使用Barrier在ConstraintLayout中创建灵活的约束
发布时间: 2023-12-19 14:49:28 阅读量: 151 订阅数: 24 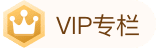
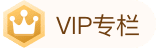
# 1. 介绍Barrier和ConstraintLayout的概念
## 1.1 Barrier的作用与用途
Barrier是Android ConstraintLayout布局中的一个特殊元素,它用于创建约束布局中的障碍物。在约束布局中,通常需要处理多个视图之间的依赖关系,例如一个视图需要依赖于其他视图的位置或大小。Barrier的作用就是在布局中创建一个可变的障碍物,用于控制视图的位置关系。
使用Barrier可以简化和优化布局代码,提高布局的灵活性和可拓展性。例如,在一个水平布局中,我们可以使用Barrier来创建左右两边的约束,而不需要为每个视图单独指定约束。
## 1.2 ConstraintLayout的基本概述
ConstraintLayout是Android布局中的一个强大的约束布局容器,它可以灵活地定义视图之间的相对关系,并根据这些关系自动调整视图的位置和大小。ConstraintLayout通过约束属性来定义视图的位置和大小,例如顶部对齐、左侧对齐、居中等。
与其他布局容器相比,ConstraintLayout更加灵活和高效。它可以减少视图层次的嵌套, 提高布局绘制的性能。ConstraintLayout还提供了丰富的布局属性和动画效果,使开发者更容易实现复杂的UI布局。
接下来,我们将展开讨论如何使用Barrier在ConstraintLayout中创建灵活的约束布局。
# 2. 使用Barrier创建水平约束
Barrier是ConstraintLayout中一个非常有用的特性,它可以帮助我们创建复杂的约束布局。在这一章节中,将介绍如何使用Barrier来创建水平约束。
### 2.1 使用Barrier创建左右两边的约束
首先,让我们来看一个简单的例子。假设我们有一个包含两个按钮的布局,想要让这两个按钮分别与父布局的左右两边对齐。
```java
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/leftButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Left Button"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/rightButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Right Button"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
为了使这两个按钮能够与父布局的左右两边对齐,我们可以使用Barrier来创建水平约束。下面是如何实现的:
```java
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/leftButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Left Button"
android:layout_marginEnd="8dp"
app:layout_constraintEnd_toStartOf="@+id/barrier"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/rightButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Right Button"
android:layout_marginStart="8dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toEndOf="@+id/barrier"
app:layout_constraintTop_toTopOf="parent" />
<androidx.constraintlayout.widget.Barrier
android:id="@+id/barrier"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:barrierDirection="left"
app:constraint_referenced_ids="leftButton,rightButton" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
在上面的代码中,我们在左边的按钮和右边的按钮之间创建了一个Barrier。`app:barrierDirection="left"`表示Barrier在左边按钮和右边按钮之间。`app:constraint_referenced_ids="leftButton,rightButton"`表示Barrier所依赖的控件的id。
### 2.2 使用Barrier创建屏幕中间的约束
除了创建左右两边的约束,Barrier还可以帮助我们创建水平居中的约束。下面是一个示例:
```java
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/startButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Start Button"
app:layout_constraintEnd_toStartOf="@+id/barrier"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/endButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="End Button"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toEndOf="@+id/barrier"
app:layout_constraintTop_toTopOf="parent" />
<androidx.constraintlayout.widget.Barrier
android:id="@+id/barrier"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:barrierDirection="start"
app:constraint_referenced_ids="startButton,endButton" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
在上面的代码中,我们将Barrier设置为居中对齐,即`app:barrierDirection="start"`。通过这样的设置,Bar分隔符将会位于屏幕的中间,从而实现了水平居中的约束布局。
### 2.3 使用Barrier创建可变数量的水平约束
Barrier还支持创建可变数量的水平约束,意味着你可以根据需要动态地添加或删除约束。下面是一个示例:
```java
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 1"
app:layout_constraintEnd_toStartOf="@+id/barrier"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 2"
app:layout_constraintEnd_toStartOf="@+id/barrier"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button1" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 3"
app:layout_constraintEnd_toStartOf="@+id/barrier"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button2" />
<androidx.constraintlayout.widget.Barrier
android:id="@+id/barrier"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:barrierDirection="end"
app:constraint_referenced_ids="button1,button2,button3" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
在上面的代码中,我们使用了三个按钮来演示可变数量的水平约束。Barrier的`app:constraint_referenced_ids`属性包含了所有参与水平约束的按钮的id。这样,无论您添加或删除多少个按钮,Barrier都可以自动调整约束布局。
通过本章节的讲解,你已经学会了使用Barrier创建水平约束布局。无论是左右两边对齐、屏幕中间对齐,还是可变数量的约束,Barrier都能帮助我们轻松实现。在接下来的章节中,我们将继续探讨如何使用Barrier创建垂直约束布局。
# 3. 使用Barrier创建垂直约束
在前面的章节中,我们已经了解了如何使用Barrier创建水平约束。接下来,我们将介绍如何使用Barrier创建垂直约束。
#### 3.1 使用Barrier创建上下两边的约束
首先,让我们来看一个简单的例子,使用Barrier创建上下两边的约束:
```java
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<
```
0
0