在Python中使用Apache Thrift进行通信
发布时间: 2024-02-24 19:21:25 阅读量: 40 订阅数: 24 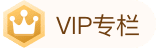
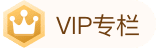
# 1. 理解Apache Thrift
Apache Thrift是一个跨语言的远程服务调用框架,可以帮助不同编程语言之间实现跨平台的通信。在本章中,我们将深入理解Apache Thrift的作用、优势以及应用场景。让我们一起来探索吧。
## 1.1 什么是Apache Thrift
Apache Thrift是一个用于构建高效且可扩展的服务开发框架,最初由Facebook开发。它允许开发者在不同编程语言之间进行远程调用,使得不同平台的应用程序可以轻松地进行通信和交互。
## 1.2 Apache Thrift的优势
- **跨语言支持**:Apache Thrift支持多种编程语言,包括Python、Java、Go等,使得不同语言之间的通信变得更加简单。
- **高效性**:Thrift使用二进制传输,比起文本传输具有更高的效率和性能。
- **可扩展性**:Thrift支持自定义数据类型和接口,并能够自动生成相应的代码,方便快捷地实现服务的扩展。
- **平台无关性**:Thrift生成的代码可以在不同平台上运行并相互通信,极大地提高了系统的灵活性和可移植性。
## 1.3 Apache Thrift的应用场景
Apache Thrift在各种场景下都有着广泛的应用,例如:
- **微服务架构**:Thrift可以帮助不同微服务之间进行通信,实现服务间的相互调用。
- **分布式系统**:在分布式系统中,不同节点之间需要进行数据交换和通信,Thrift可以简化这一过程。
- **跨平台应用**:对于需要跨平台通信的应用,如移动开发、Web开发等,Thrift可以提供便利的解决方案。
通过对Apache Thrift的理解,我们可以更好地利用这一工具来构建高效稳定的跨语言通信系统。
# 2. 在Python中安装Apache Thrift
Apache Thrift是一种跨语言的服务框架,它可以帮助不同编程语言的应用程序进行通信。在本章节中,我们将详细介绍如何在Python中安装Apache Thrift,并配置相应的环境以及安装Thrift Python库。
### 2.1 安装Apache Thrift
首先,我们需要在本地机器上安装Apache Thrift。我们可以从官方网站https://thrift.apache.org/ 下载最新版本的Apache Thrift,并根据相应的安装文档进行安装。
### 2.2 配置Python环境
在安装完成Apache Thrift之后,我们需要配置Python的环境以便与Apache Thrift进行通信。我们可以通过以下步骤进行配置:
1. 确保Python已经安装在本地机器上,并且配置了正确的环境变量。
2. 安装pip(Python的包管理工具),可以通过官方网站https://pip.pypa.io/en/stable/installation/ 获取安装指南。
3. 使用pip安装thrift包,可以通过命令行执行`pip install thrift`来安装Thrift Python库。
### 2.3 安装Thrift Python库
Thrift Python库是Apache Thrift的Python实现,它提供了与Thrift服务进行通信的相关功能。在安装完成Thrift Python库之后,我们可以在Python代码中引入Thrift库,从而实现与其他语言编写的服务进行通信的能力。
通过以上步骤,我们就可以在Python中使用Apache Thrift进行通信了。接下来,我们将在第三章中详细介绍如何定义Thrift接口。
# 3. 定义Thrift接口
Apache Thrift 是一种跨语言的服务框架,它使用简单的接口定义语言(IDL)来定义和创建跨语言的服务。在本章中,我们将学习如何使用 Apache Thrift 来定义接口。
#### 3.1 Thrift接口定义语言
Thrift 接口定义语言(IDL)是一种类似于结构体和接口的语言,用于定义数据类型和接口的方法。它支持基本数据类型,容器类型和结构体,并且还可以定义服务接口,包括方法的参数和返回类型。
#### 3.2 编写Thrift接口文件
首先,让我们创建一个简单的 Thrift 接口文件 `example.thrift`,用于定义一个简单的服务接口:
```thrift
namespace py example
struct User {
1: i32 id,
2: string name,
3: string email
}
service UserService {
User getUserById(1: i32 id),
void saveUser(1: User user)
}
```
在上面的例子中,我们定义了一个名为 `User` 的结构体,以及一个名为 `UserService` 的服务接口,包括了 `getUserById` 和 `saveUser` 两个方法。
#### 3.3 Thrift接口文件的结构和语法
- `namespace`:命名空间定义,用于避免命名冲突。
- `struct`:定义结构体,包括字段和字段的数据类型。
- `service`:定义服务接口,包括方法名称、参数和返回类型。
在接下来的章节中,我们将使用这个 Thrift 接口文件来生成 Python 代码,并实现服务端和客户端的通信。
在这一章节中,我们学习了如何使用 Apache Thrift 来定义接口。接下来,我们将继续学习如何生成 Python 代码并实现服务端和客户端的通信。
# 4. 生成Python代码
在本章中,我们将介绍如何使用Thrift编译器生成Python代码,以及生成的Python代码的介绍和使用方式。
#### 4.1 使用Thrift编译器生成Python代码
要生成Python代码,我们首先需要编写Thrift文件(.thrift),定义接口和数据结构。接着,我们可以使用Thrift编译器将Thrift文件编译成Python代码。假设我们已经编写好了一个名为example.thrift的Thrift文件,其中定义了接口和数据类型,现在我们需要使用Thrift编译器生成Python代码。
打开终端,使用以下命令来生成Python代码:
