自动文本摘要的算法和应用
发布时间: 2024-01-17 20:13:28 阅读量: 15 订阅数: 13 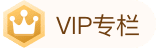
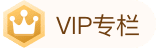
# 1. 文本摘要概述
## 1.1 文本摘要的定义和作用
文本摘要是指将文本中的重要信息提炼出来,以便让读者在较短的篇幅内获得全文的主要内容。文本摘要在信息检索、搜索引擎、自动问答等领域有着广泛的应用。
## 1.2 自动文本摘要与人工摘要的对比
自动文本摘要是指利用计算机程序自动提取文本中的关键信息,而人工摘要则是由人工阅读全文后进行提炼。自动文本摘要能够提高效率并应用于大规模文本处理任务,而人工摘要受限于时间和人力资源。
## 1.3 自动文本摘要的发展历程
随着自然语言处理和机器学习技术的不断发展,自动文本摘要经历了从基于统计特征的方法到深度学习模型的演进,实现了在不同场景下更加准确和有效的信息提取。
# 2. 文本摘要的算法原理
### 2.1 抽取式文本摘要算法
抽取式文本摘要算法是基于对原始文本的统计分析和抽取关键信息的方法来生成摘要。这种算法通过识别关键词、短语或句子来表示文本的主要内容,并将其组合成一个简明扼要的摘要。常见的抽取式文本摘要算法有:
- **基于词频统计的算法**:将文本中出现频率较高的词语作为关键词,并按照一定规则组合生成摘要。
```python
def word_frequency(text):
word_freq = {}
words = text.split()
for word in words:
if word in word_freq:
word_freq[word] += 1
else:
word_freq[word] = 1
return word_freq
def extract_summary(text):
word_freq = word_frequency(text)
sorted_freq = sorted(word_freq.items(), key=lambda x:x[1], reverse=True)
summary_length = int(len(sorted_freq) * 0.3) # 提取前30%的关键词
summary = [word[0] for word in sorted_freq[:summary_length]]
return ' '.join(summary)
```
- **基于句子位置和长度的算法**:根据句子在文本中的位置和长度来判断其重要性,选择相对重要的句子生成摘要。
```python
def extract_summary(text, summary_length):
sentences = text.split('. ')
sorted_sentences = sorted(sentences, key=lambda x:(len(x), -sentences.index(x)), reverse=True)
summary = sorted_sentences[:summary_length]
return '. '.join(summary)
```
### 2.2 归纳式文本摘要算法
归纳式文本摘要算法是通过对原始文本进行理解和归纳,将其主要内容转化为新的表达方式,生成摘要。这种算法往往需要借助自然语言处理和机器学习等技术来实现。常见的归纳式文本摘要算法有:
- **基于文本统计特征的算法**:通过对文本的结构、语法、词法等进行分析和建模,提取出文本的统计特征,并根据这些特征生成摘要。
```python
import nltk
from nltk.tokenize import sent_tokenize, word_tokenize
def extract_summary(text):
sentences = sent_tokenize(text)
words = word_tokenize(text)
word_freq = nltk.FreqDist(words)
top_words = word_freq.most_common(10) # 提取出现频率最高的10个词语
summary = [word[0] for word in top_words]
return ' '.join(summary)
```
- **基于图模型的算法**:将文本表示为图的形式,节点表示词语或句子,边表示词语或句子之间的关系,通过图的分析和图算法来生成摘要。
```python
import networkx as nx
def extract_summary(text):
sentences = sent_tokenize(text)
words = word_tokenize(text)
word_graph = nx.Graph()
word_graph.add_nodes_from(words)
for i in range(len(sentences)):
sentence_words = word_tokenize(sentences[i])
for j in range(len(sentence_words
```
0
0
相关推荐
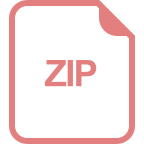
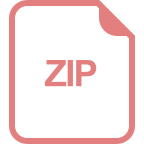
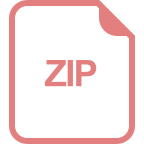





