区块链中的密码学:密码学技术与加密标准
发布时间: 2024-01-28 15:30:58 阅读量: 42 订阅数: 38 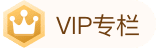
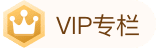
# 1. 区块链技术概述
## 1.1 区块链的基本原理
区块链是一种去中心化的分布式账本技术,其基本原理包括以下几个关键点:
- **去中心化**:区块链中的数据存储在网络中的多个节点上,不存在一个中心化的控制机构。每个节点均可参与到交易的验证和数据存储中,形成一个共识机制。
- **分布式账本**:区块链上的每一笔交易都被记录在一个称为“区块”的容器中,并按照时间顺序连接成一个链状结构的账本,保证了交易的不可篡改性和透明性。
- **共识机制**:区块链采用一种共识算法,确保网络中的各个节点对于交易的验证和顺序的达成一致,常见的共识机制包括工作量证明(Proof of Work)和权益证明(Proof of Stake)等。
## 1.2 区块链的应用领域
区块链技术具有去中心化、不可篡改、透明等特点,适用于多个领域的应用,包括但不限于:
- **数字货币**:比特币是第一个基于区块链技术的加密货币,区块链技术可以实现加密货币的发行、交易和结算。
- **供应链金融**:区块链可以追踪商品的来源,确保供应链中的透明度和可信度,同时实现跨境支付和融资等功能。
- **身份认证**:区块链可以提供分布式身份认证技术,在数字世界中确保用户的身份安全和隐私保护。
## 1.3 区块链技术的发展与挑战
区块链技术自诞生以来已经发展了多个阶段,主要包括以下几个发展阶段:
1. 初期阶段:比特币的兴起,引爆了区块链技术的热潮,并推动了区块链的初步发展。
2. 平台建设阶段:各大企业和机构开始积极投入研发和建设自己的区块链平台,以探索区块链技术在各个行业的应用。
3. 标准化与合作阶段:随着区块链技术的逐渐成熟,国际组织和标准化机构开始制定相关标准,并促进跨界合作。
4. 实际应用阶段:区块链技术的应用逐渐从概念验证阶段向实际商业应用转变,涉及金融、供应链、医疗等多个领域。
然而,区块链技术还面临一些挑战,包括扩展性、隐私保护、能源消耗等问题,未来仍需不断突破和创新。
# 2. 密码学基础
密码学是区块链技术中至关重要的一部分,它提供了保护数据安全性和隐私的基础。本章将介绍密码学的基本概念和核心技术。
### 2.1 对称加密与非对称加密
在密码学中,对称加密和非对称加密是两种常见的加密方式。
#### 2.1.1 对称加密
对称加密算法使用相同的密钥来进行加密和解密操作。常见的对称加密算法有AES、DES和3DES等。
以下是一个使用Python实现的对称加密例子:
```python
import hashlib
from Crypto.Cipher import AES
def encrypt(key, plaintext):
cipher = AES.new(hashlib.sha256(key.encode()).digest(), AES.MODE_ECB)
ciphertext = cipher.encrypt(plaintext)
return ciphertext
def decrypt(key, ciphertext):
cipher = AES.new(hashlib.sha256(key.encode()).digest(), AES.MODE_ECB)
plaintext = cipher.decrypt(ciphertext)
return plaintext
key = "mysecretkey"
plaintext = "Hello, world!"
