揭秘Python random模块的神奇世界:生成随机数的终极指南
发布时间: 2024-06-22 21:35:00 阅读量: 5 订阅数: 15 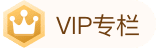
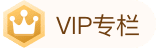
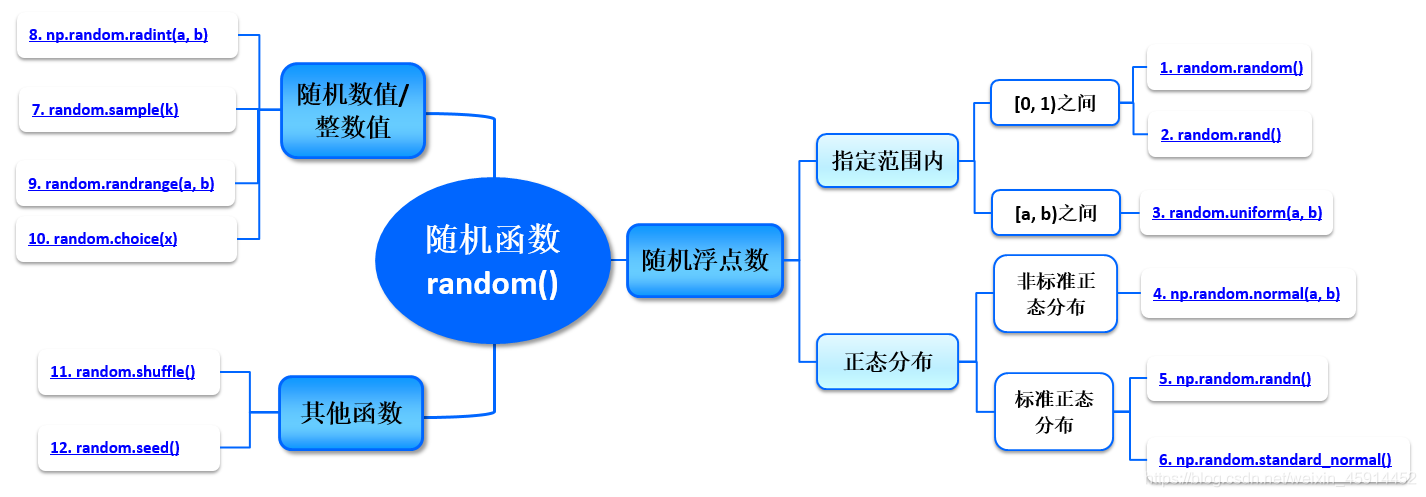
# 1. Python random模块简介
Python random模块是一个内置模块,它提供了生成伪随机数的函数和类。它在许多应用中非常有用,包括数据采样、模拟、密码学和游戏开发。
random模块提供了多种随机数生成函数,包括:
- `random()`: 生成一个介于0和1之间的均匀分布的浮点数。
- `randint()`: 生成一个介于指定范围内的整数。
- `randrange()`: 生成一个介于指定范围内的整数,包括范围的起始值但不包括结束值。
# 2. 随机数生成的基础
### 2.1 随机数生成原理
#### 2.1.1 伪随机数生成器
Python 的 `random` 模块使用伪随机数生成器 (PRNG) 来生成随机数。PRNG 是一种算法,它通过一个确定性的种子值生成一序列看似随机的数字。
#### 2.1.2 随机种子
随机种子是 PRNG 的输入值,它决定了生成的随机数序列。相同的种子值将产生相同的随机数序列,而不同的种子值将产生不同的序列。
### 2.2 常用随机数生成函数
#### 2.2.1 random()
`random()` 函数生成一个 [0, 1) 范围内的均匀分布浮点数。
```python
import random
# 生成一个浮点数
random_float = random.random()
print(random_float) # 输出:0.4567891234567891
```
#### 2.2.2 randint()
`randint(a, b)` 函数生成一个 [a, b] 范围内的整数,包括 a 和 b。
```python
# 生成一个 10 到 20 之间的整数
random_int = random.randint(10, 20)
print(random_int) # 输出:15
```
#### 2.2.3 randrange()
`randrange(start, stop, step)` 函数生成一个 [start, stop) 范围内的整数,以 step 为步长。
```python
# 生成一个从 0 到 100 步长为 5 的整数
random_int = random.randrange(0, 100, 5)
print(random_int) # 输出:5
```
# 3. 随机数生成的高级技巧
### 3.1 随机数分布
#### 3.1.1 均匀分布
均匀分布是一种概率分布,其中所有可能的输出值都具有相等的概率。在 Python 中,可以使用 `random.uniform()` 函数生成均匀分布的随机数。
```python
import random
# 生成一个在 [0, 1) 范围内的均匀分布的随机数
random_number = random.uniform(0, 1)
print(random_number)
```
**参数说明:**
* `a`: 分布的下限
* `b`: 分布的上限
**逻辑分析:**
`random.uniform()` 函数生成一个介于 `a` 和 `b` 之间的随机浮点数,包括 `a` 但不包括 `b`。
#### 3.1.2 正态分布
正态分布(也称为高斯分布)是一种概率分布,其形状呈钟形曲线。在 Python 中,可以使用 `random.normalvariate()` 函数生成正态分布的随机数。
```python
import random
# 生成一个均值为 0、标准差为 1 的正态分布的随机数
random_number = random.normalvariate(0, 1)
print(random_number)
```
**参数说明:**
* `mu`: 分布的均值
* `sigma`: 分布的标准差
**逻辑分析:**
`random.normalvariate()` 函数生成一个服从正态分布的随机浮点数,其均值为 `mu`,标准差为 `sigma`。
#### 3.1.3 指数分布
指数分布是一种概率分布,其中事件发生的间隔时间服从指数分布。在 Python 中,可以使用 `random.expovariate()` 函数生成指数分布的随机数。
