Jenkins高级用法:深入了解Jenkins的高级功能与技巧
发布时间: 2024-03-06 00:49:51 阅读量: 13 订阅数: 19 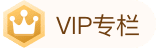
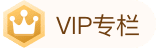
# 1. Jenkins Pipeline as Code
在现代软件开发环境中,构建与部署管道的自动化已经成为开发团队不可或缺的一部分。然而,当简单的构建管道不再满足复杂的项目需求时,Jenkins Pipeline as Code 提供了一种强大的方式来定义构建流程,允许团队以代码的形式管理与执行持续集成与交付流程。
#### 1.1 Pipeline DSL介绍
Jenkins Pipeline DSL(Domain Specific Language)是一种基于Groovy的领域特定语言,用于描述构建流水线的各个阶段、任务和逻辑。通过Pipeline DSL,开发人员可以灵活地定义并组织复杂的构建流程,实现从代码提交到部署的自动化流程。
```groovy
pipeline {
agent any
stages {
stage('Checkout') {
steps {
// Checkout source code
git 'https://github.com/example.git'
}
}
stage('Build') {
steps {
// Build the project
sh 'mvn clean package'
}
}
stage('Test') {
steps {
// Run tests
sh 'mvn test'
}
}
stage('Deploy') {
steps {
// Deploy to staging environment
sh 'kubectl apply -f deployment.yaml'
}
}
}
}
```
**代码总结:** 上述代码展示了一个简单的Pipeline DSL示例,包括代码拉取、构建、测试和部署阶段。通过DSL的方式,我们可以清晰地定义整个流程。
#### 1.2 使用Groovy编写Pipeline
Groovy作为Jenkins Pipeline的核心语言,为开发人员提供了丰富的功能和灵活性。通过Groovy脚本,我们可以编写复杂的逻辑、自定义函数以及与外部系统交互,从而实现定制化的构建与部署流程。
```groovy
def deployToProd() {
// Deployment logic to production environment
sh 'kubectl apply -f production.yaml'
}
pipeline {
agent any
stages {
stage('Checkout') {
steps {
git 'https://github.com/example.git'
}
}
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy to Staging') {
steps {
sh 'kubectl apply -f staging.yaml'
}
}
stage('Promote to Production') {
steps {
script {
if (env.BRANCH_NAME == 'main') {
deployToProd()
} else {
echo 'Skipping production deployment for feature branches'
}
}
}
}
}
}
```
**代码总结:** 在这个示例中,我们定义了一个自定义函数`deployToProd()`用于将应用部署到生产环境。通过Groovy的脚本能力,我们可以根据分支名称决定是否进行生产环境部署。
#### 1.3 实例:构建复杂的多阶段Pipeline
现实项目中,构建流水线可能涉及更多的阶段、并行任务以及复杂逻辑。下面是一个示例,演示了一个包含多个阶段和并行任务的高级Pipeline:
```groovy
pipeline {
agent any
stages {
stage('Initialize') {
steps {
script {
// Initialization steps
}
}
}
stage('Build & Test') {
parallel {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Unit Tests') {
steps {
sh 'mvn test'
}
}
stage('Integration Tests') {
steps {
sh 'mvn integration-test'
}
}
}
}
stage('Deploy to Staging') {
steps {
sh 'kubectl apply -f staging.yaml'
}
}
stage('Approve Deployment') {
input {
message 'Approve to deploy to production?
```
0
0
相关推荐
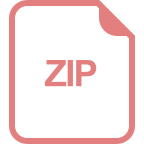
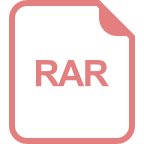
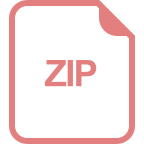
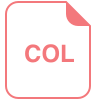
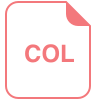
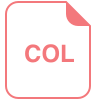
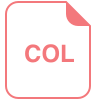

