【VSCode OpenCV 入门指南】:零基础到精通的完整教程
发布时间: 2024-08-06 08:25:09 阅读量: 51 订阅数: 45 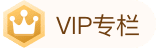
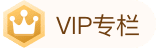
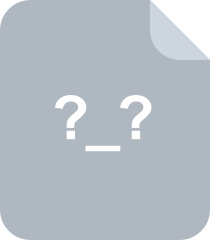
OpenCV开发指南:从入门到实战项目
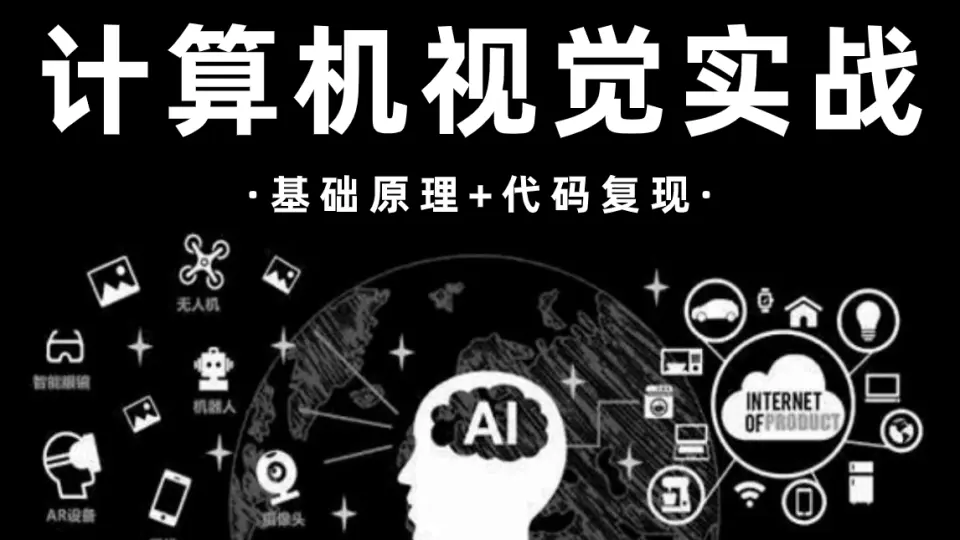
# 1. VSCode OpenCV 入门
OpenCV(Open Source Computer Vision Library)是一个开源的计算机视觉库,为图像处理、视频分析和机器学习提供了广泛的功能。本章将介绍如何在 VSCode 中设置 OpenCV 开发环境,为后续的 OpenCV 实践应用奠定基础。
### 1.1 安装 VSCode 和 OpenCV 扩展
首先,需要安装 VSCode 和 OpenCV 扩展。在 VSCode 中,转到扩展市场,搜索并安装 "OpenCV" 扩展。该扩展将提供 OpenCV 代码的语法高亮、代码完成和调试支持。
### 1.2 配置 OpenCV 开发环境
接下来,需要配置 OpenCV 开发环境。在 VSCode 中,转到 "文件" > "首选项" > "设置"。在搜索栏中输入 "OpenCV",然后找到 "OpenCV: Path to OpenCV libraries" 设置。将此路径设置为 OpenCV 库的安装目录。
# 2. OpenCV 基础理论
### 2.1 图像处理基础
#### 2.1.1 图像表示和存储
图像在计算机中通常以矩阵形式表示,其中每个元素对应图像中一个像素的值。像素值表示该像素的颜色强度或其他属性。
**图像格式:**
* **位图 (BMP):** 未压缩的图像格式,文件体积较大。
* **JPEG (JPG):** 有损压缩格式,可显著减小文件体积,但会损失图像质量。
* **PNG:** 无损压缩格式,可保持图像质量,但文件体积较大。
* **TIFF:** 高质量无损格式,常用于专业图像处理。
**图像数据类型:**
* **uint8:** 无符号 8 位整数,表示 0-255 范围内的值。
* **int8:** 有符号 8 位整数,表示 -128-127 范围内的值。
* **float32:** 32 位浮点数,表示小数和负值。
#### 2.1.2 图像处理常用算法
**图像增强:**
* **直方图均衡化:** 调整图像直方图,增强图像对比度。
* **伽马校正:** 调整图像亮度和对比度。
* **锐化:** 增强图像边缘,提高清晰度。
**图像转换:**
* **灰度转换:** 将彩色图像转换为灰度图像。
* **二值化:** 将图像转换为黑白图像。
* **颜色空间转换:** 将图像从一种颜色空间转换为另一种颜色空间,如 RGB 到 HSV。
**图像几何变换:**
* **缩放:** 改变图像尺寸。
* **旋转:** 旋转图像。
* **透视变换:** 纠正图像透视失真。
### 2.2 OpenCV 库简介
#### 2.2.1 OpenCV 的历史和发展
OpenCV(Open Source Computer Vision Library)是一个开源计算机视觉库,最初由 Intel 开发。
* **1999 年:** OpenCV 项目启动。
* **2000 年:** OpenCV 1.0 发布。
* **2009 年:** OpenCV 2.0 发布,引入 C++ 接口。
* **2015 年:** OpenCV 3.0 发布,支持 Python 和 Java 接口。
* **2018 年:** OpenCV 4.0 发布,引入深度学习支持。
#### 2.2.2 OpenCV 的模块和功能
OpenCV 包含多个模块,涵盖图像处理、计算机视觉、机器学习等领域。
**主要模块:**
* **Core:** 基本图像处理和数据结构。
* **Imgproc:** 图像处理算法。
* **Highgui:** 图像输入输出和用户界面。
* **Ml:** 机器学习算法。
* **Objdetect:** 物体检测和识别。
* **Video:** 视频处理。
**功能示例:**
* 图像读取和保存
