Python动物代码实战:构建一个虚拟动物园,体验动物世界的生机勃勃
发布时间: 2024-06-20 13:45:27 阅读量: 140 订阅数: 26 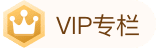
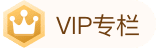
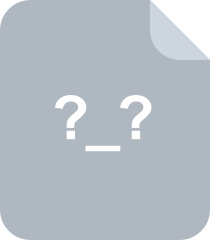
python虚拟环境创建

# 1. Python动物代码实战概述
Python动物代码实战是一种利用Python编程语言模拟虚拟动物园的编程练习。它涉及数据结构、算法、面向对象编程等基础知识,以及命令行交互、图形化展示等高级应用。通过构建一个虚拟动物园,学习者可以深入理解Python编程原理,并提升解决实际问题的编程能力。
本实战项目将从动物类和动物园类设计开始,逐步实现动物行为模拟、动物园管理、命令行交互和图形化展示等功能。在过程中,将重点讲解Python编程中的关键概念,如数据结构、算法、面向对象编程、命令行交互和图形化展示。
# 2. Python动物代码基础
### 2.1 Python数据结构和算法
#### 2.1.1 列表、字典和集合
Python提供了强大的数据结构,包括列表、字典和集合。
* **列表**是有序的可变序列,可以存储任何类型的数据。
```python
animals = ['lion', 'tiger', 'bear', 'wolf']
```
* **字典**是无序的键值对集合,其中键是唯一的,而值可以是任何类型的数据。
```python
animal_attributes = {
'lion': {'size': 'large', 'color': 'yellow'},
'tiger': {'size': 'large', 'color': 'orange'},
'bear': {'size': 'medium', 'color': 'brown'},
'wolf': {'size': 'small', 'color': 'gray'}
}
```
* **集合**是无序的唯一元素集合,可以用于快速查找和删除元素。
```python
unique_animals = set(['lion', 'tiger', 'bear', 'wolf'])
```
#### 2.1.2 排序、搜索和遍历
Python提供了用于对数据结构进行排序、搜索和遍历的算法。
* **排序**将元素按特定顺序排列。
```python
animals.sort() # 按字母顺序排序
```
* **搜索**查找特定元素的位置。
```python
if 'lion' in animals:
print('The lion is in the list.')
```
* **遍历**逐个访问数据结构中的元素。
```python
for animal in animals:
print(animal)
```
### 2.2 Python面向对象编程
#### 2.2.1 类和对象
面向对象编程(OOP)是一种编程范式,它将数据和方法组织成称为类的对象。
* **类**是对象的蓝图,它定义了对象的属性和方法。
```python
class Animal:
def __init__(self, name, species, age):
self.name = name
self.species = species
self.age = age
```
* **对象**是类的实例,它包含特定于该实例的数据和方法。
```python
lion = Animal('Leo', 'lion', 5)
```
#### 2.2.2 继承和多态
* **继承**允许一个类从另一个类继承属性和方法。
```python
class Lion(Animal):
def roar(self):
print('Roar!')
```
* **多态**允许具有不同类的对象以一致的方式响应相同的操作。
```python
def make_sound(animal):
animal.make_sound() # 调用不同的 make_sound() 方法,具体取决于 animal 的类型
```
# 3. 虚拟动物园设计与实现
### 3.1 动物类设计
#### 3.1.1 动物属性和方法
动物类是虚拟动物园中动物的抽象,它定义了动物的属性和行为。动物属性包括名称、种类、年龄、性别等基本信息,以及生命值、饱食度等动态属性。动物方法包括移动、进食、繁殖等行为。
```python
class Animal:
def __init__(self, name, species, age, gender):
self.name = name
self.species = species
self.age = age
self.gender = gender
self.health = 100
self.hunger = 100
def move(self):
print(f"{self.name} is moving.")
def eat(self):
print(f"{self.name} is eating.")
def breed(self, other_animal):
print(f"{self.name} is breeding with {other_animal.name}.")
