【单片机串口驱动程序开发实战】:从入门到精通,掌握串口通信的奥秘
发布时间: 2024-07-10 09:44:52 阅读量: 72 订阅数: 55 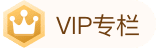
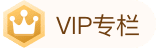
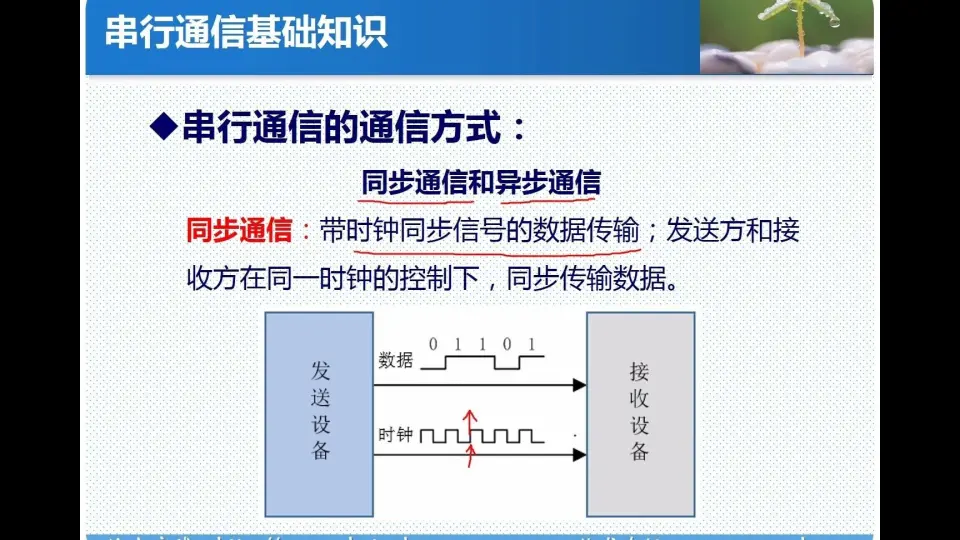
# 1. 单片机串口通信基础
串口通信是一种广泛应用于单片机和嵌入式系统中的数据传输方式。它通过一根或多根导线进行异步串行通信,具有成本低、易于实现、可靠性高的特点。
### 1.1 串口硬件结构
串口硬件通常包括发送器、接收器、移位寄存器和控制逻辑。发送器将并行数据转换为串行数据,然后通过导线发送出去。接收器将串行数据转换为并行数据,并将其提供给单片机。
### 1.2 串口通信协议
串口通信协议定义了数据传输的规则,包括数据位、停止位、奇偶校验位等参数。常见的串口通信协议有UART、RS-232、RS-485等。
# 2. 串口驱动程序理论
### 2.1 串口通信原理
**2.1.1 串口硬件结构**
串口硬件主要由以下部分组成:
- **串口控制器 (UART):**负责管理串口通信,包括数据传输、接收和错误处理。
- **发送缓冲区和接收缓冲区:**用于存储待发送和已接收的数据。
- **数据寄存器:**用于存储当前正在发送或接收的数据。
- **控制寄存器:**用于配置串口参数,如波特率、数据位、奇偶校验等。
- **中断线:**当数据到达或发送完成时,中断线会触发中断,通知处理器。
### 2.1.2 串口通信协议
串口通信协议定义了数据在串口线上传输的方式。常见的串口协议包括:
- **异步通信:**数据以单个字符为单位传输,每个字符包含一个起始位、数据位、奇偶校验位和停止位。
- **同步通信:**数据以帧为单位传输,每个帧包含一个同步字符、数据和一个帧结束字符。
**参数说明:**
- **波特率:**数据传输速率,单位为比特/秒 (bps)。
- **数据位:**每个字符包含的数据位数,通常为 5、6、7 或 8 位。
- **奇偶校验:**用于检测数据传输中的错误,奇偶校验位可以是奇校验或偶校验。
- **停止位:**字符传输结束时发送的位数,通常为 1 或 2 位。
### 2.2 串口驱动程序设计
**2.2.1 驱动程序架构**
串口驱动程序通常采用分层架构:
- **硬件抽象层 (HAL):**提供对串口硬件的低级访问,屏蔽硬件差异。
- **驱动程序核心:**实现串口通信的基本功能,如数据发送、接收和中断处理。
- **应用层接口 (API):**提供用户应用程序与驱动程序交互的接口。
**2.2.2 中断处理机制**
串口通信通常使用中断机制来处理数据传输和接收。当数据到达或发送完成时,UART 会触发中断,处理器会执行中断服务程序 (ISR)。ISR 的主要职责是:
- **接收中断:**读取接收缓冲区中的数据并将其存储在用户缓冲区中。
- **发送中断:**从用户缓冲区中读取数据并将其发送到串口线上。
**代码块:**
```c
void UART_ISR(void) {
// 判断中断类型
if (UART_GetInterruptStatus() & UART_RX_INT) {
// 接收中断
uint8_t data = UART_ReceiveData();
// 将数据存储在用户缓冲区中
UserBuffer_AddData(data);
} else if (UART_GetInterruptStatus() & UART_TX_INT) {
// 发送中断
uint8_t data = UserBuffer_GetData();
// 将数据发送到串口线上
UART_SendData(data);
}
}
```
**代码逻辑分析:**
1. 判断中断类型,如果是接收中断,则读取接收缓冲区中的数据并存储在用户缓冲区中。
2. 如果是发送中断,则从用户缓冲区中读取数据并发送到串口线上。
# 3.1 驱动程序开发环境搭建
#### 3.1.1 开发工具选择
驱动程序开发需要使用专门的开发工具,常见的选择包括:
- **Keil MDK**:一款流行的集成开发环境(IDE),支持多种微控制器和开发板。
- **IAR Embedded Workbench**:另一个流行的IDE,以其强大的调试功能而闻名。
- **GCC Toolchain**:一个开源的编译器套件,支持多种操作系统和架构。
选择开发工具时,需要考虑以下因素:
- **目标微控制器支持:**确保开发工具支持目标微控制器。