* 图像转换和增强
* 图像分析和识别
* 机器学习和计算机视觉算法
# 3. OpenCV 实践应用
### 3.1 图像读取、显示和保存
#### 3.1.1 使用 OpenCV 读写图像
OpenCV 提供了多种函数来读取和写入图像。最常用的函数是 `cv2.imread()` 和 `cv2.imwrite()`。
**cv2.imread()** 函数用于读取图像。它接受两个参数:
* 图像文件的路径
* 标志,指定图像的读取方式
标志可以是以下值之一:
* `cv2.IMREAD_COLOR`:读取彩色图像
* `cv2.IMREAD_GRAYSCALE`:读取灰度图像
* `cv2.IMREAD_UNCHANGED`:读取图像而不进行任何转换
**示例代码:**
```python
import cv2
# 读取彩色图像
image = cv2.imread('image.jpg', cv2.IMREAD_COLOR)
# 读取灰度图像
image = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
```
**cv2.imwrite()** 函数用于写入图像。它接受两个参数:
* 图像文件的路径
* 图像数据
**示例代码:**
```python
import cv2
# 写入彩色图像
cv2.imwrite('image.jpg', image)
```
#### 3.1.2 图像的显示和保存
**显示图像**
OpenCV 提供了 `cv2.imshow()` 函数来显示图像。它接受两个参数:
* 图像窗口的名称
* 要显示的图像
**示例代码:**
```python
import cv2
# 显示图像
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**保存图像**
除了 `cv2.imwrite()` 函数,OpenCV 还提供了 `cv2.imwrite()` 函数来保存图像。它接受两个参数:
* 图像文件的路径
* 图像数据
**示例代码:**
```python
import cv2
# 保存图像
cv2.imwrite('image.jpg', image)
```
### 3.2 图像处理操作
#### 3.2.1 图像转换和增强
**图像转换**
OpenCV 提供了多种函数来转换图像的格式和颜色空间。最常用的函数是:
* `cv2.cvtColor()`:转换图像的颜色空间
* `cv2.resize()`:调整图像的大小
* `cv2.flip()`:翻转图像
**示例代码:**
```python
import cv2
# 将图像转换为灰度图像
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 将图像调整为一半大小
half_image = cv2.resize(image, (0, 0), fx=0.5, fy=0.5)
# 将图像水平翻转
flipped_image = cv2.flip(image, 1)
```
**图像增强**
OpenCV 提供了多种函数来增强图像的对比度、亮度和锐度。最常用的函数是:
* `cv2.equalizeHist()`:均衡图像的直方图
* `cv2.adjustGamma()`:调整图像的伽马值
* `cv2.GaussianBlur()`:对图像进行高斯模糊
**示例代码:**
```python
import cv2
# 均衡图像的直方图
eq_image = cv2.equalizeHist(image)
# 调整图像的伽马值
gamma_image = cv2.adjustGamma(image, 1.5)
# 对图像进行高斯模糊
blur_image = cv2.GaussianBlur(image, (5, 5), 0)
```
#### 3.2.2 图像几何变换
**图像几何变换**
OpenCV 提供了多种函数来对图像进行几何变换。最常用的函数是:
* `cv2.warpAffine()`:对图像进行仿射变换
* `cv2.warpPerspective()`:对图像进行透视变换
* `cv2.getRotationMatrix2D()`:获取图像的旋转矩阵
**示例代码:**
```python
import cv2