```
#### 3.1.2 动物行为模拟
动物行为模拟是通过算法实现动物在虚拟动物园中的动态行为。例如,动物会随着时间推移而移动、进食和繁殖。动物移动算法可以采用随机游走或寻路算法,进食算法可以根据动物的饥饿程度和周围环境进行判断,繁殖算法可以根据动物的年龄和性别进行概率计算。
```python
import random
def move_randomly(animal):
x = random.randint(-1, 1)
y = random.randint(-1, 1)
animal.x += x
animal.y += y
def eat_if_hungry(animal):
if animal.hunger < 50:
animal.eat()
def breed_if_ready(animal):
if animal.age > 5 and animal.gender != animal.gender:
animal.breed(other_animal)
```
### 3.2 动物园类设计
#### 3.2.1 动物园结构
动物园类是虚拟动物园的整体结构,它包含所有动物和动物园环境信息。动物园环境包括围栏、水源和食物来源等。动物园结构可以采用网格状或树状结构,方便动物移动和管理。
```python
class Zoo:
def __init__(self, width, height):
self.width = width
self.height = height
self.animals = []
self.fences = []
self.water_sources = []
self.food_sources = []
def add_animal(self, animal):
self.animals.append(animal)
def add_fence(self, fence):
self.fences.append(fence)
def add_water_source(self, water_source):
self.water_sources.append(water_source)
def add_food_source(self, food_source):
self.food_sources.append(food_source)
```
#### 3.2.2 动物园管理
动物园管理包括动物的添加、删除、移动和繁殖等操作。动物园管理算法可以采用事件驱动或状态机模式,方便动物园的动态管理。
```python
def add_animal_to_zoo(zoo, animal):
zoo.add_animal(animal)
def remove_animal_from_zoo(zoo, animal):
zoo.animals.remove(animal)
def move_animal_in_zoo(zoo, animal, x, y):
animal.x = x
animal.y = y
def breed_animals_in_zoo(zoo):
for animal in zoo.animals:
breed_if_ready(animal)
```
# 4. 动物园交互与展示
### 4.1 命令行交互
#### 4.1.1 命令解析
动物园的命令行交互功能允许用户通过输入命令与动物园进行交互。这些命令可以用于管理动物、查看动物信息、模拟动物行为等。
命令解析器负责将用户输入的命令解析成可执行的指令。它通常遵循以下步骤:
1. **命令识别:**将输入的字符串与已知的命令列表进行匹配。
2. **参数提取:**从命令中提取参数,这些参数可以是动物名称、行为类型等。
3. **命令执行:**根据命令和参数,调用相应的函数执行命令。
#### 4.1.2 输出格式化
命令执行后,需要将结果以可读的方式呈现给用户。输出格式化器负责将数据结构转换为文本或其他格式。
常见的数据结构包括列表、字典和对象。输出格式化器可以将这些数据结构转换为以下格式:
- **文本格式:**以文本形式打印数据,例如:
```
动物列表:
- 狮子
- 老虎
- 猴子
```
- **表格格式:**将数据组织成表格,例如:
```
| 动物 | 年龄 | 性别 |
|---|---|---|
| 狮子 | 5 | 雄性 |
| 老虎 | 3 | 雌性 |
| 猴子 | 2 | 雄性 |
```
- **JSON格式:**以JSON格式输出数据,例如:
```json
{
"animals": [
{
"name": "狮子",
"age": 5,
"gender": "雄性"
},
{
"name": "老虎",
"age": 3,
"gender": "雌性"
},
{
"name": "猴子",
"age": 2,
"gender": "雄性"
}
]
}
```
### 4.2 图形化展示
#### 4.2.1 PyGame库介绍
PyGame是一个用于创建2D游戏和交互式应用程序的跨平台库。它提供了以下功能:
- **图形渲染:**创建窗口、绘制图形、处理事件。
- **声音播放:**播放音乐和音效。
- **输入处理:**处理键盘、鼠标和游戏手柄输入。
- **物理引擎:**模拟物理交互,例如重力、碰撞。
#### 4.2.2 动物园图形化界面
PyGame可用于创建动物园的图形化界面。该界面可以显示动物、动物园环境,并允许用户与动物交互。
以下代码示例展示了如何使用PyGame创建动物园的图形化界面:
```python
import pygame
# 初始化PyGame
pygame.init()
# 设置屏幕大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 创建动物园对象
zoo = Zoo()
# 游戏主循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 更新动物园状态
zoo.update()
# 渲染画面
screen.fill((0, 0, 0))
zoo.draw(screen)
# 更新显示
pygame.display.update()
# 退出PyGame
pygame.quit()
```
在这个示例中,`Zoo`类表示动物园,它包含动物列表、动物园环境和交互逻辑。`update()`方法更新动物园状态,`draw()`方法将动物园渲染到屏幕上。
# 5. 动物园扩展与优化
### 5.1 动物园扩展
#### 5.1.1 新动物添加
动物园的扩展包括添加新的动物种类。要添加新动物,需要遵循以下步骤:
1. **定义动物类:**创建新的动物类,继承自基类`Animal`,并实现其抽象方法。
2. **实现动物属性和方法:**定义新动物的属性和方法,包括其独特特征和行为。
3. **注册动物类:**将新动物类注册到动物园的动物类型字典中。
**代码示例:**
```python
# 定义新动物类
class Giraffe(Animal):
def __init__(self, name, age, gender):
super().__init__(name, age, gender)
self.height = 5.5 # 长颈鹿的独特属性
def eat(self):
print(f"{self.name} the giraffe is eating leaves.")
def sleep(self):
print(f"{self.name} the giraffe is sleeping standing up.")
