没有合适的资源?快使用搜索试试~ 我知道了~
首页C++ STL设计:构建高效组件与实践指南
C++ STL设计:构建高效组件与实践指南

"《使用C++ STL设计组件》是一本权威且及时的指南,它全面介绍了C++标准模板库(STL)在软件开发中的应用和扩展。本书由Ulrich Breymann撰写,针对C++程序员提供了深入而实用的教程。作者以其丰富的经验和对STL内部机制的深入了解,展示了如何利用STL来构建高效、可靠的数据结构、算法和抽象数据类型。 该书的主要目标包括:首先,引导读者了解STL的基本概念;其次,展示如何充分利用这个强大的工具,提高软件质量与开发效率;最后,通过实例演示如何将STL应用到新组件的设计中,从而创建更为复杂和功能强大的解决方案。修订版完全符合最新的ISO/IEC C++标准,并配备了一个包含最新示例的官方网站,便于读者下载学习。 电子版只提供PDF格式,是对版权页中提到的原书进行了改进和更新。书中强调了遵循ISO/ANSI标准的重要性,确保代码的可移植性、维护性和复用性。有趣的是,STL正是在1994年夏季的Waterloo会议上被接纳为ISO/ANSI C++标准的一部分,自此开始了其在编程领域的广泛运用和成功。 书中涵盖了STL的各种容器(如vector、list、map等)、迭代器、算法以及模板的设计和使用技巧,帮助读者提升C++编程技能,使他们的软件开发工作更具效率和可靠性。无论你是初学者还是经验丰富的开发者,这本书都是构建现代C++应用程序的强大工具,值得每个认真对待C++的开发者珍藏在自己的图书馆中。"
资源推荐
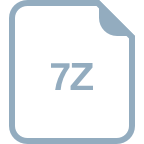


















给思维做按摩
- 粉丝: 5
- 资源: 55
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 解决Eclipse配置与导入Java工程常见问题
- 真空发生器:工作原理与抽吸性能分析
- 爱立信RBS6201开站流程详解
- 电脑开机声音解析:故障诊断指南
- JAVA实现贪吃蛇游戏
- 模糊神经网络实现与自学习能力探索
- PID型模糊神经网络控制器设计与学习算法
- 模糊神经网络在自适应PID控制器中的应用
- C++实现的学生成绩管理系统设计
- 802.1D STP 实现与优化:二层交换机中的生成树协议
- 解决Windows无法完成SD卡格式化的九种方法
- 软件测试方法:Beta与Alpha测试详解
- 软件测试周期详解:从需求分析到维护测试
- CMMI模型详解:软件企业能力提升的关键
- 移动Web开发框架选择:jQueryMobile、jQTouch、SenchaTouch对比
- Java程序设计试题与复习指南
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


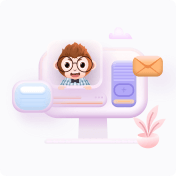
安全验证
文档复制为VIP权益,开通VIP直接复制