# 对图像进行仿射变换
affine_image = cv2.warpAffine(image, cv2.getRotationMatrix2D((image.shape[1] / 2, image.shape[0] / 2), 30, 1), (image.shape[1], image.shape[0]))
# 对图像进行透视变换
perspective_image = cv2.warpPerspective(image, cv2.getPerspectiveTransform(np.float32([[0, 0], [image.shape[1], 0], [0, image.shape[0]], [image.shape[1], image.shape[0]]]), np.float32([[0, 0], [image.shape[1], 0], [0, image.shape[0]], [image.shape[1], image.shape[0]]])), (image.shape[1], image.shape[0]))
```
### 3.3 图像分析和识别
#### 3.3.1 图像分割和边缘检测
**图像分割**
图像分割是将图像分解成不同区域的过程。OpenCV 提供了多种图像分割算法,包括:
* `cv2.threshold()`:基于阈值的分割
* `cv2.kmeans()`:基于 K 均值的分割
* `cv2.watershed()`:基于分水岭的分割
**示例代码:**
```python
import cv2
# 基于阈值的分割
thresh_image = cv2.threshold(image, 127, 255, cv2.THRESH_BINARY)[1]
# 基于 K 均值的分割
kmeans_image = cv2.kmeans(image, 3, None, (cv2.TERM_CRITERIA_EPS + cv2.TERM_CRITERIA_MAX_ITER, 10, 1.0), 10, cv2.KMEANS_RANDOM_CENTERS)[1]
# 基于分水岭的分割
watershed_image = cv2.watershed(image, np.zeros((image.shape[0], image.shape[1]), dtype=np.uint8), None, (-1, -1, -1, -1), cv2.WSECT_FLOOD)
```
**边缘检测**
边缘检测是检测图像中强度变化的边界。OpenCV 提供了多种边缘检测算法,包括:
* `cv2.Canny()`:Canny 边缘检测
* `cv2.Sobel()`:Sobel 边缘检测
* `cv2.Laplacian()`:拉普拉斯边缘检测
**示例代码:**
```python
import cv2
# Canny 边缘检测
canny_image = cv2.Canny(image, 100, 200)
# Sobel 边缘检测
sobel_image = cv2.Sobel(image, cv2.CV_64F, 1, 0, ksize=5)
# 拉普拉斯边缘检测
laplacian_image = cv2.Laplacian(image, cv2.CV_64F)
```
#### 3.3.2 物体检测和识别
**物体检测**
物体检测是检测图像中特定物体的过程。OpenCV 提供了多种物体检测算法,包括:
* `cv2.CascadeClassifier()`:基于级联分类器的物体检测
* `cv2.HOGDescriptor()`:基于梯度直方图的物体检测
* `cv2.YOLO()`:基于 You Only Look Once 的物体检测
**示例代码:**
```python
import cv2
# 基于级联分类器的物体检测
cascade_classifier = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
faces = cascade_classifier.detectMultiScale(image, 1.1, 4)
# 基于梯度直方图的物体检测
hog_descriptor = cv2.HOGDescriptor()
hog_features = hog_descriptor.compute(image)
hog_classifier = cv2.SVM()
