【OpenCV缺陷检测实战宝典】:从零基础到实战应用的全面指南
发布时间: 2024-08-09 17:47:27 阅读量: 64 订阅数: 27 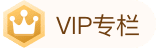
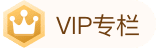
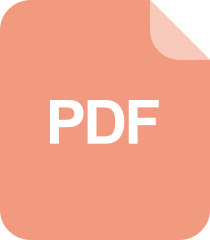
Opencv C++图像处理全面指南:从环境搭建到实战案例解析
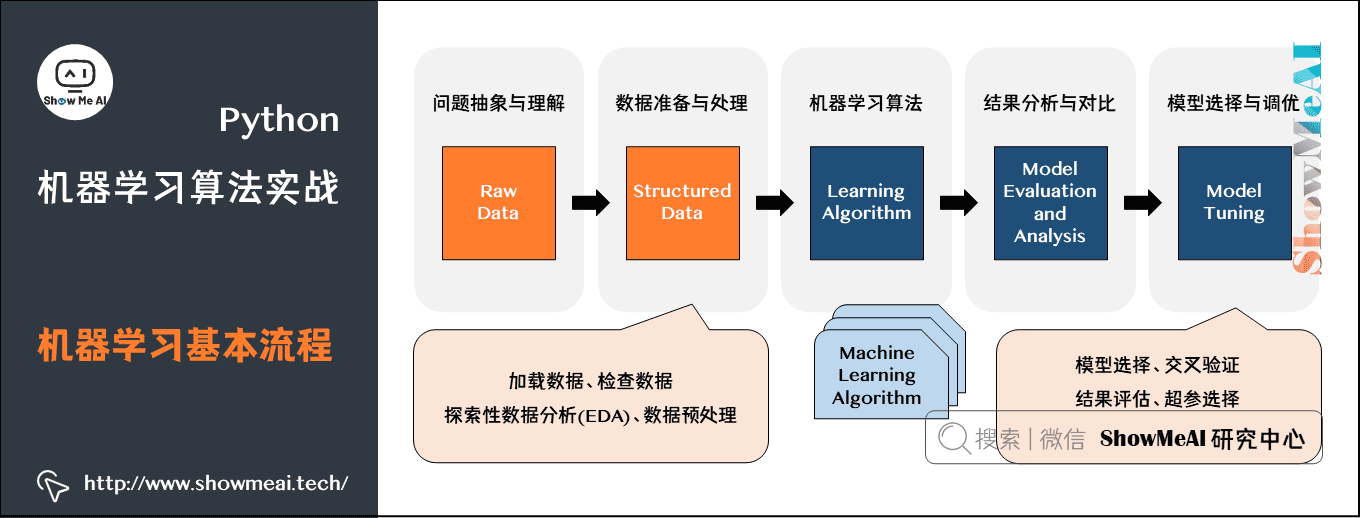
# 1. OpenCV基础理论**
OpenCV(Open Source Computer Vision Library)是一个开源计算机视觉库,广泛应用于图像处理、视频分析和计算机视觉等领域。它提供了丰富的算法和函数,用于图像的获取、处理、分析和识别。
OpenCV的核心概念包括:
* **图像:**一个二维数组,存储像素值。
* **像素:**图像中的最小单位,具有颜色和位置信息。
* **通道:**图像中表示不同颜色分量的数组,通常为RGB或灰度。
* **矩阵:**一个二维数组,用于表示图像或其他数据结构。
# 2.1 图像预处理
图像预处理是图像处理中的一个重要步骤,它可以改善图像的质量,为后续的图像处理任务做好准备。OpenCV提供了丰富的图像预处理函数,包括图像灰度化、二值化、平滑和锐化。
### 2.1.1 图像灰度化和二值化
**图像灰度化**将彩色图像转换为灰度图像,即只包含亮度信息的图像。这可以简化后续的处理,因为灰度图像仅有一个通道,而不是彩色图像的三个通道。OpenCV中的`cvtColor()`函数可用于将彩色图像转换为灰度图像:
```python
import cv2
# 读取彩色图像
image = cv2.imread('image.jpg')
# 将彩色图像转换为灰度图像
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 显示灰度图像
cv2.imshow('Gray Image', gray_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**图像二值化**将灰度图像转换为二值图像,即只有黑色和白色像素的图像。这可以进一步简化图像,并用于分割和识别对象。OpenCV中的`threshold()`函数可用于对灰度图像进行二值化:
```python
# 对灰度图像进行二值化
thresh_image = cv2.threshold(gray_image, 127, 255, cv2.THRESH_BINARY)[1]
# 显示二值图像
cv2.imshow('Thresholded Image', thresh_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 2.1.2 图像平滑和锐化
**图像平滑**可以去除图像中的噪声和细节,使图像更加平滑。OpenCV中的`blur()`函数可用于对图像进行平滑,它提供了多种平滑算法,如均值滤波、高斯滤波和中值滤波。
```python
# 对图像进行均值滤波
blurred_image = cv2.blur(image, (5, 5))
# 显示平滑后的图像
cv2.imshow('Blurred Image', blurred_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**图像锐化**可以增强图像中的边缘和细节,使图像更加清晰。OpenCV中的`Laplacian()`函数可用于对图像进行锐化,它使用拉普拉斯算子来检测图像中的边缘。
```python
# 对图像进行锐化
sharpened_image = cv2.Laplacian(image, cv2.CV_64F)
# 显示锐化后的图像
cv2.imshow('Sharpened Image', sharpened_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
# 3. OpenCV缺陷检测实践
### 3.1 缺陷检测算法概述
缺陷检测算法旨在从图像中识别和定位缺陷或异常。这些算法可分为两大类:传统方法和深度学习方法。
**3.1.1 传统缺陷检测方法**
传统缺陷检测方法通常基于图像处理技术,如:
- **边缘检测:**识别图像中的边缘和轮廓,从而突出缺陷区域。
- **阈值分割:**将图像像素分为缺陷和非缺陷区域。
- **形态学操作:**使用数学形态学操作,如膨胀和腐蚀,来增强缺陷区域。
这些方法通常需要手动特征工程和参数调整,并且在复杂图像中可能难以获得准确的结果。
**3.1.2 深度学习缺陷检测方法**
深度学习缺陷检测方法利用卷积神经网络(CNN)等深度学习模型,直接从图像中学习缺陷特征。这些方法通常具有更高的精度和鲁棒性,但需要大量标记数据进行训练。
### 3.2 缺陷检测实战项目
**3.2.1 缺陷图像数据集的获取和预处理**
缺陷图像数据集是缺陷检测模型训练和评估的基础。获取数据集时,应注意以下几点:
- **数据多样性:**数据集应包含各种缺陷类型、尺寸和背景。
- **数据标注:**缺陷区域应准确标注,以确保模型学习正确的特征。
- **数据预处理:**图像应进行预处理,如调整大小、归一化和数据增强,以提高模型性能。
**3.2.2 缺陷检测模型的训练和评估**
缺陷检测模型的训练和评估是一个迭代的过程,包括以下步骤:
- **模型选择:**选择合适的CNN模型,如 ResNet 或 VGGNet。
- **训练:**使用标记数据集训练模型,调整模型参数和超参数以优化性能。
