UVM中的Component对象构建技巧
发布时间: 2024-03-29 06:49:38 阅读量: 46 订阅数: 32 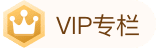
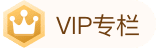

uvm1.2 lab uvm1.2 workshop
# 1. 介绍UVM框架
在本章中,我们将介绍UVM框架的概述,包括UVM框架的概念、架构和组成部分,以及重点关注UVM中的Component对象的作用和重要性。让我们一起深入了解UVM框架!
# 2. Component对象基础
在这一章节中,我们将深入探讨UVM中的Component对象基础知识,包括Component的定义和特性、构建和实例化方法以及生命周期管理等内容。让我们一起来了解吧!
# 3. 优化Component对象的继承与扩展
在UVM中,Component对象的继承和扩展是非常常见的操作,可以通过继承已有的Component对象来扩展其功能,提高代码的复用性和可维护性。然而,在进行继承和扩展时,需要注意一些技巧和最佳实践,以避免潜在的问题。
#### 3.1 使用继承来扩展Component对象
通过继承一个已有的Component对象,可以在不改变原有功能的基础上,添加新的功能或调整现有功能。这种方式可以有效减少重复代码的编写,提高代码的可重用性。
```python
class BaseComponent(UVMComponent):
// BaseComponent的实现代码
class ExtendedComponent(BaseComponent):
// 在BaseComponent的基础上扩展新功能的实现代码
```
在上面的代码示例中,ExtendedComponent继承自BaseComponent,可以方便地使用BaseComponent已有的功能,并在此基础上扩展新的功能。
#### 3.2 避免多重继承导致的问题
在进行Component对象的继承时,需要注意避免多重继承可能带来的问题。多重继承会增加代码的复杂性,容易导致代码的混乱和不易维护。
#### 3.3 使用Interface提高Component对象的通用性
除了继承外,还可以使用Interface来提高Component对象的通用性。通过定义接口,不同的Component对象可以实现相同的接口,从而实现更好的代码解耦和灵活性。
```python
interface MyInterface;
function void doSomething();
endinterface
class MyComponent extends UVMComponent implements MyInterface;
// 实现MyInterface定义的方法
endclass
```
通过使用Interface,可以实现不同Component对象之间的通用性,减少耦合度,提高代码的灵活性和可维护性。
# 4. Component对象的配置和参数化
在UVM中,Component对象的配置和参数化是非常重要的,它们能够增强测试环境的灵活性和可维护性。本章将重点讨论如何通过配置和参数化Component对象来实现这一目的。
#### 4.1 通过Config Objects配置Component对象
在UVM中,通过Config Objects的方式为Component对象进行配置是一种常见的做法。Config Objects是一种用于存储配置信息的数据结构,可以在运行时动态地为Component对象提供不同的配置参数。
##### 示例代码:
```python
import uvm
class MyConfig(uvm.uvm_object):
def __init__(self, name="MyConfig"):
super().__init__(name)
self.param1 = 0
self.param2 = "default"
class MyComponent(uvm.uvm_component):
def __init__(self, name, parent):
super().__init__(name, parent)
self.config = MyConfig()
def build_phase(self):
super().build_phase()
uvm_coreservice_t.get().set_config_int(self, "config.param1", 42)
uvm_coreservice_t.get().set_config_string(self, "config.param2", "custom_value")
def connect_phase(self):
super().connect_phase()
uvm_info("MyComponent", f"Param1: {self.config.param1}, Param2: {self.config.param2}", uvm.LOW)
```
##### 代码解释:
- `MyConfig`类定义了需要配置的参
0
0
相关推荐
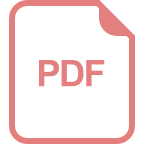
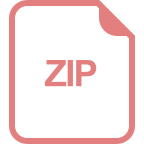
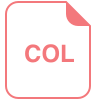
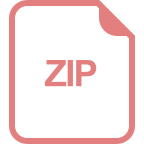
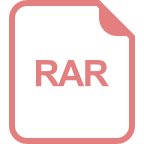
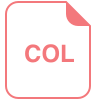
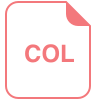
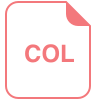