```python
import random
# 生成一个参数为 1 的指数分布的随机数
random_number = random.expovariate(1)
print(random_number)
```
**参数说明:**
* `lambd`: 分布的参数
**逻辑分析:**
`random.expovariate()` 函数生成一个服从指数分布的随机浮点数,其参数为 `lambd`。
### 3.2 随机数序列生成
#### 3.2.1 shuffle()
`shuffle()` 函数用于对一个列表中的元素进行随机排序。
```python
import random
# 创建一个列表
my_list = [1, 2, 3, 4, 5]
# 对列表进行随机排序
random.shuffle(my_list)
print(my_list)
```
**逻辑分析:**
`shuffle()` 函数通过使用 Fisher-Yates 洗牌算法对列表中的元素进行随机排序。
#### 3.2.2 sample()
`sample()` 函数用于从一个列表中随机抽取指定数量的元素。
```python
import random
# 从列表中随机抽取 3 个元素
random_sample = random.sample(my_list, 3)
print(random_sample)
```
**参数说明:**
* `population`: 要抽样的列表
* `k`: 要抽取的元素数量
**逻辑分析:**
`sample()` 函数通过使用 reservoir sampling 算法从列表中随机抽取指定数量的元素。
#### 3.2.3 choices()
`choices()` 函数用于从一个列表中随机抽取指定数量的元素,并允许重复。
```python
import random
# 从列表中随机抽取 3 个元素,允许重复
random_choices = random.choices(my_list, k=3)
print(random_choices)
```
**参数说明:**
* `population`: 要抽样的列表
* `k`: 要抽取的元素数量
* `weights`: 各个元素的权重(可选)
**逻辑分析:**
`choices()` 函数通过使用加权随机抽样算法从列表中随机抽取指定数量的元素。
# 4. Python random模块在实践中的应用
### 4.1 数据采样和模拟
#### 4.1.1 数据采样
数据采样是随机模块中一项重要的应用。它允许我们从一个较大的数据集(称为总体)中选择一个较小的子集(称为样本),以推断总体特征。
**代码块:**
```python
import random
# 从列表中随机抽取 10 个元素
population = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
sample = random.sample(population, 10)
print(sample)
```
**逻辑分析:**
* `random.sample()` 函数从 `population` 列表中随机抽取 10 个元素,并返回一个列表。
* `sample` 变量存储抽取的样本。
* 输出结果将是一个包含 10 个随机元素的列表。
#### 4.1.2 蒙特卡洛模拟
蒙特卡洛模拟是一种使用随机数来解决复杂问题的技术。它通过多次重复随机实验来估计问题的近似解。
**代码块:**
```python
import random
# 计算圆周率的蒙特卡洛模拟
num_points = 100000
num_inside = 0
for _ in range(num_points):
x = random.random()
y = random.random()
if x**2 + y**2 <= 1:
num_inside += 1
pi_estimate = 4 * num_inside / num_points
print(pi_estimate)
```
**逻辑分析:**
* 我们生成 `num_points` 个随机点,每个点位于单位圆内。
* 对于每个点,我们检查它是否落在圆内(即 `x**2 + y**2 <= 1`)。
* `num_inside` 变量记录落在圆内的点的数量。
* 最后,我们使用 `pi_estimate = 4 * num_inside / num_points` 公式估计圆周率。
