揭秘多线程编程实战:掌握并发编程基石,提升代码性能
发布时间: 2024-08-26 11:16:37 阅读量: 9 订阅数: 18 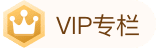
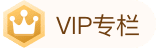
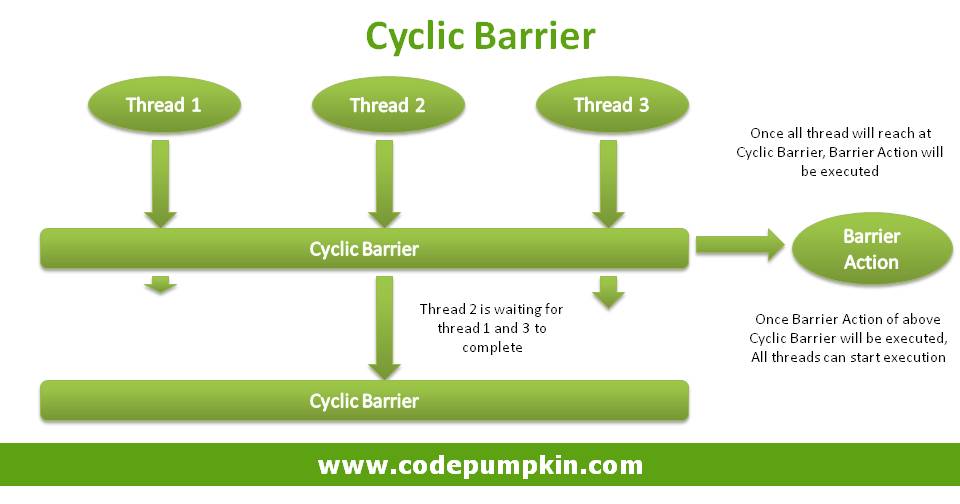
# 1. 多线程编程概述**
多线程编程是一种编程范式,它允许一个程序同时执行多个任务。通过创建和管理多个线程,程序可以充分利用多核处理器的优势,提高程序的执行效率。多线程编程广泛应用于各种领域,例如操作系统、Web服务器、并行计算等。
多线程编程的关键概念包括:
- **并发:**多个任务同时执行,但它们共享相同的地址空间和资源。
- **并行:**多个任务同时执行,但它们拥有独立的地址空间和资源。
# 2. 多线程编程理论基础
### 2.1 并发与并行
**并发**是指多个任务在同一时间段内交替执行,但它们并不是同时执行的。并发通过时间片轮转机制,让多个任务交替使用CPU资源,从而给用户一种同时执行的错觉。
**并行**是指多个任务真正同时执行,需要多核CPU或多处理器系统。并行可以显著提高程序的执行效率,但实现难度也更大。
### 2.2 线程的概念与生命周期
**线程**是进程中的一个执行单元,它拥有自己的程序计数器、栈和局部变量。线程与进程的区别在于,线程共享进程的地址空间和全局变量。
**线程生命周期**包括以下几个阶段:
- **创建:**线程被创建并分配资源。
- **就绪:**线程等待被调度执行。
- **运行:**线程正在执行。
- **阻塞:**线程由于等待资源而无法执行。
- **终止:**线程执行完毕或被终止。
### 2.3 线程同步与互斥
**线程同步**是指协调多个线程的执行,确保它们按预期顺序执行。常用的线程同步机制包括:
- **锁:**锁是一种互斥机制,一次只能允许一个线程访问共享资源。
- **信号量:**信号量是一种计数器,用于限制同时访问共享资源的线程数量。
- **条件变量:**条件变量用于等待某个条件满足,然后唤醒等待的线程。
**互斥**是指确保共享资源在同一时间只能被一个线程访问。互斥机制可以防止数据竞争和程序崩溃。
### 2.4 死锁与饥饿
**死锁**是指多个线程相互等待对方释放资源,导致所有线程都无法继续执行。死锁通常发生在多个线程竞争同一组资源时。
**饥饿**是指某个线程长时间无法获得资源,导致其无法执行。饥饿通常发生在线程优先级不合理或资源分配不公平时。
**代码示例:**
```python
import threading
def worker(lock):
with lock:
# 访问共享资源
lock = threading.Lock()
threads = []
for i in range(10):
thread = threading.Thread(target=worker, args=(lock,))
threads.append(thread)
for thread in threads:
thread.start()
for thread in threads:
thread.join()
```
**逻辑分析:**
该代码创建了10个线程,每个线程都尝试访问共享资源。`lock`用于确保一次只有一个线程可以访问共享资源,防止数据竞争。`with`语句确保在访问共享资源时自动获取和释放锁。
**参数说明:**
- `lock`:用于同步线程访问共享资源的锁对象。
- `worker`:要执行的线程函数。
- `threads`:存储所有创建的线程的列表。
# 3.1 创建和管理线程
### 线程创建
**Java 中创建线程的方法:**
- **继承 Thread 类:**创建子类并重写 `run()` 方法。
- **实现 Runnable 接口:**创建实现 `Runnable` 接口的类,并在 `run()` 方法中定义线程逻辑。
**代码块:**
```java
// 继承 Thread 类创建线程
public class MyThread extends Thread {
@Override
public void run() {
// 线程逻辑
}
}
// 实现 Runnable 接口创建线程
public class MyRunnable implements Runnable {
@Override
public void run() {
// 线程逻辑
}
}
```
### 线程启动
**启动线程:**
- 调用 `start()` 方法。
**代码块:**
```java
// 启动继承 Thread 类创建的线程
MyThread thread = new MyThread();
thread.start();
// 启动实现 Runnable 接口创建的线程
Runnable runnable = new MyRunnable();
Thread thread = new Thread(runnable);
thread.start();