- **评估:**使用未见数据集评估模型的精度、召回率和 F1 分数等指标。
- **优化:**根据评估结果,调整模型架构、训练参数或数据预处理技术,以提高模型性能。
**代码块 1:缺陷检测模型训练代码**
```python
import tensorflow as tf
# 加载数据集
train_dataset = tf.keras.preprocessing.image_dataset_from_directory(
"path/to/train_dataset",
label_mode="binary",
batch_size=32,
image_size=(224, 224),
)
# 定义模型
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation="relu", input_shape=(224, 224, 3)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation="relu"),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(128, activation="relu"),
tf.keras.layers.Dense(1, activation="sigmoid"),
])
# 编译模型
model.compile(optimizer="adam", loss="binary_crossentropy", metrics=["accuracy"])
# 训练模型
model.fit(train_dataset, epochs=10)
```
**代码逻辑分析:**
- 使用 `image_dataset_from_directory` 函数加载训练数据集。
- 定义一个卷积神经网络模型,包括卷积层、池化层、全连接层和激活函数。
- 编译模型,指定优化器、损失函数和评估指标。
- 使用 `fit` 方法训练模型,指定训练数据集和训练轮数。
**参数说明:**
- `path/to/train_dataset`:训练数据集路径。
- `label_mode="binary"`:将标签设置为二进制(缺陷/非缺陷)。
- `batch_size=32`:每个训练批次的大小。
- `image_size=(224, 224)`:输入图像的大小。
- `epochs=10`:训练轮数。
# 4.1 实时缺陷检测系统
### 4.1.1 缺陷检测算法的优化
在实时缺陷检测系统中,算法的效率至关重要。以下是一些优化缺陷检测算法的技巧:
- **并行处理:**利用多核处理器或GPU并行处理图像,提高处理速度。
- **图像金字塔:**使用图像金字塔对图像进行降采样,在较小分辨率的图像上进行缺陷检测,减少计算量。
- **区域划分:**将图像划分为较小的区域,并仅对感兴趣区域进行缺陷检测。
- **算法选择:**选择适合实时处理的算法,如轻量级卷积神经网络或基于阈值的算法。
### 4.1.2 实时图像采集和处理
实时缺陷检测系统需要实时采集和处理图像。以下是一些关键步骤:
- **图像采集:**使用高速相机或视频流采集图像。
- **图像预处理:**对图像进行预处理,包括灰度化、二值化、平滑等。
- **缺陷检测:**应用优化后的缺陷检测算法检测图像中的缺陷。
- **缺陷标记:**将检测到的缺陷标记在图像上,以便可视化和进一步分析。
### 代码示例
以下代码示例展示了如何使用OpenCV进行实时缺陷检测:
```python
import cv2
# 初始化摄像头
cap = cv2.VideoCapture(0)
# 设置图像预处理参数
gray_threshold = 127
blur_kernel_size = 5
# 主循环
while True:
# 读取帧
ret, frame = cap.read()
# 图像预处理
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (blur_kernel_size, blur_kernel_size), 0)
thresh = cv2.threshold(blur, gray_threshold, 255, cv2.THRESH_BINARY)[1]
# 缺陷检测
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 缺陷标记
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 0, 255), 2)
# 显示结果
cv2.imshow("实时缺陷检测", frame)
# 按下 ESC 键退出
if cv2.waitKey(1) & 0xFF == 27:
break
# 释放摄像头
cap.release()
cv2.destroyAllWindows()
```
### 参数说明
- `gray_threshold`:灰度化阈值
- `blur_kernel_size`:高斯模糊内核大小
- `contours`:检测到的缺陷轮廓
- `x, y, w, h`:缺陷边界框的坐标和尺寸
### 流程图
以下流程图展示了实时缺陷检测系统的流程:
```mermaid
graph LR
subgraph 图像采集
A[摄像头采集图像] --> B[预处理]
end
subgraph 缺陷检测
C[灰度化] --> D[二值化] --> E[平滑] --> F[缺陷检测]
end
subgraph 缺陷标记
G[缺陷标记] --> H[显示结果]
end
A --> B --> C --> D --> E --> F --> G --> H
