分布式并发编程中的 ZooKeeper:分布式协调服务的利器,管理分布式系统中的复杂性
发布时间: 2024-08-26 11:54:20 阅读量: 7 订阅数: 18 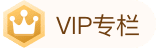
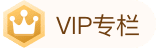
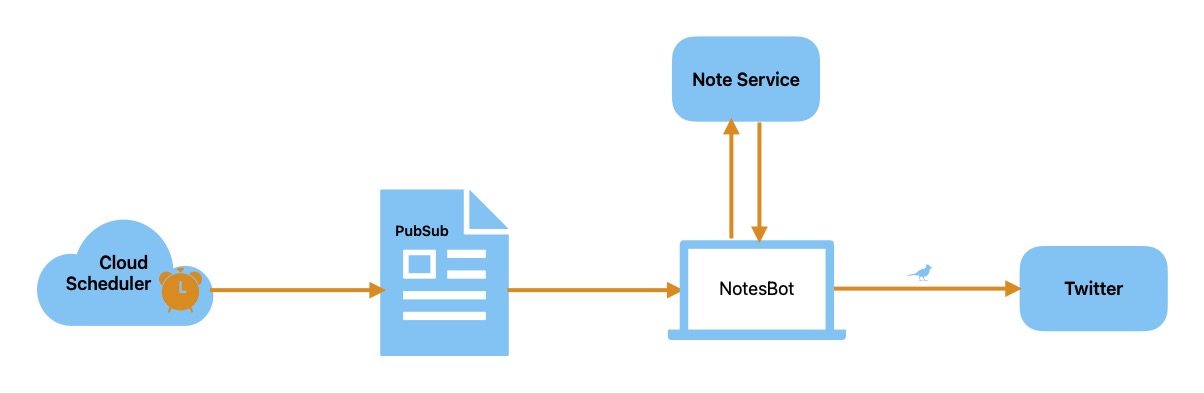
# 1. 分布式并发编程的挑战
分布式并发编程是一种编程范式,涉及在多台计算机上并行执行任务。与单机编程相比,分布式并发编程面临着独特的挑战,包括:
* **数据一致性:**确保分布在不同机器上的数据在所有节点上保持一致。
* **并发控制:**协调对共享资源的访问,以防止数据损坏或死锁。
* **容错性:**处理节点故障或网络中断等异常情况,以确保系统继续运行。
# 2. ZooKeeper 的分布式协调服务
### 2.1 ZooKeeper 的架构和特性
#### 2.1.1 ZooKeeper 的集群结构
ZooKeeper 采用集群结构,由多个服务器节点组成,其中一个节点为主节点(Leader),其余节点为从节点(Follower)。主节点负责处理客户端请求并同步数据到从节点,而从节点负责备份数据并随时准备接替主节点。
#### 2.1.2 ZooKeeper 的数据模型
ZooKeeper 使用分层数据模型,类似于文件系统。数据存储在节点中,节点可以有子节点,形成树状结构。每个节点都存储一个数据值,可以是任意字节数组。
### 2.2 ZooKeeper 的基本操作
#### 2.2.1 创建和删除节点
```java
// 创建节点
zk.create("/myNode", "myData".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT);
// 删除节点
zk.delete("/myNode");
```
#### 2.2.2 读写节点数据
```java
// 读取节点数据
byte[] data = zk.getData("/myNode", false, null);
// 写入节点数据
zk.setData("/myNode", "newData".getBytes(), -1);
```
#### 2.2.3 监听节点变化
```java
zk.exists("/myNode", new Watcher() {
@Override
public void process(WatchedEvent event) {
// 节点变化处理逻辑
}
});
```
# 3.1 分布式锁
#### 3.1.1 分布式锁的原理
分布式锁是一种在分布式系统中协调多个进程或线程对共享资源的访问的机制。它确保在任何给定时间,只有一个进程或线程可以访问该资源。
分布式锁的原理通常基于以下思想:
- **互斥性:**确保在任何给定时间,只有一个进程或线程可以持有锁。
- **容错性:**即使系统中出现故障,锁机制也必须能够正常工作。
- **可扩展性:**锁机制应该能够在系统规模不断增长的同时保持有效。
#### 3.1.2 ZooKeeper 实现分布式锁
ZooKeeper 可以通过使用 **临时顺序节点** 来实现分布式锁。临时顺序节点是一种特殊的 ZooKeeper 节点,它会在创建后自动删除,并且在创建时会自动分配一个唯一的顺序号。
**获取锁:**
1. 每个进程或线程创建一个临时顺序节点。
2. 获取所有临时顺序节点的列表,并按顺序号排序。
3. 如果当前进程或线程创建的节点具有最小的顺序号,则它获得了锁。
**释放锁:**
1. 当前进程或线程删除其创建的临时顺序节点。
2. 其他进程或线程将重新获取临时顺序节点的列表,并检查是否获得锁。
**代码示例:**
```java
import org.apache.zookeeper.KeeperException;
import org.apache.zookeeper.WatchedEvent;
import org.apache.zookeeper.Watcher;
import org.apache.zookeeper.ZooKeeper;
import org.apache.zookeeper.data.Stat;
import java.io.IOException;
import java.util.Collections;
import java.util.List;
public class DistributedLock {
private ZooKeeper zooKeeper;
private String lockPath;
public DistributedLock(String lockPath) throws IOException {
this.lockPath = lockPath;
zooKeeper = new ZooKeeper("localhost:2181", 3000, new Watcher() {
@Override
public void process(WatchedEvent event) {
// TODO: Handle ZooKeeper events
}
});
}
public void acquireLock() throws KeeperException, InterruptedException {
// 创建临时顺序节点
String nodePath = zooKeeper.create(lockPath + "/lock-", null, ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL_SEQUENTIAL);
// 获取所有临时顺序节点的列表
List<String> children = zooKeeper.getChildren(lockPath, false);
// 按顺序号排序
Collections.sort(children);
// 检查当前节点是否具有最小的顺序号
if (nodePath.equals(lockPath + "/" + children.get(0))) {
System.out.println("获取锁成功");
} else {
// 监
```
0
0
相关推荐
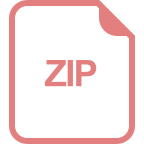
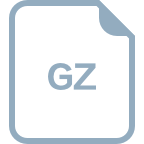
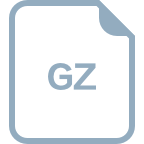





