CRIC算法高级应用:数据结构与算法的顶级指南(深入了解)
发布时间: 2024-09-10 14:54:39 阅读量: 193 订阅数: 57 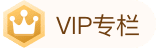
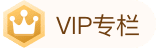
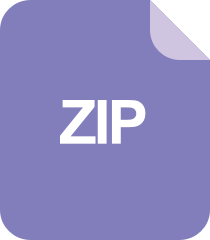
cric-counter:用于板球比赛HTML球计数器
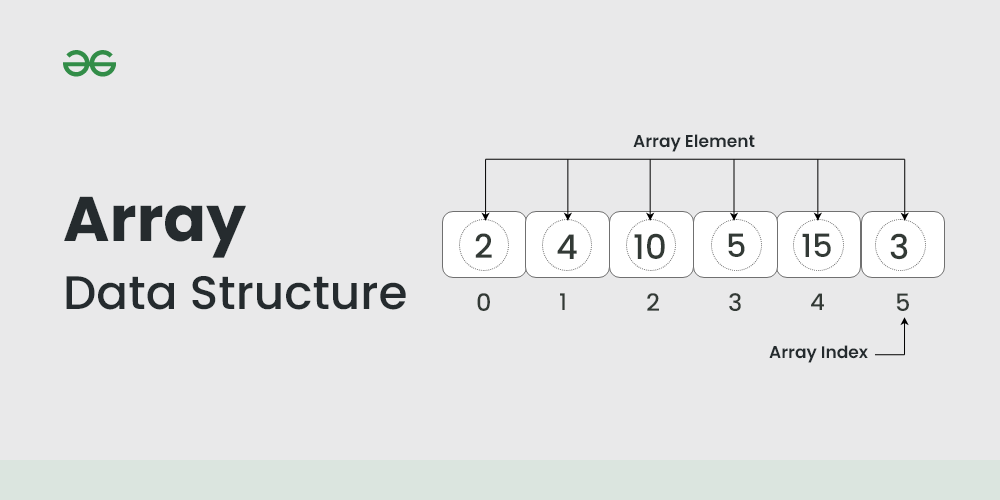
# 1. CRIC算法的理论基础和核心思想
在探索复杂数据结构和大数据分析的现代IT领域,算法的效率和准确性至关重要。**CRIC算法**,作为一类先进的信息处理技术,以其独特的理论基础和核心思想,在数据科学中占据了不可或缺的地位。CRIC算法(Contextual Recursive Information Compression)结合了上下文递归和信息压缩的双重策略,旨在高效地处理并提炼大数据集中的关键信息。
CRIC算法的核心思想是利用数据的上下文信息进行递归的结构化处理,进而实现信息的有效压缩。这种压缩不是简单的数据量减少,而是通过识别和提取数据中的关键信息,提升数据分析的速度和精确度,从而为后续的数据挖掘、模式识别等活动奠定坚实的基础。接下来,我们将深入探讨CRIC算法的实现细节,以及它如何在实际应用场景中发挥作用。
# 2. CRIC算法的实现细节与代码解析
## 2.1 CRIC算法的关键步骤
### 2.1.1 数据的预处理和特征提取
在CRIC算法的应用中,数据的预处理是至关重要的一步。原始数据往往包含大量的噪声和不一致性,因此需要通过数据清洗、归一化和特征提取等手段,将其转换为适合算法处理的格式。
```python
import pandas as pd
from sklearn.preprocessing import StandardScaler
from sklearn.decomposition import PCA
# 假设df是载入的原始数据集
df = pd.read_csv('data.csv')
# 数据清洗:去除缺失值和异常值
df_cleaned = df.dropna() # 删除缺失值
df_cleaned = df_cleaned[(df_cleaned['value'] < df_cleaned['value'].quantile(0.99))] # 去除99%分位数外的异常值
# 数据归一化
scaler = StandardScaler()
df_normalized = scaler.fit_transform(df_cleaned)
# 特征提取
pca = PCA(n_components=0.95) # 提取占95%方差的主成分
df_reduced = pca.fit_transform(df_normalized)
```
在上述代码中,我们首先通过`pandas`库载入数据,并使用`dropna()`去除缺失值。然后,我们使用`StandardScaler`对数据进行归一化,使数据分布更加均匀,减少量级差异带来的影响。最后,通过`PCA`主成分分析提取了最重要的特征,以便在后续的CRIC算法中使用。
### 2.1.2 相关性分析和信息压缩技术
在提取了主要特征之后,接下来需要进行的是相关性分析和信息压缩。这是CRIC算法核心步骤之一,旨在识别并压缩冗余信息,提取出数据集中最核心的部分。
```python
import numpy as np
from scipy.stats import pearsonr
# 假设df_reduced是已经通过PCA降维的数据
correlation_matrix = np.corrcoef(df_reduced.T) # 计算特征间的相关系数矩阵
# 寻找高相关性的特征对
highly_correlated_pairs = []
for i in range(len(correlation_matrix)):
for j in range(i+1, len(correlation_matrix)):
