processes = []
for url in urls:
process = multiprocessing.Process(target=fetch_url, args=(url,))
processes.append(process)
for process in processes:
process.start()
for process in processes:
process.join()
```
**逻辑分析:**
* 与多线程类似,创建一个 `fetch_url` 函数来获取URL响应。
* 定义一个包含要获取的URL列表。
* 创建一个空列表 `processes` 来存储进程。
* 遍历URL列表,为每个URL创建一个进程,并将其添加到 `processes` 列表中。
* 启动所有进程。
* 等待所有进程完成。
**5.1.2 异步请求**
Requests库还支持异步请求,这对于处理大量并发请求非常有用。异步请求允许程序在等待请求响应的同时继续执行其他任务。
**使用 `aiohttp` 库**
```python
import asyncio
import aiohttp
async def fetch_url(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
print(f"Fetched {url} with status code {response.status}")
urls = ["https://www.google.com", "https://www.amazon.com", "https://www.facebook.com"]
async def main():
tasks = [fetch_url(url) for url in urls]
await asyncio.gather(*tasks)
asyncio.run(main())
```
**逻辑分析:**
* 使用 `aiohttp` 库的 `ClientSession` 和 `get` 方法进行异步请求。
* 定义一个 `fetch_url` 协程函数来获取URL响应。
* 定义一个 `main` 协程函数来创建所有异步任务。
* 使用 `asyncio.gather` 等待所有任务完成。
* 使用 `asyncio.run` 运行 `main` 协程。
**5.2 Requests库的扩展**
**5.2.1 扩展库**
Requests库提供了许多扩展库,可以增强其功能。例如:
* `requests-cache`:用于缓存请求响应。
* `requests-oauthlib`:用于OAuth认证。
* `requests-html`:用于解析HTML响应。
**5.2.2 自定义适配器**
Requests库允许创建自定义适配器来处理特定的传输协议。例如,我们可以创建一个自定义适配器来使用Tor代理。
```python
import requests
class TorAdapter(requests.adapters.HTTPAdapter):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.tor_proxy = "socks5://127.0.0.1:9050"
def send(self, request, **kwargs):
kwargs["proxies"] = {"http": self.tor_proxy, "https": self.tor_proxy}
return super().send(request, **kwargs)
session = requests.Session()
session.mount("http://", TorAdapter())
session.mount("https://", TorAdapter())
response = session.get("https://example.com")
```
**逻辑分析:**
* 创建一个自定义适配器类 `TorAdapter`,继承自 `requests.adapters.HTTPAdapter`。
* 在 `__init__` 方法中,设置Tor代理地址。
* 在 `send` 方法中,将Tor代理添加到请求的代理设置中。
* 创建一个Requests会话并挂载自定义适配器。
* 使用会话发送请求,该请求将通过Tor代理。
# 6. Requests库最佳实践**
**6.1 性能优化**
**6.1.1 缓存机制**
缓存机制可以有效减少对远程服务器的请求次数,提高请求响应速度。Requests库提供了内置的缓存机制,可以通过设置 `cache_control` 参数来启用:
```python
import requests
# 创建一个带有缓存机制的会话
session = requests.Session()
session.cache_control = True
# 发送请求
response = session.get('https://example.com')
# 检查缓存状态
if response.from_cache:
print('响应来自缓存')
else:
print('响应来自远程服务器')
```
**6.1.2 负载均衡**
负载均衡可以将请求分布到多个服务器上,避免单点故障并提高整体性能。Requests库支持使用 `adapters` 参数指定负载均衡器:
```python
import requests
from requests.adapters import HTTPAdapter
# 创建一个带有负载均衡器的会话
session = requests.Session()
session.mount('https://example.com', HTTPAdapter(max_retries=3))
# 发送请求
response = session.get('https://example.com')
```
**6.2 安全实践**
**6.2.1 防御XSS攻击**
XSS(跨站脚本)攻击是一种注入恶意脚本代码到网页中的攻击方式。Requests库提供了 `html` 参数,可以自动转义 HTML 响应中的特殊字符,防止 XSS 攻击:
```python
import requests
# 创建一个带有 XSS 防御的会话
session = requests.Session()
session.html = True
# 发送请求
response = session.get('https://example.com')
# 检查 HTML 转义
print(response.text)
```
**6.2.2 防御CSRF攻击**
CSRF(跨站请求伪造)攻击是一种利用受害者身份发起未经授权请求的攻击方式。Requests库提供了 `verify` 参数,可以验证服务器响应中的 SSL 证书,防止 CSRF 攻击:
```python
import requests
# 创建一个带有 CSRF 防御的会话
session = requests.Session()
session.verify = True
# 发送请求
response = session.get('https://example.com')
# 检查 SSL 证书验证
if response.verify:
print('SSL 证书验证成功')
else:
print('SSL 证书验证失败')
```
0
0
相关推荐
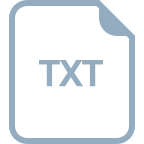
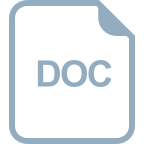
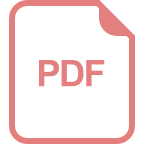
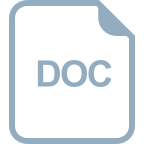
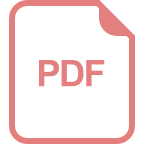
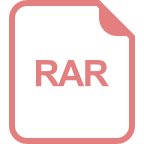
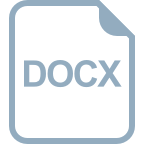