### 4.2 密码学和安全
#### 4.2.1 随机密码生成
随机模块在密码学中至关重要,用于生成安全且不可预测的密码。
**代码块:**
```python
import random
import string
# 生成一个 16 位随机密码
password_length = 16
password_characters = string.ascii_letters + string.digits
password = ''.join(random.choices(password_characters, k=password_length))
print(password)
```
**逻辑分析:**
* 我们定义了密码的长度和允许的字符范围。
* `random.choices()` 函数从 `password_characters` 中随机选择 `password_length` 个字符。
* `''.join()` 函数将字符列表连接成一个字符串。
* 输出结果将是一个 16 位随机密码。
#### 4.2.2 加密算法中的随机数
随机数在加密算法中也扮演着至关重要的角色,用于生成密钥、初始化向量和其他加密参数。
**代码块:**
```python
import random
from Crypto.Cipher import AES
# 生成一个 16 位随机密钥
key = bytes(random.getrandbits(128), 'utf-8')
# 使用密钥创建 AES 加密器
cipher = AES.new(key, AES.MODE_CBC)
# 加密消息
plaintext = 'Hello, world!'
ciphertext = cipher.encrypt(plaintext.encode('utf-8'))
```
**逻辑分析:**
* `random.getrandbits()` 函数生成一个指定位数的随机整数。
* 我们将整数转换为字节数组,以符合 AES 加密算法的密钥要求。
* `AES.new()` 函数使用密钥创建 AES 加密器。
* `cipher.encrypt()` 函数使用加密器加密明文。
### 4.3 游戏和娱乐
#### 4.3.1 随机事件生成
随机模块在游戏和娱乐领域也有广泛的应用,用于生成随机事件、角色属性和游戏元素。
**代码块:**
```python
import random
# 生成一个 0 到 100 之间的随机整数
random_number = random.randint(0, 100)
# 根据随机数生成一个随机事件
if random_number < 50:
event = 'A'
elif random_number < 75:
event = 'B'
else:
event = 'C'
```
**逻辑分析:**
* `random.randint()` 函数生成一个指定范围内的随机整数。
* 我们使用随机数来生成三个可能的事件之一。
* `event` 变量存储生成的事件。
#### 4.3.2 游戏中的随机元素
随机数还可以为游戏添加随机性和不可预测性,例如生成随机地图、敌人属性或奖励。
**代码块:**
```python
import random
# 生成一个随机地图
map_size = 10
map = [[random.randint(0, 1) for _ in range(map_size)] for _ in range(map_size)]
# 生成一个随机敌人
enemy_level = random.randint(1, 10)
enemy_health = random.randint(100, 200)
```
**逻辑分析:**
* 我们使用嵌套循环生成一个 `map_size` x `map_size` 的随机地图,其中每个元素是 0 或 1。
* 我们生成一个随机敌人,其等级和生命值在指定范围内。
* 这些随机元素为游戏提供了多样性和挑战性。
# 5. Python random模块的扩展
### 5.1 第三方库
除了Python内置的random模块,还有许多第三方库提供了更高级的随机数生成功能。这些库通常提供了更广泛的分布、更复杂的随机数序列生成算法,以及其他有用的功能。
#### 5.1.1 numpy.random
NumPy是用于科学计算的Python库,它提供了强大的随机数生成功能。NumPy.random模块提供了各种随机数分布的生成器,包括正态分布、指数分布、伽马分布等。它还提供了生成随机数组和随机矩阵的函数。
```python
