没有合适的资源?快使用搜索试试~ 我知道了~
首页Learning JavaScript Design Patterns V1.7.0.pdf
Learning JavaScript Design Patterns V1.7.0.pdf
需积分: 9 8 下载量 53 浏览量
更新于2023-05-24
评论
收藏 2.26MB PDF 举报
Learning JavaScript Design Patterns V1.7.0. pdf 这是一本通过Javascript语言来阐述设计模式的书籍。 前面讲解的Javascript特性以及面向对象思想还是很有意思的。 由于Javascrip中的设计模式大都源自Java,所以作为设计模式入门材料还是不错的
资源详情
资源评论
资源推荐
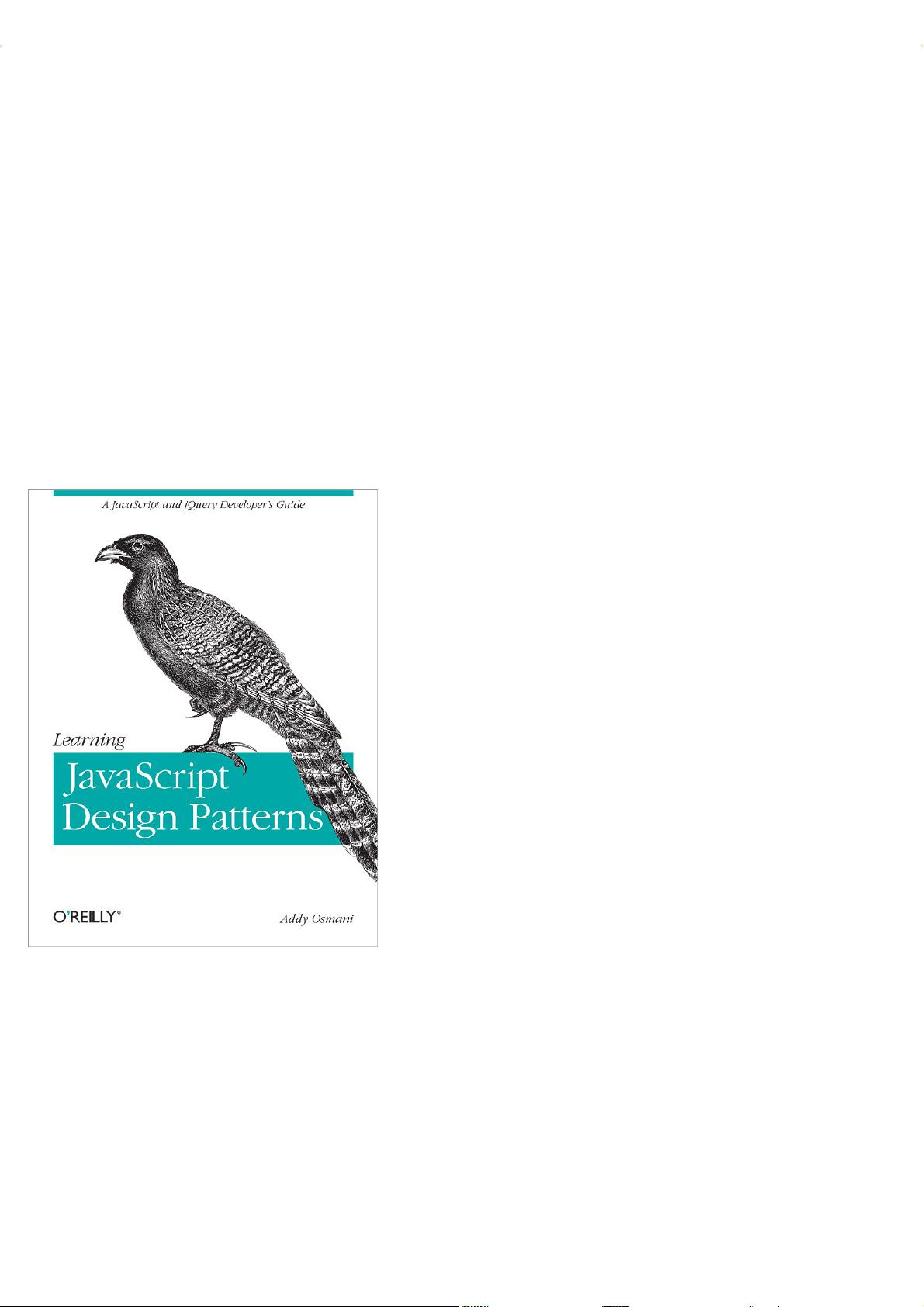
2018/6/1 Learning JavaScript Design Patterns
https://addyosmani.com/resources/essentialjsdesignpatterns/book/ 1/202
Learning JavaScript Design Paerns
A book by Addy Osmani
Volume 1.7.0
Copyright © Addy Osmani 2017.