# 注册新动物类
Animal.register_animal_type("Giraffe", Giraffe)
```
#### 5.1.2 动物园环境扩展
除了添加新动物外,还可以扩展动物园的环境,例如添加新的栖息地或设施。
**代码示例:**
```python
# 添加新栖息地
zoo.add_habitat("African Savanna")
# 添加新设施
zoo.add_facility("Water Hole")
```
### 5.2 动物园优化
#### 5.2.1 性能优化
性能优化对于大型动物园至关重要。可以采用以下方法优化性能:
* **使用高效的数据结构:**使用列表或字典等高效的数据结构存储动物和栖息地信息。
* **优化算法:**使用快速排序或二分查找等优化算法进行搜索和排序操作。
* **并行化任务:**使用多线程或多进程并行化动物行为模拟或图形化展示等任务。
**代码示例:**
```python
# 使用并行化任务
import threading
def simulate_animal_behavior(animal):
# 模拟动物行为
threads = []
for animal in zoo.animals:
thread = threading.Thread(target=simulate_animal_behavior, args=(animal,))
threads.append(thread)
for thread in threads:
thread.start()
```
#### 5.2.2 代码重构
代码重构可以提高代码的可读性、可维护性和可扩展性。可以采用以下方法重构代码:
* **提取方法:**将重复的代码块提取到单独的方法中。
* **使用设计模式:**使用设计模式(如工厂模式或观察者模式)来提高代码的灵活性。
* **重构类结构:**重构类结构以提高代码的模块化和可重用性。
**代码示例:**
```python
# 提取方法
def create_animal(animal_type, name, age, gender):
animal_class = Animal.get_animal_type(animal_type)
return animal_class(name, age, gender)
# 使用设计模式
class AnimalObserver:
def __init__(self, animal):
self.animal = animal
def update(self):
print(f"{self.animal.name} has changed its state.")
# 重构类结构
class ZooManager:
def __init__(self):
self.animals = []
self.habitats = []
def add_animal(self, animal):
self.animals.append(animal)
def add_habitat(self, habitat):
self.habitats.append(habitat)
```
# 6.1 实战总结
通过Python动物代码实战,我们深入探索了Python编程的各个方面,从数据结构和算法到面向对象编程,再到虚拟动物园的设计和实现。
**数据结构和算法**:我们使用了列表、字典和集合等数据结构来存储动物信息,并应用了排序、搜索和遍历等算法来高效处理数据。
**面向对象编程**:我们设计了动物类和动物园类,利用继承和多态等特性实现了动物行为和动物园管理的模拟。
**虚拟动物园设计和实现**:我们创建了一个虚拟动物园,其中包含各种动物,并提供了命令行交互和图形化展示等功能。
**动物园交互与展示**:我们实现了命令行交互,允许用户输入命令来管理动物园,并使用PyGame库创建了图形化界面,直观地展示动物园中的动物。
**动物园扩展与优化**:我们扩展了动物园,添加了新动物和环境,并优化了代码性能和结构,提高了动物园的运行效率和可维护性。
## 6.2 动物代码的应用与展望
Python动物代码实战不仅是一个有趣的编程项目,它还为我们提供了宝贵的经验和洞察力,可应用于实际的软件开发中。
**应用**:
* **虚拟宠物游戏**:可以扩展动物代码来创建虚拟宠物游戏,允许玩家与动物互动并照顾它们。
* **动物行为模拟**:可以利用动物代码来模拟动物行为,用于研究和教育目的。
* **数据分析**:可以将动物代码与数据分析库结合使用,分析动物数据并提取有价值的见解。
**展望**:
* **人工智能集成**:可以将人工智能技术集成到动物代码中,使动物能够自主学习和适应环境。
* **云计算支持**:可以将动物代码部署到云平台上,实现可扩展性和高可用性。
* **移动应用开发**:可以将动物代码移植到移动设备上,创建移动版的虚拟动物园或宠物游戏。
0
0
相关推荐
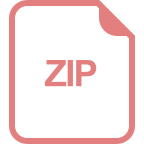
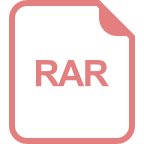
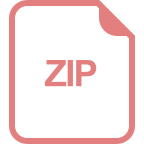
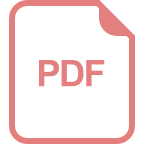
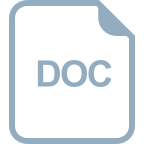