Android获取GPS与基站经纬度实现教程
"这篇资源主要介绍了在Android平台上如何获取GPS和基站信息来得到经纬度地址。作者通过搜集网络上的资料,整理出一段实现这一功能的代码,并分享出来以供学习和参考。" 在Android开发中,获取设备的位置信息是常见的需求,这通常涉及到GPS和移动网络基站的数据。以下是一个简单的示例,展示了如何使用Android API来获取这些信息: 首先,我们需要在AndroidManifest.xml文件中添加必要的权限,允许应用访问位置服务: ```xml <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> ``` `ACCESS_FINE_LOCATION`允许应用访问GPS,而`ACCESS_COARSE_LOCATION`则允许使用网络定位。 接下来,在布局文件中,可以创建一个用于显示位置信息的界面,例如: ```xml <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#FFD3D7DF" android:orientation="vertical"> <!-- ...其他视图... --> <LinearLayout android:id="@+id/location" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginBottom="20dip" android:layout_marginLeft="20dip" android:layout_marginRight="20dip" android:layout_marginTop="20dip" android:background="@drawable/bg_frame" android:gravity="center_vertical" android:orientation="vertical" android:paddingBottom="2dip" android:paddingLeft="10dip" android:paddingRight="10dip" android:paddingTop="10dip"> <TextView android:id="@+id/providerTitle" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="获取经纬度:" android:textColor="#007979"/> <!-- ...其他视图用于显示经纬度... --> </LinearLayout> <!-- ...其他视图... --> </LinearLayout> ``` 在对应的Activity或Fragment中,我们需要实例化LocationManager并请求位置更新: ```java LocationManager locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE); locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, new LocationListener() { @Override public void onLocationChanged(Location location) { if (location != null) { // 更新UI,显示经纬度 TextView textView = findViewById(R.id.latitudeTextView); textView.setText("经度: " + location.getLongitude()); TextView textView2 = findViewById(R.id.longitudeTextView); textView2.setText("纬度: " + location.getLongitude()); } } // ...