Learning JavaScript Design Paerns is released under a Creative Commons Aribution-Noncommercial-No
Derivative Works 3.0 unported license. It is available for purchase via O'Reilly Media but will remain
available for both free online and as a physical (or eBook) purchase for readers wishing to support the
project.
Preface
Design paerns are reusable solutions to commonly occurring problems in software design. They are
both exciting and a fascinating topic to explore in any programming language.
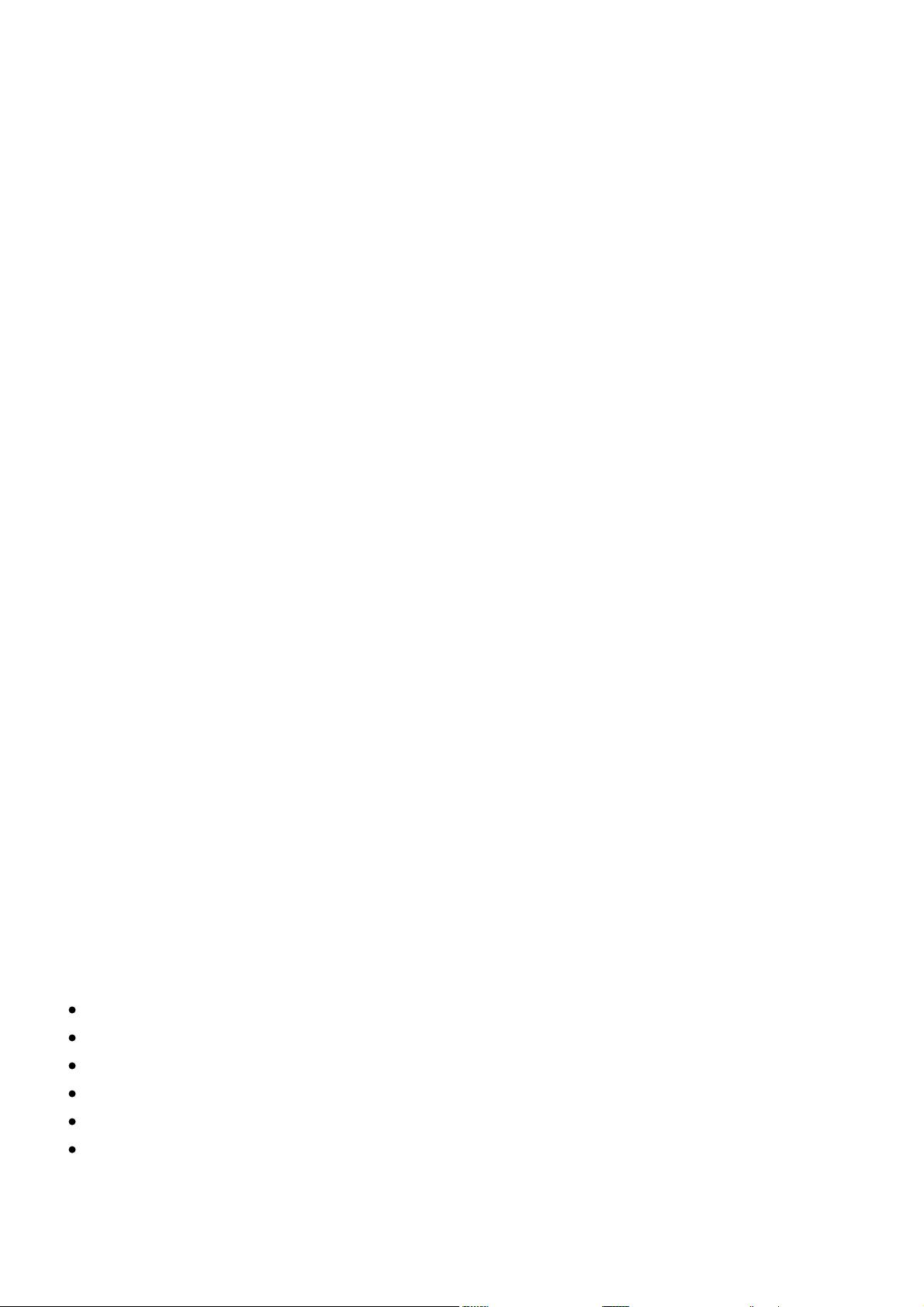
2018/6/1 Learning JavaScript Design Patterns
https://addyosmani.com/resources/essentialjsdesignpatterns/book/ 2/202
One reason for this is that they help us build upon the combined experience of many developers that
came before us and ensure we structure our code in an optimized way, meeting the needs of problems
we're aempting to solve.
Design paerns also provide us a common vocabulary to describe solutions. This can be significantly
simpler than describing syntax and semantics when we're aempting to convey a way of structuring a
solution in code form to others.
In this book we will explore applying both classical and modern design paerns to the JavaScript
programming language.
Target Audience
This book is targeted at professional developers wishing to improve their knowledge of design paerns