```
### 线程状态管理
**线程状态:**
- NEW:线程刚创建。
- RUNNABLE:线程可运行。
- WAITING:线程等待资源。
- TIMED_WAITING:线程在指定时间内等待资源。
- TERMINATED:线程已终止。
**获取线程状态:**
- `getState()` 方法。
**代码块:**
```java
Thread thread = new Thread();
System.out.println(thread.getState()); // 输出 NEW
```
### 线程优先级
**线程优先级:**
- 范围:1-10,10 最高。
- 默认优先级:5。
**设置线程优先级:**
- `setPriority()` 方法。
**代码块:**
```java
Thread thread = new Thread();
thread.setPriority(Thread.MAX_PRIORITY); // 设置最高优先级
```
### 线程组
**线程组:**
- 管理线程集合。
- 提供对线程组中线程的控制和监控。
**创建线程组:**
- `ThreadGroup` 类。
**代码块:**
```java
ThreadGroup group = new ThreadGroup("MyThreadGroup");
```
### 线程中断
**线程中断:**
- 向线程发送中断信号,请求线程停止。
- 通过 `interrupt()` 方法发送中断信号。
**代码块:**
```java
Thread thread = new Thread();
thread.interrupt();
```
# 4.1 线程安全与并发编程
### 4.1.1 线程安全的概念
线程安全是指一个对象或代码块可以在多个线程同时访问和修改的情况下,仍然保持其正确性和一致性。在多线程环境中,如果不考虑线程安全问题,可能会导致数据竞争、死锁等问题。
### 4.1.2 线程安全实现方法
实现线程安全有以下几种常见方法:
- **互斥锁(Mutex)**:互斥锁是一种同步原语,它允许同一时刻只有一个线程访问共享资源。当一个线程获取互斥锁后,其他线程将被阻塞,直到该线程释放互斥锁。
- **读写锁(RWLock)**:读写锁允许多个线程同时读取共享资源,但只能有一个线程同时写入共享资源。这样可以提高并发读操作的性能。
- **原子操作**:原子操作是一组不可分割的操作,要么全部执行成功,要么全部执行失败。原子操作可以保证在多线程环境中操作的正确性。
- **无锁并发编程**:无锁并发编程是一种通过使用无锁数据结构和算法来实现线程安全的方法。无锁并发编程可以避免锁带来的性能开销,但实现难度较高。
### 4.1.3 线程安全注意事项
在进行并发编程时,需要注意以下几点:
- **识别共享资源**:确定哪些数据或对象需要保护,并采取相应的线程安全措施。
- **避免数据竞争**:数据竞争是指多个线程同时访问和修改同一份数据,导致数据不一致。可以通过使用同步原语或无锁并发编程来避免数据竞争。
- **考虑死锁**:死锁是指多个线程相互等待对方释放资源,导致所有线程都无法继续执行。在设计并发程序时,应避免死锁的发生。
### 代码示例
**互斥锁示例**
```java
public class Counter {
private int count = 0;
private final Object lock = new Object();
public void increment() {
synchronized (lock) {
count++;
}
}
public int getCount() {
synchronized (lock) {
return count;
}
}
}
```
**读写锁示例**
```java
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class ReadWriteCounter {
private int count = 0;
private final ReentrantReadWriteLock lock = new ReentrantReadWriteLock();
public void increment() {
lock.writeLock().lock();
try {
count++;
} finally {
lock.writeLock().unlock();
}
}
public int getCount() {
lock.readLock().lock();
try {
return count;
} finally {
lock.readLock().unlock();
}
}
}
```
**原子操作示例**
```java
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicCounter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
```