hog_classifier.train(hog_features, np.array([1, 0]))
hog_predictions = hog_classifier.predict(hog_features)
# 基于 You Only Look Once 的物体检测
yolo_net = cv2.dnn.readNetFromDarknet('yolov3.cfg', 'yolov3.weights')
yolo_blob = cv2.dnn.blobFromImage(image, 1 / 255.0, (416, 416), (0, 0, 0), swapRB=True, crop=False)
yolo_net.setInput(yolo_blob)
yolo_detections = yolo_net.forward()
```
**物体识别**
物体识别是识别图像中特定物体的过程。OpenCV 提供了多种物体识别算法,包括:
* `cv2.face_landmark_model()`:基于人脸关键点的物体识别
* `cv2.face_recognition_model()`:基于人脸识别的物体识别
* `cv2.object_detection_model
# 4. OpenCV 进阶应用
### 4.1 OpenCV 与机器学习
#### 4.1.1 机器学习基础
机器学习是一种人工智能技术,它使计算机能够在没有明确编程的情况下从数据中学习。机器学习算法可以从数据中识别模式并做出预测。
机器学习算法分为两类:
- **监督学习:**使用标记数据进行训练,其中输入数据与预期的输出数据相关联。
- **无监督学习:**使用未标记数据进行训练,其中输入数据与输出数据之间没有明确的关联。
#### 4.1.2 OpenCV 中的机器学习模块
OpenCV 提供了广泛的机器学习模块,包括:
- **分类:**用于预测数据点属于哪个类别。
- **回归:**用于预测连续值。
- **聚类:**用于将数据点分组到不同的簇中。
- **降维:**用于减少数据点的维度。
- **特征提取:**用于从数据中提取有意义的特征。
### 4.2 OpenCV 与计算机视觉
#### 4.2.1 计算机视觉基础
计算机视觉是人工智能的一个分支,它使计算机能够从图像和视频中理解世界。计算机视觉算法可以检测和识别对象、跟踪运动、估计深度和生成 3D 模型。
#### 4.2.2 OpenCV 中的计算机视觉模块
OpenCV 提供了强大的计算机视觉模块,包括:
- **图像处理:**用于增强和转换图像。
- **特征检测和描述:**用于从图像中提取特征。
- **对象检测和识别:**用于检测和识别图像中的对象。
- **运动分析:**用于跟踪图像和视频中的运动。
- **立体视觉:**用于从多个图像中生成 3D 模型。
**代码示例:**
```python
import cv2
# 加载图像
image = cv2.imread("image.jpg")
# 使用 Haar 级联分类器检测人脸
face_cascade = cv2.CascadeClassifier("haarcascade_frontalface_default.xml")
faces = face_cascade.detectMultiScale(image, 1.1, 4)
# 在图像中绘制人脸边界框
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 2)
# 显示检测结果
cv2.imshow("Faces Detected", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**代码逻辑分析:**
1. 加载图像并将其存储在 `image` 变量中。
2. 创建一个 Haar 级联分类器,该分类器用于检测人脸。
3. 使用分类器在图像中检测人脸。
4. 遍历检测到的所有人脸,并使用矩形在图像中绘制边界框。
5. 显示检测结果。
**参数说明:**
- `cv2.CascadeClassifier(path)`:创建 Haar 级联分类器,其中 `path` 是分类器文件的路径。
- `detectMultiScale(image, scaleFactor, minNeighbors)`:检测图像中的人脸,其中 `image` 是输入图像,`scaleFactor` 是检测图像缩放比例,`minNeighbors` 是检测人脸所需的最小相邻矩形数量。