```shell
thrift --gen py example.thrift
```
执行该命令后,Thrift编译器会生成一个gen-py目录,里面包含了生成的Python代码。
#### 4.2 生成的Python代码介绍
生成的Python代码包含了接口定义和数据结构的实现,以及客户端和服务器端的代码。让我们简要介绍一下这些文件:
- **example.thrift**:原始的Thrift定义文件。
- **gen-py/example/constants.py**:包含了定义的常量。
- **gen-py/example/ttypes.py**:包含了定义的数据结构。
- **gen-py/example/ExampleService.py**:包含了定义的服务接口。
- **gen-py/ExampleService**:包含了服务器端和客户端的代码实现。
#### 4.3 Python代码的使用方式
生成的Python代码可以直接被导入和使用。在服务器端,我们可以实例化一个处理器对象,并将其绑定到服务器端口,启动Thrift服务。客户端需要导入生成的代码,并通过生成的客户端类来调用服务器端暴露的接口。
下面是一个简单的示例代码,用于演示如何使用生成的Python代码进行服务端和客户端的通信:
```python
# 服务器端代码
import sys
sys.path.append('gen-py')
from example import ExampleService
from example.ttypes import *
from thrift.transport import TSocket
from thrift.transport import TTransport
from thrift.protocol import TBinaryProtocol
from thrift.server import TServer
class ExampleHandler:
def ping(self):
return "pong"
handler = ExampleHandler()
processor = ExampleService.Processor(handler)
transport = TSocket.TServerSocket("localhost", 9090)
tfactory = TTransport.TBufferedTransportFactory()
pfactory = TBinaryProtocol.TBinaryProtocolFactory()
server = TServer.TSimpleServer(processor, transport, tfactory, pfactory)
print("Starting the server...")
server.serve()
print("Server stopped")
# 客户端代码
from example import ExampleService
from example.ttypes import *
from thrift import Thrift
from thrift.transport import TSocket
from thrift.transport import TTransport
from thrift.protocol import TBinaryProtocol
transport = TSocket.TSocket('localhost', 9090)
transport = TTransport.TBufferedTransport(transport)
protocol = TBinaryProtocol.TBinaryProtocol(transport)
client = ExampleService.Client(protocol)
transport.open()
response = client.ping()
print("Response from server: " + response)
transport.close()
```
在上面的示例中,我们首先启动了一个Thrift服务器,并在客户端调用了服务器暴露的ping接口。这演示了如何在Python中使用生成的Thrift代码进行通信。
### 总结
通过本章的学习,我们了解了如何使用Thrift编译器生成Python代码,以及生成的Python代码的介绍和使用方式。在下一章中,我们将进一步探讨如何使用Thrift进行通信。
# 5. 使用Thrift进行通信
Apache Thrift作为一种跨语言的远程过程调用(RPC)框架,在不同语言之间实现了更加高效的通信。在Python中使用Apache Thrift进行通信,可以通过定义Thrift接口文件、生成Python代码以及实现服务器端和客户端来实现。
### 5.1 服务器端实现
在服务器端,首先需要编写服务实现类来处理客户端的请求。以下是一个简单的Python示例:
```python
from example import ExampleService
from thrift.transport import TSocket
from thrift.transport import TTransport
from thrift.protocol import TBinaryProtocol
from thrift.server import TServer
class ExampleServiceHandler:
def __init__(self):
pass
def add(self, num1, num2):
return num1 + num2
handler = ExampleServiceHandler()
processor = ExampleService.Processor(handler)
transport = TSocket.TServerSocket(port=9090)
tfactory = TTransport.TBufferedTransportFactory()
pfactory = TBinaryProtocol.TBinaryProtocolFactory()
server = TServer.TSimpleServer(processor, transport, tfactory, pfactory)
print('Starting the server...')