# 5. 多线程编程性能优化
### 5.1 线程调度与负载均衡
**线程调度**
线程调度是操作系统负责将线程分配给CPU执行的过程。常见的线程调度算法有:
- **先来先服务 (FCFS)**:按照线程到达就绪队列的顺序执行。
- **时间片轮转 (RR)**:每个线程分配一个时间片,在时间片内执行,时间片用完后,线程会被挂起,等待下一个时间片。
- **优先级调度**:根据线程的优先级执行,优先级高的线程优先执行。
- **公平调度**:保证每个线程获得公平的CPU时间。
**负载均衡**
负载均衡是指在多核CPU或多台服务器上合理分配线程,以充分利用计算资源。常用的负载均衡策略有:
- **轮询**:将线程依次分配给不同的CPU或服务器。
- **加权轮询**:根据CPU或服务器的负载情况,分配不同的权重,权重高的CPU或服务器获得更多的线程。
- **最少连接**:将线程分配给负载最小的CPU或服务器。
- **动态负载均衡**:根据系统负载情况动态调整负载均衡策略。
### 5.2 性能瓶颈分析与解决
**性能瓶颈**
性能瓶颈是指系统中限制性能的因素。常见的性能瓶颈有:
- **CPU瓶颈**:CPU处理能力不足,导致线程无法及时执行。
- **内存瓶颈**:内存不足或访问速度慢,导致线程频繁进行页面置换。
- **IO瓶颈**:IO设备处理能力不足或访问速度慢,导致线程等待IO操作完成。
- **锁争用**:多个线程同时争用同一把锁,导致线程阻塞。
**解决性能瓶颈**
解决性能瓶颈的方法有:
- **优化代码**:减少不必要的计算和IO操作,提高代码执行效率。
- **增加资源**:增加CPU、内存或IO设备,提高系统处理能力。
- **优化线程调度**:选择合适的线程调度算法,合理分配线程。
- **优化负载均衡**:选择合适的负载均衡策略,充分利用计算资源。
- **减少锁争用**:使用无锁并发编程技术,减少锁的使用。
### 5.3 线程池调优与最佳实践
**线程池调优**
线程池调优是指根据系统负载情况调整线程池的大小和配置。常见的线程池调优参数有:
- **核心线程数**:线程池中始终保持的最小线程数。
- **最大线程数**:线程池中允许的最大线程数。
- **队列大小**:线程池中等待执行的线程队列的大小。
**最佳实践**
使用线程池的最佳实践包括:
- **使用固定大小的线程池**:避免频繁创建和销毁线程,降低系统开销。
- **设置合理的线程池大小**:根据系统负载情况设置合适的线程池大小,避免资源浪费或线程饥饿。
- **使用队列**:使用队列缓冲等待执行的线程,避免线程频繁阻塞。
- **监控线程池状态**:监控线程池的负载情况,及时调整线程池大小或配置。
# 6.1 并发Web服务
在现代Web应用中,并发性至关重要。用户希望快速响应,即使服务器负载很高。多线程编程是实现并发Web服务的关键技术。
### 线程池管理
Web服务器通常使用线程池来处理请求。线程池是一组预先创建的线程,当有新请求到达时,服务器将从池中分配一个线程来处理它。这可以减少创建和销毁线程的开销,从而提高性能。
```java
ExecutorService executorService = Executors.newFixedThreadPool(10);
executorService.submit(() -> {
// 处理请求
});
```
### 异步处理
异步处理允许服务器在处理请求时释放线程。这对于长时间运行的请求非常有用,因为它可以防止线程被阻塞,从而提高整体吞吐量。
```java
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// 处理请求
});
future.thenAccept(result -> {
// 处理结果
});
```
### 负载均衡
当服务器负载过高时,负载均衡可以将请求分布到多个服务器上。这有助于防止任何一台服务器过载,从而提高整体可用性和性能。
```
// Nginx负载均衡配置
upstream my_servers {
server server1.example.com:80;
server server2.example.com:80;
}
```
### 缓存
缓存可以减少数据库查询和文件读取的开销。通过在内存中存储经常访问的数据,Web服务器可以显著提高响应时间。
```java
@Cacheable(value = "users", key = "#username")
public User getUserByUsername(String username) {
// 从数据库获取用户
}
```
### 优化技巧
* 使用轻量级线程。
* 避免线程阻塞。
* 优化线程池大小。
* 使用异步处理。
* 实现负载均衡。
* 利用缓存。
0
0
相关推荐
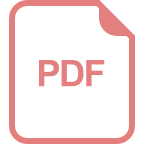
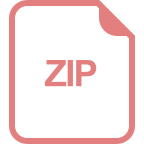
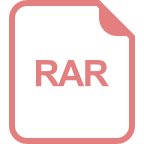





