Python求和代码与区块链:求和在区块链中的共识机制应用
发布时间: 2024-06-19 03:50:00 阅读量: 8 订阅数: 11 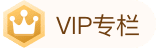
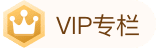
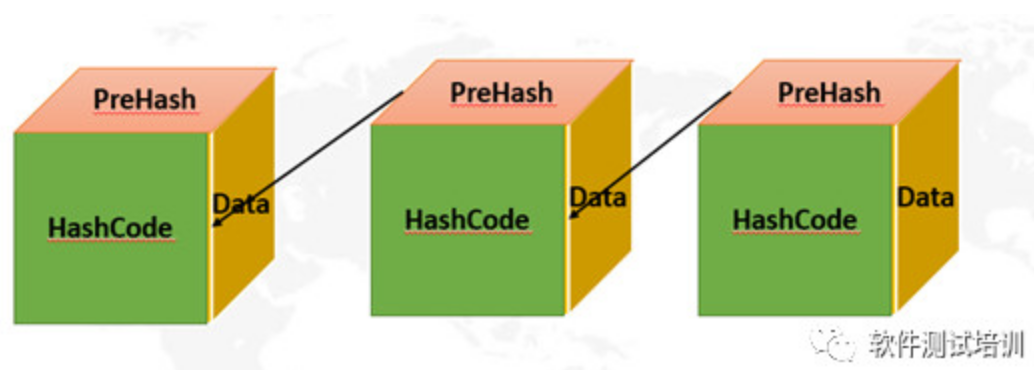
# 1. Python求和代码的基础**
Python求和代码是一种用于计算一组数字总和的强大工具。其语法简单,仅需使用`sum()`函数,即可轻松实现求和操作。例如,求和列表`[1, 2, 3, 4, 5]`,只需`sum([1, 2, 3, 4, 5])`即可得到结果15。此外,`sum()`函数还支持对字典、元组等其他可迭代对象进行求和,为数据处理提供了极大的便利性。
# 2. Python求和代码的应用
### 2.1 区块链中的共识机制
区块链技术是一种分布式账本技术,它通过共识机制来确保网络中的所有节点对账本上的交易达成一致。共识机制是区块链系统中至关重要的组成部分,它决定了区块链的安全性、效率和可扩展性。
### 2.1.1 工作量证明
工作量证明(PoW)是比特币等区块链系统中使用的一种共识机制。在PoW机制中,矿工通过解决复杂的数学难题来竞争记账权。第一个解决难题的矿工将获得记账权,并将其记账信息打包成区块添加到区块链中。
### 2.1.2 权益证明
权益证明(PoS)是另一种共识机制,它基于节点持有的代币数量。在PoS机制中,拥有更多代币的节点更有可能被选为记账人。这与PoW机制中依靠算力竞争记账权不同,PoS机制更加节能环保。
### 2.1.3 委托权益证明
委托权益证明(DPoS)是PoS机制的一种变体,它允许代币持有者将他们的投票权委托给其他节点。被委托的节点称为见证人,他们负责记账和验证交易。DPoS机制可以提高共识效率,但它也可能导致权力集中。
### 2.2 Python求和代码在共识机制中的应用
Python求和代码在区块链共识机制中有着广泛的应用。例如:
### 2.2.1 工作量证明中的应用
在PoW机制中,矿工需要解决一个复杂的数学难题,即哈希难题。哈希难题的难度与区块链网络的算力有关。矿工使用Python求和代码来计算哈希值,并不断调整哈希值中的随机数,直到找到一个满足难度要求的哈希值。
```python
import hashlib
def find_nonce(difficulty, target):
"""
Find a nonce that satisfies the difficulty requirement.
Args:
difficulty: The difficulty of the hash puzzle.
target: The target hash value.
Returns:
A nonce that satisfies the difficulty requirement.
"""
nonce = 0
while True:
hash_value = hashlib.sha256(str(nonce).encode('utf-8')).hexdigest()
if hash_value[:difficulty] == target:
return nonce
nonce += 1
```
### 2.2.2 权益证明中的应用
在PoS机制中,节点需要持有足够的代币才能参与共识。节点的投票权与他们持有的代币数量成正比。Python求和代码可以用来计算节点的投票权,并根据投票权来选择记账人。
```python
def calculate_voting_power(balances):
"""
Calculate the voting power of each node.
Args:
balances: A dictionary of node addresses to their balances.
Returns:
A dictionary of node addresses to their voting power.
"""
total_balance = sum(balances.values())
voting_power = {}
for address, balance in balances.items():
voting_power[address] = balance / total_balance
return voting_power
```
### 2.2.3 委托权益证明中的应用
在DPoS机制中,代币持有者可以将他们的投票权委托给见证人。Python求和代码可以用来计算见证人的总投票权,并根据总投票权来选择见证人。
```python
def calculate_witness_voting_power(delegations):
"""
Calculate the total voting power of each witness.
Args:
delegations: A dictionary of witness addresses to their delegations.
Returns:
A dictionary of witness addresses to their total voting power.
"""
total_voting_power = sum(delegations.values())
witness_voting_power = {}
for witness, delegation in delegations.items():
witness_voting_power[witness] = delegation / total_voting_power
return witness_voting_power
```
# 3.1 代码优化技术
**3.1.1 循环优化**
循环优化主要针对循环语句进行优化,常见技术包括:
- **循环展开:**将循环体中的代码复制到循环外,减少循环次数。
- *
0
0
相关推荐