- `rectangle(image, pt1, pt2, color, thickness)`:在图像中绘制矩形,其中 `image` 是输入图像,`pt1` 和 `pt2` 是矩形的两个对角点,`color` 是矩形颜色,`thickness` 是矩形线宽。
- `imshow(windowName, image)`:显示图像,其中 `windowName` 是图像窗口名称,`image` 是要显示的图像。
- `waitKey(delay)`:等待键盘输入,其中 `delay` 是等待时间(以毫秒为单位)。
- `destroyAllWindows()`:关闭所有 OpenCV 窗口。
# 5. VSCode OpenCV 开发环境配置
### 5.1 VSCode 环境搭建
#### 5.1.1 安装 VSCode 和 OpenCV 扩展
1. 下载并安装 Visual Studio Code(VSCode)。
2. 在 VSCode 中安装 OpenCV 扩展。打开 VSCode,点击扩展图标,搜索 "OpenCV",然后点击 "Install" 按钮。
#### 5.1.2 配置 OpenCV 开发环境
1. 在 VSCode 中打开 "Settings",搜索 "C/C++: IntelliSense Mode",并将其设置为 "msvc-x64"。
2. 在 VSCode 中打开 "Tasks",点击 "Configure Task",然后选择 "C/C++: g++.exe build active file"。
3. 在 "Command" 字段中,输入以下内容:
```
g++ ${file} -o ${fileDirname}/${fileBasenameNoExtension}.exe -I${env:INCLUDE} -L${env:LIB} -lopencv_core -lopencv_imgproc -lopencv_highgui
```
4. 点击 "Save" 保存任务。
### 5.2 OpenCV 项目开发
#### 5.2.1 创建 OpenCV 项目
1. 在 VSCode 中,点击 "File" -> "New" -> "Folder",创建一个新的文件夹。
2. 在文件夹中,右键单击,选择 "Open in Terminal"。
3. 在终端中,运行以下命令:
```
mkdir src
mkdir include
mkdir build
```
4. 在 "src" 文件夹中,创建一个名为 "main.cpp" 的文件。
#### 5.2.2 编写 OpenCV 代码
在 "main.cpp" 文件中,编写以下代码:
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
// 读取图像
Mat image = imread("image.jpg");
// 显示图像
imshow("Image", image);
// 等待用户输入
waitKey(0);
// 保存图像
imwrite("output.jpg", image);
return 0;
}
```
#### 5.2.3 调试和运行 OpenCV 程序
1. 在 VSCode 中,按 `F5` 键调试程序。
2. 在终端中,程序将编译并运行。
3. 程序将显示 "Image" 窗口,其中包含加载的图像。
4. 按任意键关闭窗口。
5. 程序将保存输出图像 "output.jpg"。
0
0
相关推荐
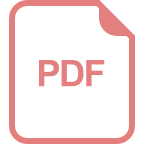
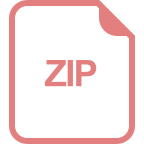
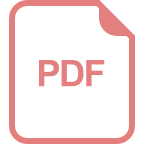
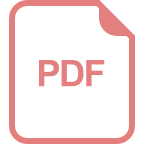
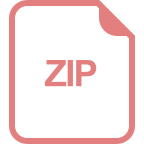
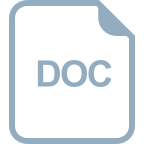
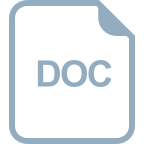
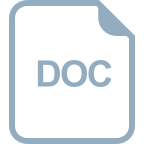