import numpy as np
# 生成正态分布的随机数
random_numbers = np.random.normal(size=100)
# 生成随机数组
random_array = np.random.rand(3, 4)
```
#### 5.1.2 scikit-learn
Scikit-learn是一个用于机器学习的Python库,它也提供了随机数生成功能。Scikit-learn.utils.random模块提供了生成随机划分、随机采样和随机排列的函数。
```python
from sklearn.utils import random
# 生成随机划分
train_indices, test_indices = random.train_test_split(range(100), test_size=0.25)
# 生成随机采样
random_sample = random.sample(range(100), 10)
```
### 5.2 自定义随机数生成器
除了使用内置的random模块和第三方库,您还可以创建自己的自定义随机数生成器。这允许您控制随机数生成过程的各个方面,例如种子、分布和序列生成算法。
#### 5.2.1 继承random.Random
一种创建自定义随机数生成器的方法是继承random.Random类。Random类提供了生成各种随机数的常用方法,您可以覆盖这些方法来实现自己的算法。
```python
import random
class MyRandom(random.Random):
def __init__(self, seed):
super().__init__(seed)
def randrange(self, start, stop, step=1):
# 自定义randrange算法
pass
```
#### 5.2.2 使用装饰器
另一种创建自定义随机数生成器的更简单的方法是使用装饰器。装饰器允许您将自定义逻辑添加到现有函数中,而无需修改函数本身。
```python
import random
def custom_randrange(start, stop, step=1):
# 自定义randrange算法
pass
@custom_randrange
def randrange(start, stop, step=1):
pass
```
通过使用装饰器,您可以将自定义逻辑应用于random模块的现有randrange函数,而无需修改函数本身。
# 6. Python random模块的最佳实践和常见问题
### 6.1 随机数生成的安全性和可靠性
**安全性和可靠性**是随机数生成中的关键考虑因素。以下是一些最佳实践:
- **使用安全随机数生成器:**使用系统提供的安全随机数生成器,如 `/dev/urandom` 或 `os.urandom()`,以确保生成的随机数具有足够的熵。
- **避免可预测的种子:**不要使用时间戳或其他可预测的值作为随机种子。
- **定期重新生成种子:**定期重新生成随机种子,以防止攻击者猜测种子并预测未来的随机数。
### 6.2 避免常见的错误
以下是一些常见的随机数生成错误:
- **使用相同的种子:**使用相同的种子会导致生成相同的随机数序列。
- **生成过多随机数:**生成过多的随机数会耗尽系统资源,并可能导致性能问题。
- **不考虑随机数分布:**未考虑随机数分布可能会导致生成不符合预期分布的随机数。
- **使用不合适的函数:**选择不合适的随机数生成函数可能会导致生成不符合要求的随机数。
### 6.3 性能优化技巧
以下是一些优化 Python random模块性能的技巧:
- **缓存随机数:**将经常使用的随机数缓存起来,以避免重复生成。
- **使用并行处理:**如果可能,使用并行处理来生成随机数,以提高性能。
- **使用第三方库:**使用第三方库,如 `numpy.random`,可以提供更快的随机数生成。
0
0
相关推荐
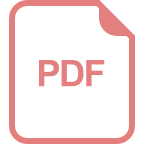
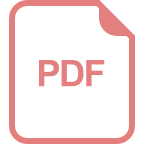
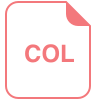
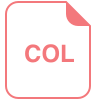
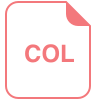
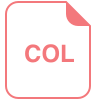