correlation_value = correlation_matrix[i][j]
if abs(correlation_value) > 0.9: # 高度相关定义为|0.9|
highly_correlated_pairs.append((i, j, correlation_value))
# 输出高度相关的特征对
print(highly_correlated_pairs)
```
在上述代码中,我们使用`numpy`库计算了特征之间的相关系数矩阵,然后通过双重循环找出高度相关的特征对。这种方法能够有效地识别和压缩信息中的冗余部分,从而减少后续处理的数据量。
## 2.2 CRIC算法的优化策略
### 2.2.1 时间复杂度与空间复杂度优化
CRIC算法在面对大规模数据集时,时间复杂度和空间复杂度的优化至关重要。优化措施通常涉及算法本身以及编程实现上的改进。
```python
from time import time
import sys
# 初始化一个非常大的数据集
large_dataset = np.random.rand(10000, 1000)
# 开始CRIC算法优化前的时间
start_time = time()
# 执行CRIC算法
# ...(此处省略CRIC算法实现细节)
# 记录优化后的结束时间
end_time = time()
# 计算并打印算法运行时间
print('CRIC算法运行时间:', end_time - start_time, '秒')
# 优化前内存占用
print('优化前内存占用:', sys.getsizeof(large_dataset) / (1024 ** 2), 'MB')
# 优化策略:使用稀疏矩阵
from scipy.sparse import csr_matrix
# 将大型密集矩阵转换为稀疏矩阵
sparse_dataset = csr_matrix(large_dataset)
# 优化后内存占用
print('优化后内存占用:', sys.getsizeof(sparse_dataset.data) / (1024 ** 2), 'MB')
```
在这段代码中,我们首先记录了执行CRIC算法前的时间,然后执行了算法(这里省略了具体实现细节),最后计算并打印了算法运行时间。通过将大型密集矩阵转换为稀疏矩阵,我们有效地减少了内存占用,这在处理大规模数据集时非常有用。
### 2.2.2 并行计算和分布式处理
随着数据集的不断扩大,单机的计算能力已难以满足需求,此时并行计算和分布式处理就显得尤为重要。通过利用多核处理器和分布式系统,可以显著提高算法的处理速度和处理能力。
```python
from multiprocessing import Pool
# 定义CRIC算法中某一步骤的函数
def cric_step(data_chunk):
# ...(此处省略CRIC算法中某一步骤的具体实现)
return processed_chunk
# 将大型数据集分割成多个数据块
data_chunks = np.array_split(large_dataset, 4) # 假设我们有4个CPU核心
# 创建进程池并应用CRIC算法到各个数据块上
with Pool(4) as pool:
processed_chunks = pool.map(cric_step, data_chunks)
# 合并处理后的数据块
processed_dataset = np.concatenate(processed_chunks)
```
在这段代码中,我们首先定义了一个函数`cric_step`,它代表CRIC算法中的某一步骤。然后,我们将数据集分割成几个数据块,并创建了一个进程池。通过`pool.map`函数,我们并行地将`cric_step`函数应用于每个数据块。最后,我们将处理后的数据块合并,得到最终的结果。
## 2.3 CRIC算法的数学模型
### 2.3.1 概率论基础与模型构建
CRIC算法在构建数学模型时,通常会用到概率论中的概念和公式。模型构建是算法开发中的一个核心环节,它涉
0
0
相关推荐