- **功能和特性:**评估开发工具提供的功能和特性,例如调试器、代码编辑器和编译器。
- **价格和许可:**考虑开发工具的成本和许可条款。
#### 3.1.2 开发环境配置
开发环境配置包括以下步骤:
1. **安装开发工具:**从官方网站下载并安装选定的开发工具。
2. **创建项目:**在开发工具中创建一个新的项目,指定目标微控制器和开发板。
3. **添加源代码:**将驱动程序源代码文件添加到项目中。
4. **配置编译器和链接器:**设置编译器和链接器选项,例如优化级别和链接器脚本。
5. **调试配置:**配置调试器,以便在调试过程中单步执行代码和检查变量。
配置开发环境时,需要仔细检查设置,以确保驱动程序能够正确编译、链接和调试。
# 4. 串口驱动程序高级应用**
**4.1 串口数据缓冲区管理**
**4.1.1 缓冲区设计**
串口数据缓冲区是用来存储待发送或已接收的数据。其设计需要考虑以下因素:
- **缓冲区大小:**应根据应用程序的需求和串口通信速率确定。过小的缓冲区可能导致数据丢失,过大的缓冲区会浪费内存。
- **缓冲区类型:**可以选择循环缓冲区或线性缓冲区。循环缓冲区可以实现数据循环覆盖,而线性缓冲区需要手动管理缓冲区指针。
- **缓冲区访问机制:**需要定义用于访问缓冲区的同步机制,如互斥锁或信号量。
**4.1.2 缓冲区管理算法**
缓冲区管理算法负责控制数据的写入和读取操作。常见的算法包括:
- **先入先出 (FIFO):**数据按写入顺序读取。
- **后入先出 (LIFO):**数据按写入顺序的逆序读取。
- **优先级队列:**根据数据优先级进行读取。
**4.2 串口通信协议实现**
**4.2.1 通信协议设计**
串口通信协议定义了数据传输的格式和规则。其设计需要考虑以下方面:
- **帧格式:**定义数据帧的结构,包括帧头、帧尾、数据区等。
- **数据编码:**指定数据如何编码,如 ASCII、二进制等。
- **错误检测和纠正:**使用校验和或 CRC 等机制检测和纠正传输错误。
**4.2.2 协议解析和处理**
协议解析和处理模块负责解析接收到的数据帧,提取有效数据并执行相应的操作。其主要步骤包括:
- **帧同步:**识别帧头和帧尾,确定帧边界。
- **数据提取:**从数据区提取有效数据。
- **错误检测:**验证校验和或 CRC,确保数据完整性。
- **数据处理:**根据协议定义,执行相应的操作,如数据存储、命令执行等。
# 5. 串口驱动程序调试和优化
### 5.1 驱动程序调试方法
驱动程序调试是发现和修复驱动程序中错误和问题的过程。常见的调试方法包括:
- **日志打印:**在驱动程序代码中添加日志语句,以记录重要的事件和数据。这有助于识别问题发生的时机和原因。
- **断点调试:**使用调试器在特定代码行设置断点,以暂停程序执行并检查变量值和内存状态。
### 5.2 驱动程序性能优化
优化驱动程序性能可以提高系统效率和响应能力。以下是一些优化方法:
- **代码优化:**使用编译器优化选项、避免不必要的函数调用和循环、减少代码复杂度。
- **硬件优化:**选择合适的硬件设备、优化数据传输路径、使用DMA(直接内存访问)技术减少CPU开销。
**代码优化示例:**
```c
// 未优化代码
for (i = 0; i < 100; i++) {
if (data[i] == 0) {
// 处理数据
}
}
// 优化后的代码
int count = 0;
for (i = 0; i < 100; i++) {
if (data[i] == 0) {
count++;
}
}
if (count > 0) {
// 处理数据
}
```
**硬件优化示例:**
- 使用 DMA 传输数据,减少 CPU 开销。
- 选择具有更高传输速率的串口设备。
- 优化数据传输路径,减少延迟。
0
0
相关推荐
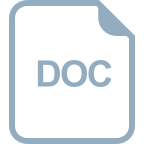
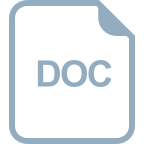
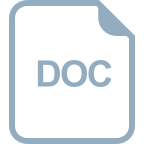
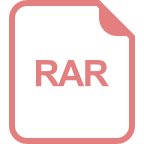
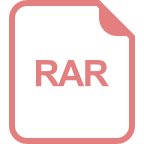
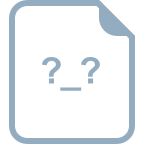
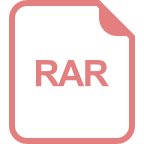
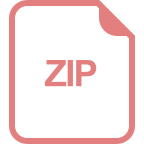