format方法在网络编程中的字符串处理技能
发布时间: 2024-04-10 22:16:34 阅读量: 9 订阅数: 11 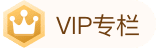
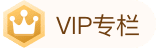
# 1. format方法在网络编程中的字符串处理技能
## 1. 介绍
### 什么是format方法
格式化字符串是在编程中经常遇到的操作,它使我们能够动态地插入变量值、格式化文本并创建更具可读性和可维护性的代码。`format()` 方法是Python字符串类的一个重要方法之一,它提供了一种灵活的机制来格式化字符串。
### format方法在字符串处理中的重要性
在网络编程中,处理各种请求参数、构建响应数据或处理数据库查询语句等场景时,经常需要对字符串进行格式化处理。format方法提供了一种简洁而强大的工具,使得我们可以轻松地处理不同类型的数据并构建复杂的字符串。
### 示例代码
```python
# 使用format方法格式化字符串
name = "Alice"
age = 30
formatted_string = "My name is {} and I am {} years old".format(name, age)
print(formatted_string)
```
#### 示例输出:
```
My name is Alice and I am 30 years old
```
### format方法的重要性
- 方便处理各种数据类型
- 提高代码可读性和可维护性
- 避免拼接字符串时的繁琐操作
# 2. 基础用法
### format方法基本语法
在Python中,我们可以使用`format`方法来进行字符串格式化,其基本语法如下:
```python
# 使用位置参数
"{} {}".format(value1, value2)
# 使用关键字参数
"{name} {age}".format(name="Alice", age=30)
```
### 示例:简单的字符串格式化
下面是一个简单的示例,演示了如何使用`format`方法进行字符串的格式化:
```python
# 使用位置参数
name = "Alice"
age = 30
print("My name is {}, and I am {} years old.".format(name, age))
# 使用关键字参数
print("My name is {name}, and I am {age} years old.".format(name="Bob", age=25))
```
执行上述代码,将输出:
```
My name is Alice, and I am 30 years old.
My name is Bob, and I am 25 years old.
```
### 使用位置参数
可以通过位置参数的顺序来填充字符串中的占位符,示例如下:
1. 使用位置参数 `format` 函数进行字符串格式化
2. 将参数按照顺序填充到字符串中的占位符中
3. 输出格式化后的字符串
### 使用关键字参数
也可以通过关键字参数来指定填充占位符的值,示例如下:
1. 使用关键字参数 `format` 函数进行字符串格式化
3. 用关键字参数来指定填充占位符的值
4. 打印出经过格式化的字符串
下面是这两种方法的具体用法,旨在帮助读者更好地理解`format`方法的基础用法。
# 3. format方法的进阶技巧
在实际的编程应用中,我们经常需要使用format方法来灵活处理字符串。下面将介绍format方法的一些进阶技巧,包括使用位置参数、关键字参数以及格式化数字等特殊数据类型。
### 使用位置参数
使用位置参数可以根据参数在格式字符串中的位置来进行格式化,示例如下:
```python
# 使用位置参数
age = 25
name = "Alice"
formatted_str = "My name is {1} and I am {0} years old.".format(age, name)
print(formatted_str)
```
输出结果为:
```
My name is Alice and I am 25 years old.
```
### 使用关键字参数
通过使用关键字参数,我们可以在格式化字符串时指定参数的名称,这样可以提高代码的可读性,示例如下:
```python
# 使用关键字参数
order = 123
product = "Smartphone"
formatted_order = "Order number: {order}, Product: {product}".format(order=order, product=product)
print(formatted_order)
```
输出结果为:
```
Order number: 123, Product: Smartphone
```
### 格式化数字等特殊数据类型
除了字符串,我们也可以使用format方法来格式化数字等特殊数据类型,例如设置小数位数或千位分隔符,示例如下:
```python
# 格式化数字
pi = 3.141592653
```
0
0
相关推荐
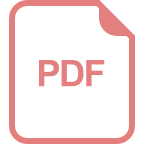
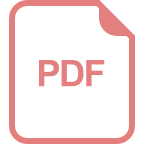
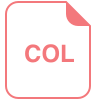
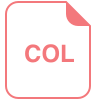
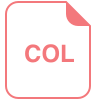
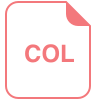

