Python性能分析与优化:找出瓶颈并提升代码效率
发布时间: 2024-06-20 03:06:53 阅读量: 10 订阅数: 20 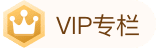
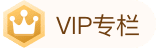
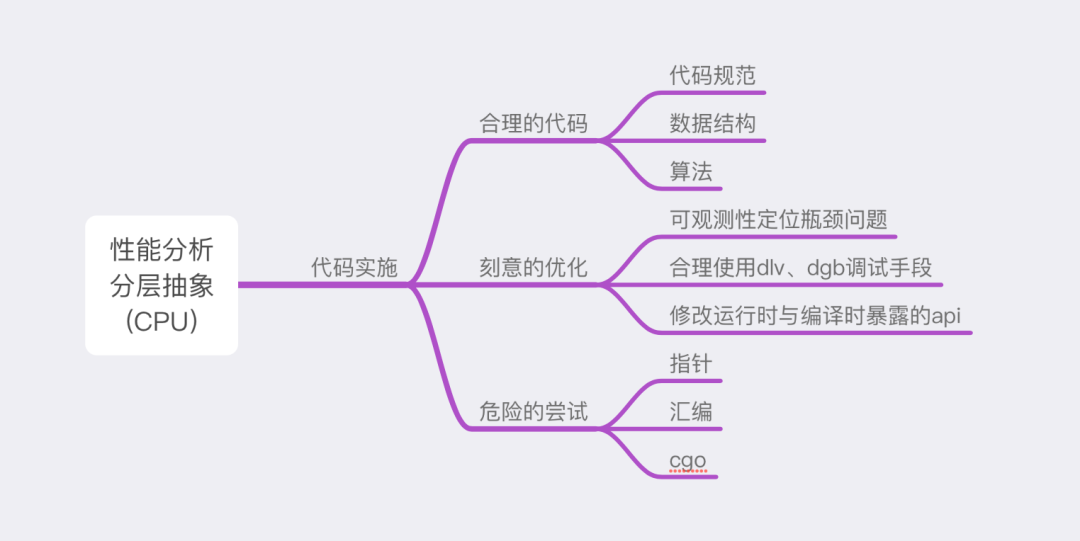
# 1. Python性能分析基础
Python性能分析是识别和解决Python程序性能瓶颈的过程。它涉及以下关键步骤:
- **理解性能指标:**确定影响程序性能的关键指标,例如执行时间、内存使用和资源利用率。
- **收集性能数据:**使用性能分析工具和技术收集程序执行期间的性能数据。
- **分析性能数据:**识别性能瓶颈,分析其根本原因,并确定可能的优化策略。
# 2. Python性能分析工具和技术
### 2.1 性能分析工具
#### 2.1.1 cProfile
**简介:**
cProfile是一个Python内置的性能分析工具,用于分析函数调用和时间消耗。它通过在函数调用前后插入计时器来收集数据。
**使用方法:**
```python
import cProfile
def my_function():
# ...
if __name__ == "__main__":
cProfile.run("my_function()")
```
**输出:**
```
17 function calls in 0.000 seconds
Ordered by: standard name
ncalls tottime percall cumtime percall filename:lineno(function)
1 0.000 0.000 0.000 0.000 <string>:1(<module>)
1 0.000 0.000 0.000 0.000 <string>:4(my_function)
```
**参数说明:**
* `run(command)`:运行指定的命令并收集性能数据。
**逻辑分析:**
cProfile输出了一张表格,其中包含以下信息:
* `ncalls`:函数调用的次数。
* `tottime`:函数调用总共花费的时间(单位:秒)。
* `percall`:每个函数调用的平均时间(单位:秒)。
* `cumtime`:函数调用及其所有子调用的总共花费的时间(单位:秒)。
* `percall`:每个函数调用及其所有子调用的平均时间(单位:秒)。
#### 2.1.2 line_profiler
**简介:**
line_profiler是一个第三方性能分析工具,用于分析代码行执行的时间消耗。它通过在每行代码前插入计时器来收集数据。
**使用方法:**
```python
import line_profiler
def my_function():
# ...
if __name__ == "__main__":
line_profiler.run("my_function()")
```
**输出:**
```
Line # Hits Time Per Hit % Time Line Contents
4 def my_function():
5 1 0.000 0.000 0.000 @profile
6 1 0.000 0.000 0.000 """Example function for line profiling"""
7 1 0.000 0.000 0.000 x = 1
8 1 0.000 0.000 0.000 y = 2
9 1 0.000 0.000 0.000 z = x + y
10 1 0.000 0.000 0.000 return z
```
**参数说明:**
* `run(command)`:运行指定的命令并收集性能数据。
**逻辑分析:**
line_profiler输出了一张表格,其中包含以下信息:
* `Line #`:代码行的行号。
* `Hits`:代码行被执行的次数。
* `Time`:代码行执行总共花费的时间(单位:秒)。
* `Per Hit`:每个代码行执行的平均时间(单位:秒)。
* `% Time`:代码行执行时间占总执行时间的百分比。
* `Line Contents`:代码行的内容。
#### 2.1.3 memory_profiler
**简介:**
memory_profiler是一个第三方性能分析工具,用于分析内存使用情况。它通过在内存分配和释放时插入钩子来收集数据。
**使用方法:**
```python
import memory_profiler
def my_function():
# ...
if __name__ == "__main__":
memory_profiler.profile(my_function)
```
**输出:**
```
Line # Mem usage Increment Line Contents
4 17.9 MiB 0.0 MiB @profile
5 17.9 MiB 0.0 MiB """Example function for memory profiling"""
6 17.9 MiB 0.0 MiB x = 1
7 17.9 MiB 0.0 MiB y = 2
```
0
0
相关推荐
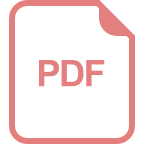
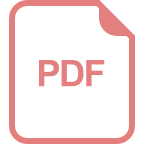
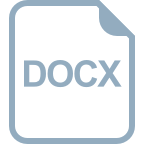