server.serve()
```
在这段代码中,我们首先导入Thrift相关模块,并定义了一个示例的服务实现类`ExampleServiceHandler`,其中包含了一个简单的`add`方法用于实现加法操作。然后创建了服务处理器`processor`,指定了传输方式`transport`、传输格式`tfactory`和协议格式`pfactory`,最后创建了一个简单的Thrift服务器并启动。
### 5.2 客户端调用
客户端需要连接到服务器并调用远程服务方法。以下是一个简单的Python客户端示例:
```python
from example import ExampleService
from thrift.transport import TSocket
from thrift.transport import TTransport
from thrift.protocol import TBinaryProtocol
transport = TSocket.TSocket('localhost', 9090)
transport = TTransport.TBufferedTransport(transport)
protocol = TBinaryProtocol.TBinaryProtocol(transport)
client = ExampleService.Client(protocol)
transport.open()
result = client.add(10, 20)
print('10 + 20 =', result)
transport.close()
```
在这段代码中,我们首先导入Thrift相关模块,并创建了一个Thrift传输`transport`,指定了服务器地址和端口,然后创建了协议`protocol`。接着创建了一个远程服务客户端`client`,通过`transport.open()`打开传输连接,调用远程服务的`add`方法并打印结果,最后关闭传输连接。
### 5.3 Thrift通信的异常处理
在Thrift通信过程中,可能会出现各种异常情况,因此在代码中需要做必要的异常处理。例如,可以捕获`TTransportException`和`TException`等异常类型,并进行相应的处理,以确保通信的稳定性和可靠性。
通过以上示例,我们可以很好地理解如何在Python中使用Apache Thrift进行通信,包括在服务器端实现服务、客户端调用远程方法以及对通信过程中的异常进行处理。在实际应用中,可以根据具体需求和场景进一步扩展和优化代码。
# 6. 案例分析和实践
Apache Thrift的强大功能使其在实际应用中得到了广泛的应用。在本章中,我们将通过搭建简单的服务器和客户端,进行数据传输测试,并举例说明在实际场景中的应用。
#### 6.1 搭建简单的服务器和客户端
首先,我们需要编写一个简单的Thrift接口文件 `example.thrift`,定义接口和数据类型:
```thrift
namespace py example
struct UserData {
1: required i32 id,
2: required string username,
3: required string email
}
service UserService {
bool addUser(1: UserData userData),
UserData getUserById(1: i32 userId)
}
```
然后使用Thrift编译器生成Python代码:
```bash
thrift -r --gen py example.thrift
```
接着,我们编写服务器端代码 `server.py`:
```python
import sys
sys.path.append('./gen-py')
from example import UserService
from example.ttypes import UserData
from thrift.transport import TSocket
from thrift.transport import TTransport
from thrift.protocol import TBinaryProtocol
from thrift.server import TServer
class UserServiceHandler:
def addUser(self, userData):
print("Adding user:", userData.username)
# 实现添加用户的逻辑
return True
def getUserById(self, userId):
print("Getting user by id:", userId)
# 实现获取用户信息的逻辑
return UserData(id=userId, username="testuser", email="test@example.com")
if __name__ == '__main__':
handler = UserServiceHandler()
processor = UserService.Processor(handler)
transport = TSocket.TServerSocket(port=9090)
tfactory = TTransport.TBufferedTransportFactory()
pfactory = TBinaryProtocol.TBinaryProtocolFactory()
server = TServer.TSimpleServer(processor, transport, tfactory, pfactory)
print("Starting python server...")
server.serve()
```
接着,编写客户端代码 `client.py`:
```python
import sys
sys.path.append('./gen-py')
from example import UserService
from example.ttypes import UserData
from thrift import Thrift
from thrift.transport import TSocket
from thrift.transport import TTransport
from thrift.protocol import TBinaryProtocol
try:
transport = TSocket.TSocket('localhost', 9090)
transport = TTransport.TBufferedTransport(transport)
protocol = TBinaryProtocol.TBinaryProtocol(transport)
client = UserService.Client(protocol)
transport.open()
# 调用服务器端的添加用户接口
user = UserData(id=123, username="testuser", email="test@example.com")
result = client.addUser(user)
print("Add user result:", result)
# 调用服务器端的获取用户信息接口
user_id = 123
user_info = client.getUserById(user_id)
print("User Info:", user_info.username, user_info.email)
transport.close()
except Thrift.TException as tx:
print('%s' % tx.message)
```
#### 6.2 进行简单的数据传输测试
在命令行中分别运行服务器端代码和客户端代码:
```bash
python server.py
python client.py
```
可以看到客户端成功调用了服务器端的接口,并且获取到了正确的数据。
#### 6.3 实际场景中的应用举例
在实际场景中,我们可以使用Apache Thrift实现跨语言的服务通信,例如在一个分布式系统中,各个微服务可以使用不同的编程语言编写,通过Thrift定义接口和数据类型,实现不同服务之间的通信和数据交互。这种方式使得系统更加灵活和易于维护。
0
0
相关推荐