```
# 5. OpenCV缺陷检测案例分析**
**5.1 汽车零部件缺陷检测**
**应用领域:**汽车制造、质量控制
**缺陷类型:**划痕、凹痕、裂纹、气泡
**检测方法:**
- 图像预处理:灰度化、二值化、平滑
- 边缘检测:Canny算子
- 轮廓提取:轮廓查找算法
- 缺陷识别:基于形状和尺寸的规则判断
**代码示例:**
```python
import cv2
# 图像预处理
img = cv2.imread('car_part.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (5, 5), 0)
thresh = cv2.threshold(blur, 127, 255, cv2.THRESH_BINARY)[1]
# 边缘检测
edges = cv2.Canny(thresh, 100, 200)
# 轮廓提取
contours, _ = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 缺陷识别
defects = []
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
if w > 10 and h > 10 and cv2.contourArea(contour) > 100:
defects.append((x, y, w, h))
# 绘制缺陷
for defect in defects:
cv2.rectangle(img, (defect[0], defect[1]), (defect[0] + defect[2], defect[1] + defect[3]), (0, 0, 255), 2)
# 显示结果
cv2.imshow('Defect Detection', img)
cv2.waitKey(0)
```
**5.2 工业产品缺陷检测**
**应用领域:**电子制造、机械加工
**缺陷类型:**毛刺、裂纹、变形
**检测方法:**
- 图像预处理:灰度化、直方图均衡化
- 纹理分析:局部二值模式(LBP)
- 缺陷识别:基于LBP特征的分类器
**代码示例:**
```python
import cv2
import numpy as np
# 图像预处理
img = cv2.imread('industrial_product.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
equ = cv2.equalizeHist(gray)
# 纹理分析
lbp = cv2.xfeatures2d.LBP_create(radius=3, npoints=8, uniform=True)
lbp_features = lbp.compute(equ)[1].flatten()
# 缺陷识别
model = cv2.ml.SVM_create()
model.load('defect_classifier.xml')
prediction = model.predict(np.array([lbp_features]))[1]
# 绘制缺陷
if prediction == 1:
cv2.putText(img, 'Defect Detected', (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
# 显示结果
cv2.imshow('Defect Detection', img)
cv2.waitKey(0)
```
**5.3 医疗影像缺陷检测**
**应用领域:**医疗诊断、影像分析
**缺陷类型:**肿瘤、结节、出血
**检测方法:**
- 图像预处理:增强对比度、去噪
- 深度学习:卷积神经网络(CNN)
- 缺陷识别:基于CNN模型的分类
**代码示例:**
```python
import cv2
import tensorflow as tf
# 图像预处理
img = cv2.imread('medical_image.jpg')
preprocessed = cv2.normalize(img, None, 0, 255, cv2.NORM_MINMAX)
# 深度学习
model = tf.keras.models.load_model('medical_defect_classifier.h5')
prediction = model.predict(np.expand_dims(preprocessed, axis=0))[0]
# 缺陷识别
if prediction[1] > 0.5:
cv2.putText(img, 'Defect Detected', (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
# 显示结果
cv2.imshow('Defect Detection', img)
cv2.waitKey(0)
```
0
0
相关推荐
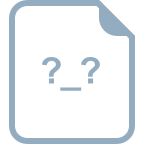
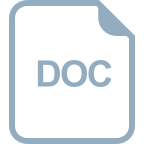
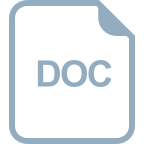
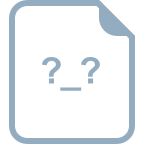
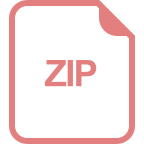
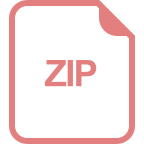
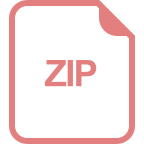