ciphertext = encrypt(key, plaintext)
decrypted_text = decrypt(key, ciphertext)
print("Ciphertext:", ciphertext)
print("Decrypted Text:", decrypted_text)
```
代码说明:
- 密钥`key`和明文`plaintext`通过AES算法进行加密,并打印出密文`ciphertext`。
- 密文`ciphertext`通过同样的密钥`key`进行解密,并打印出解密后的明文`decrypted_text`。
#### 2.1.2 非对称加密
非对称加密算法使用公钥和私钥两个不同的密钥进行加密和解密操作。常见的非对称加密算法有RSA和ECC等。
以下是一个使用Java实现的非对称加密例子:
```java
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import javax.crypto.Cipher;
public class AsymmetricEncryptionExample {
public static void main(String[] args) throws Exception {
String plaintext = "Hello, world!";
// 生成密钥对
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
KeyPair keyPair = keyPairGenerator.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// 加密
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] ciphertext = cipher.doFinal(plaintext.getBytes());
// 解密
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decryptedText = cipher.doFinal(ciphertext);
System.out.println("Ciphertext: " + new String(ciphertext));
System.out.println("Decrypted Text: " + new String(decryptedText));
}
}
```
代码说明:
- 生成RSA密钥对,并使用公钥`publicKey`进行加密操作。
- 使用私钥`privateKey`进行解密操作,并打印出解密后的明文`decryptedText`。
### 2.2 数字签名与哈希函数
#### 2.2.1 数字签名
数字签名是保证数据完整性和验证身份的重要方式。它使用私钥对数据进行签名,使用公钥对签名进行验证。
以下是一个使用Go实现的数字签名例子:
```go
package main
import (
"crypto"
"crypto/rand"
"crypto/rsa"
"crypto/sha256"
"fmt"
)
func main() {
plaintext := []byte("Hello, world!")
// 生成RSA密钥对
privateKey, _ := rsa.GenerateKey(rand.Reader, 2048)
publicKey := &privateKey.PublicKey
// 对数据进行数字签名
hashed := sha256.Sum256(plaintext)
signature, _ := rsa.SignPKCS1v15(rand.Reader, privateKey, crypto.SHA256, hashed[:])
// 验证数字签名
err := rsa.VerifyPKCS1v15(publicKey, crypto.SHA256, hashed[:], signature)
if err != nil {
fmt.Println("Signature verification failed.")
} else {
fmt.Println("Signature verification succeeded.")
}
}
```
代码说明:
- 生成RSA密钥对,并使用私钥`privateKey`对数据进行签名。
- 使用公钥`publicKey`对签名进行验证,如果验证成功,则打印"Signature verification succeeded."。
#### 2.2.2 哈希函数
哈希函数是将任意长度的输入数据转换为固定长度的输出,且输出的结果是不可逆的。
以下是一个使用JavaScript实现的哈希函数例子:
```javascript
const crypto = require('crypto');
const plaintext = 'Hello, world!';
const hash = crypto.createHash('sha256');
hash.update(plaintext);
const hashedText = hash.digest('hex');
console.log('Hashed Text:', hashedText);
```
代码说明:
- 使用Node.js内置的`crypto`模块,创建一个SHA256的哈希函数对象`hash`。
- 将明文`plaintext`输入到哈希函数中,并通过`digest`方法获取哈希结果`hashedText`。
- 打印出哈希结果。
### 2.3 公钥基础设施(PKI)的作用
公钥基础设施(PKI)是为了解决公钥分发问题而引入的一套体系,它包括了证书颁发机构(CA)、数字证书和证书验证等组成部分。
以下是一个使用Java实现的使用PKI进行数字签名的例子:
```java
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.Signature;
import java.util.Base64;
public class PkiExample {
public static void main(String[] args) throws Exception {
String plaintext = "Hello, world!";
// 生成RSA密钥对
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
KeyPair keyPair = keyPairGenerator.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// 数字签名
```
0
0
相关推荐
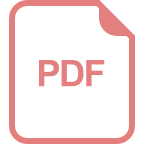
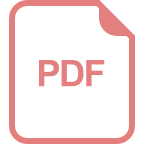
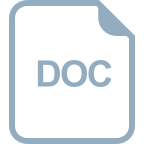
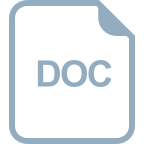
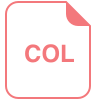
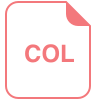
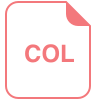
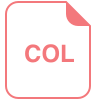