format方法和字符串模板方法的对比与区别
发布时间: 2024-04-10 22:01:43 阅读量: 65 订阅数: 22 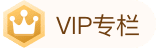
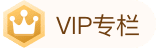
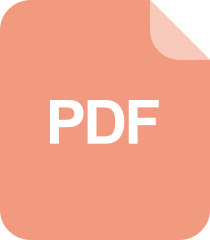
python字符串连接方法分析
# 1. **介绍**
在本章节中,我们将介绍format方法与字符串模板方法的基本概念及区别,帮助读者更好地理解其使用方法和优劣势。
1.1 了解format方法与字符串模板方法
- **format方法**:是一种字符串格式化的方法,在Python中常用于字符串格式化输出。
- **字符串模板方法**:是使用模板来替换字符串中的占位符,有助于提高代码的可读性。
通过对这两种方法的比较,我们可以更好地选择适合特定场景的方法,提高代码的效率和可维护性。
# 2. **语法**
在本章节中,我们将详细介绍format方法与字符串模板方法的语法。
#### 2.1 format方法的语法
format方法的语法非常灵活,主要包括如下几个部分:
- 基本语法:使用大括号{}作为占位符
- 索引:通过索引来指定参数的顺序
- 命名参数:为占位符指定具体参数
- 格式说明符:控制如何格式化值
- 嵌套使用:在一个占位符中嵌套其他占位符
下面是一个简单的示例代码:
```python
# 使用基本的format方法
print("Hello, {}!".format("World"))
# 使用索引指定参数顺序
print("I am {1}. My favorite number is {0}.".format(7, "Alice"))
# 使用命名参数
print("Welcome, {name}! Your age is {age}.".format(name="Bob", age=25))
```
#### 2.2 字符串模板方法的语法
字符串模板方法的语法相对简单明了,只需要使用`$`符号和`{}`来引用变量即可。
下面是一个基本的示例代码:
```python
from string import Template
# 使用字符串模板方法
tpl = Template("Hello, $name!")
print(tpl.substitute(name="World"))
```
接下来,我们将通过流程图进一步比较format方法与字符串模板方法的语法差异。
```mermaid
graph TD
A[format方法] --> B{使用大括号{}作为占位符}
B --> C[索引指定参数顺序]
B --> D[命名参数]
B --> E[格式说明符]
B --> F[嵌套使用]
G[字符串模板方法] --> H[$符号和{}引用变量]
```
通过以上内容,我们对format方法与字符串模板方法的语法有了更深入的了解。在下一节中,我们将探讨它们的具体使用方式。
# 3. 使用方式
在本节中,将详细介绍如何使用`format`方法和字符串模板方法。
1. **如何使用format方法**:
使用`format`方法进行字符串格式化时,可以在字符串中使用花括号`{}`作为占位符,然后通过`format`方法传入相应的参数进行替换。下面是一个简单的示例代码:
```python
# 使用format方法进行字符串格式化
name = "Alice"
age = 30
formatted_string = "My name is {} and I am {} years old".format(name, age)
print(formatted_string)
```
运行以上代码,将输出:"My name is Alice and I am 30 years old"。
2. **如何使用字符串模板方法**:
字符串模板方法可以使用`Template`模块提供的`substitute`方法进行字符串模板替换。需要注意的是,字符串模板方法使用`$`符号加上变量名作为占位符。以下是一个示例代码:
```python
from string import Template
# 使用字符串模板方法进行字符串格式化
name = "Bob"
age = 25
template = Template("My name is $name and I am $age years old")
formatted_string = template.substitute(name=name, age=age)
print(formatted_string)
```
运行以上代码,将输出:"My name is Bob and I am 25 years old"。
3. **比较使用方式**:
| 方法 | 占位符 | 替换方法 |
|---------------|-------------|----------------|
| `format`方法 | `{}` | `format()` |
| 字符串模板方法 | `$变量名` | `substitute()` |
4. **流程图**:
下面是一个使用Mermaid格式的流程图,展示了使用`format
0
0
相关推荐
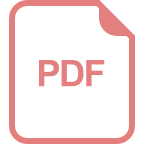
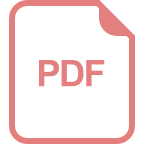
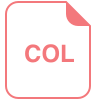
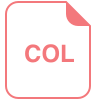
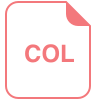
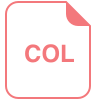
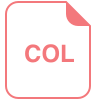
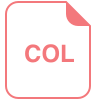