其他LocationListener方法... }); ``` 这里,我们使用了`LocationManager.GPS_PROVIDER`来获取GPS位置,如果需要同时考虑网络定位,可以使用`LocationManager.NETWORK_PROVIDER`。`requestLocationUpdates`方法用于注册监听器,参数分别是位置提供者、最小更新时间(毫秒)、最小更新距离(米)以及LocationListener对象。 此外,由于Android 6.0及以上版本需要在运行时动态申请权限,所以在应用启动时,还需要检查并请求`ACCESS_FINE_LOCATION`和`ACCESS_COARSE_LOCATION`权限: ```java if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) { ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION, Manifest.permission.ACCESS_COARSE_LOCATION}, MY_PERMISSIONS_REQUEST_LOCATION); } ``` 在处理权限请求结果时,记得检查用户是否授权,如果已授权,再执行上述的定位操作。 以上代码仅为示例,实际应用中可能需要更复杂的逻辑,比如处理位置数据的变化、优化性能、考虑用户隐私等。但这个基础框架可以帮助开发者理解如何在Android上获取和使用GPS及基站的经纬度地址。
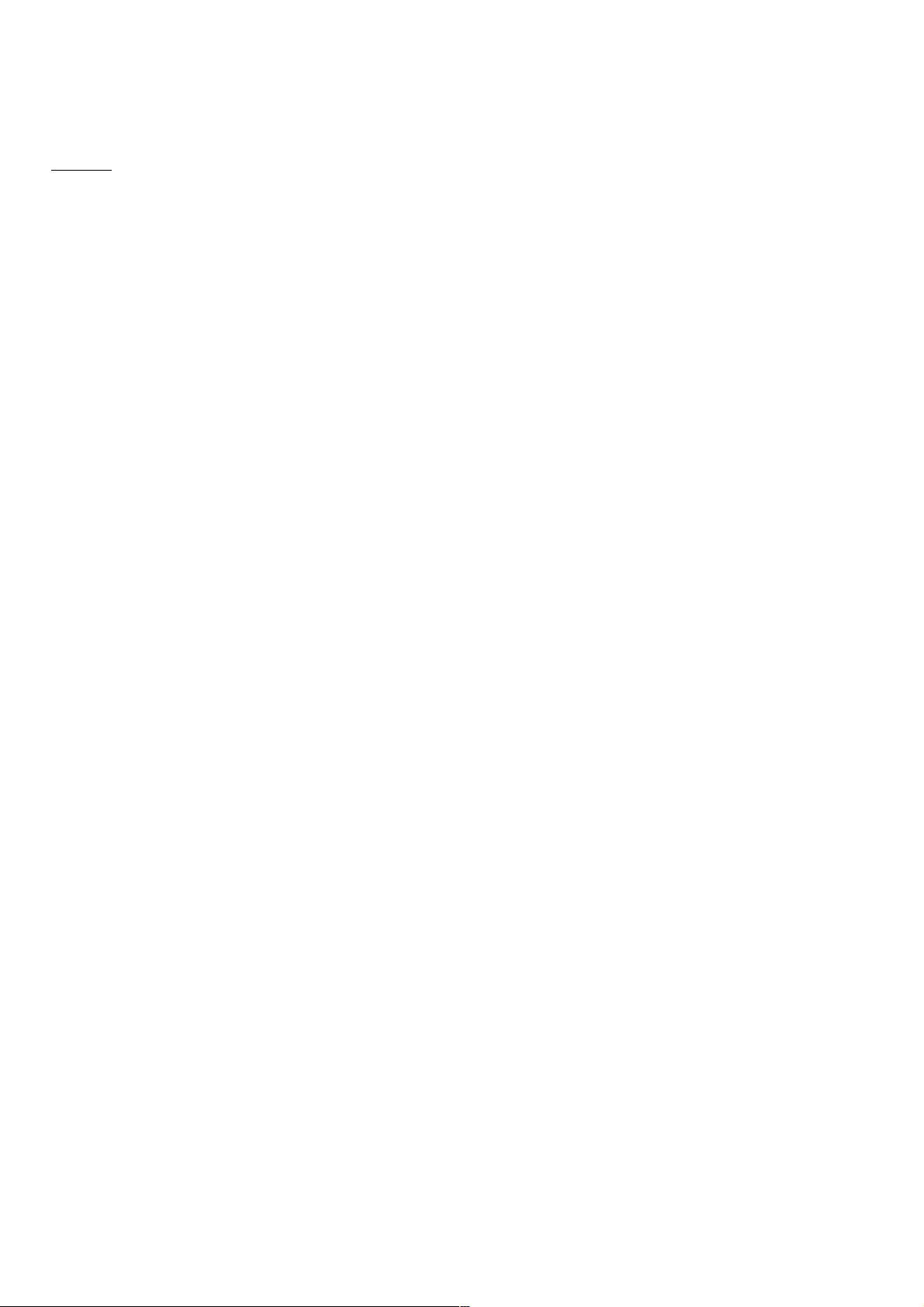
下载后可阅读完整内容,剩余3页未读,立即下载
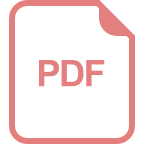
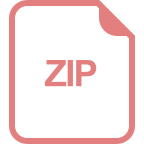

















- 粉丝: 6
- 资源: 897
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- OptiX传输试题与SDH基础知识
- C++Builder函数详解与应用
- Linux shell (bash) 文件与字符串比较运算符详解
- Adam Gawne-Cain解读英文版WKT格式与常见投影标准
- dos命令详解:基础操作与网络测试必备
- Windows 蓝屏代码解析与处理指南
- PSoC CY8C24533在电动自行车控制器设计中的应用
- PHP整合FCKeditor网页编辑器教程
- Java Swing计算器源码示例:初学者入门教程
- Eclipse平台上的可视化开发:使用VEP与SWT
- 软件工程CASE工具实践指南
- AIX LVM详解:网络存储架构与管理
- 递归算法解析:文件系统、XML与树图
- 使用Struts2与MySQL构建Web登录验证教程
- PHP5 CLI模式:用PHP编写Shell脚本教程
- MyBatis与Spring完美整合:1.0.0-RC3详解

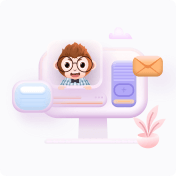
