Python装饰器的定义与高级用法详解
发布时间: 2024-02-23 05:49:56 阅读量: 49 订阅数: 32 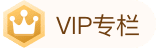
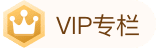
# 1. Python装饰器简介
## 1.1 什么是装饰器
装饰器是Python中一种非常强大的技术,它实际上是一个函数,可以让其他函数在不需要做任何代码修改的前提下增加额外功能,装饰器的返回也是一个函数。
```python
def decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@decorator
def say_hello():
print("Hello!")
say_hello()
```
**代码说明:**
- 通过装饰器`@decorator`来修饰函数`say_hello`,在`say_hello`函数执行前后可以添加额外的功能,而不需要修改`say_hello`函数本身。
- 输出:
```
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
```
## 1.2 装饰器的作用和优势
装饰器可以实现横切关注点(cross-cutting concerns),比如日志、性能测试、事务等代码的复用,提高了代码的可重用性和可维护性。
## 1.3 装饰器的基本语法及使用方式
```python
def decorator(func):
def wrapper(*args, **kwargs):
# 在调用被装饰函数之前的操作
result = func(*args, **kwargs)
# 在调用被装饰函数之后的操作
return result
return wrapper
```
装饰器是通过`@`符号放在函数的上一行来实现的,如`@decorator`。
# 2. 装饰器的原理解析
装饰器是Python中非常重要且灵活的功能,其原理其实并不复杂,理解了装饰器的原理,才能更好地灵活运用装饰器。
### 2.1 函数作为参数
在Python中,函数可以作为参数传递给另一个函数,这为装饰器的实现提供了基础。通过将函数作为参数传递给装饰器函数,实现对被装饰函数的修改或增强。
```python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
def say_hello():
print("Hello!")
say_hello = my_decorator(say_hello)
say_hello()
```
**注释:** 上述代码中,`my_decorator`函数接受一个函数作为参数,并定义内部函数`wrapper`来实现装饰的功能,然后返回`wrapper`函数来代替原始的`say_hello`函数。
**代码总结:** 函数作为参数是装饰器实现的基础,可以通过定义装饰器函数来修改或增强被装饰函数的功能。
**结果说明:** 执行以上代码,会在调用`say_hello`函数前后分别输出"Something is happening before the function is called."和"Something is happening after the function is called."。
### 2.2 函数嵌套
在Python中函数是一等对象,可以在函数内部定义函数,这为装饰器的嵌套提供了可能性。
```python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
```
**注释:** 上述代码中,通过在`say_hello`函数的上方添加`@my_decorator`,相当于执行了`say_hello = my_decorator(say_hello)`,实现了对`say_hello`函数的装饰。
**代码总结:** 函数嵌套是装饰器实现的一种形式,通过在被装饰函数上方添加`@decorator_func`,实现对被装饰函数的装饰功能。
**结果说明:** 执行以上代码,会在调用`say_hello`函数前后分别输出"Something is happening before the function is called."和"Something is happening after the function is called."。
### 2.3 返回函数
装饰器函数内部通常会定义一个内部函数,并返回这个内部函数,实现对被装饰函数的包裹。
```python
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
```
0
0
相关推荐