and how they can be applied to the JavaScript programming language.
Some of the concepts covered (closures, prototypal inheritance) will assume a level of basic prior
knowledge and understanding. If you find yourself needing to read further about these topics, a list of
suggested titles is provided for convenience.
If you would like to learn how to write beautiful, structured and organized code, I believe this is the
book for you.
Acknowledgments
I will always be grateful for the talented technical reviewers who helped review and improve this book,
including those from the community at large. The knowledge and enthusiasm they brought to the project
was simply amazing. The official technical reviewers tweets and blogs are also a regular source of both
ideas and inspiration and I wholeheartedly recommend checking them out.
Nicholas Zakas (hp://nczonline.net, @slicknet)
Andrée Hansson (hp://andreehansson.se, @peolanha)
Luke Smith (hp://lucassmith.name, @ls_n)
Eric Ferraiuolo (hp://ericf.me/, @ericf)
Peter Michaux (hp://michaux.ca, @petermichaux)
Alex Sexton (hp://alexsexton.com, @slexaxton)
I would also like to thank Rebecca Murphey (hp://rmurphey.com, @rmurphey) for providing the
inspiration to write this book and more importantly, continue to make it both available on GitHub and
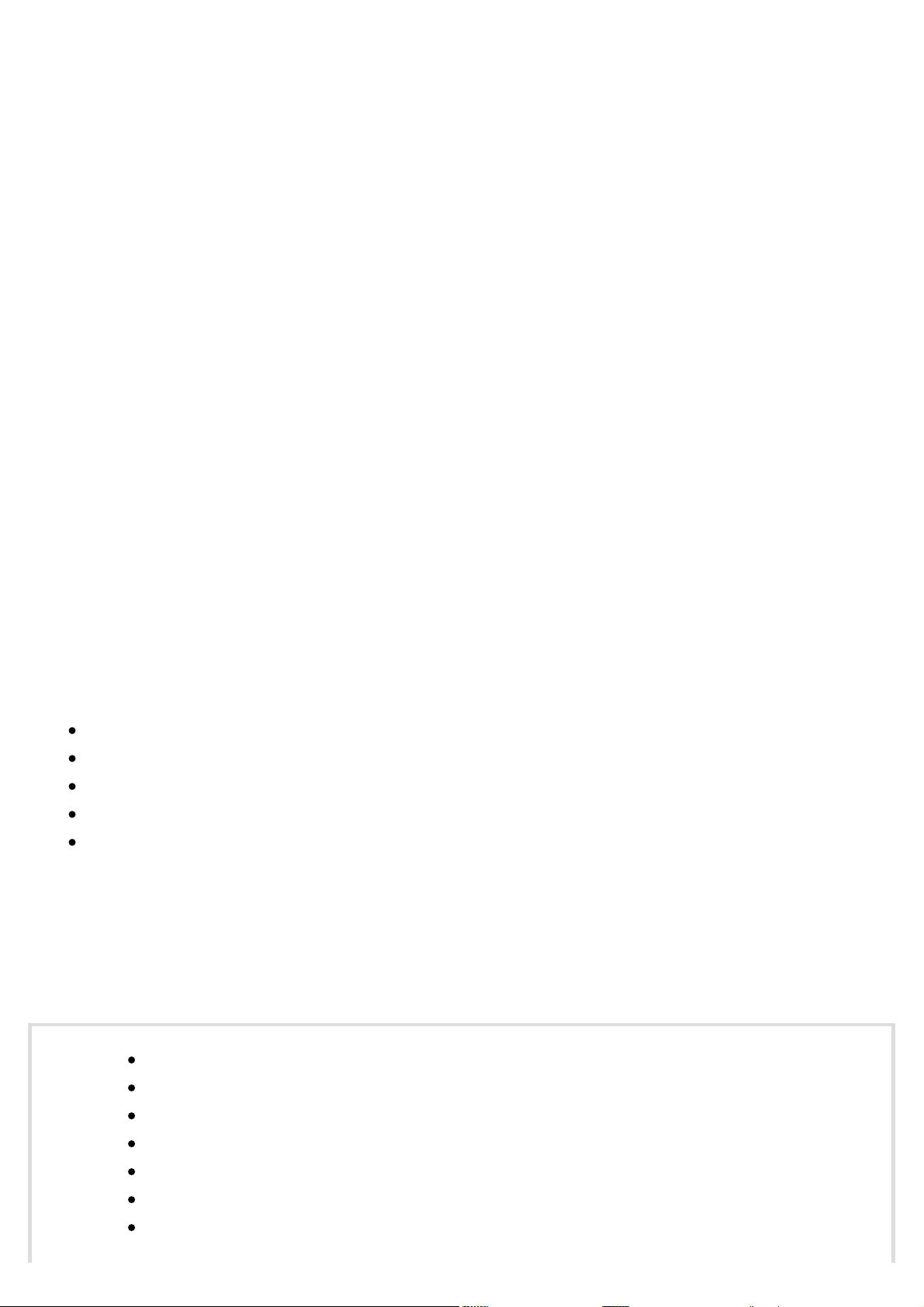
2018/6/1 Learning JavaScript Design Patterns
https://addyosmani.com/resources/essentialjsdesignpatterns/book/ 3/202
via O'Reilly.
Finally, I would like to thank my wonderful wife Ellie, for all of her support while I was puing together
this publication.
Credits
Whilst some of the paerns covered in this book were implemented based on personal experience, many
of them have been previously identified by the JavaScript community. This work is as such the
production of the combined experience of a number of developers. Similar to Stoyan Stefanov's logical
approach to preventing interruption of the narrative with credits (in JavaScript Paerns), I have listed
credits and suggested reading for any content covered in the references section.
If any articles or links have been missed in the list of references, please accept my heartfelt apologies. If
you contact me I'll be sure to update them to include you on the list.
Reading
Whilst this book is targeted at both beginners and intermediate developers, a basic understanding of
JavaScript fundamentals is assumed. Should you wish to learn more about the language, I am happy to
recommend the following titles:
JavaScript: The Definitive Guide by David Flanagan
Eloquent JavaScript by Marijn Haverbeke
JavaScript Paerns by Stoyan Stefanov
Writing Maintainable JavaScript by Nicholas Zakas
JavaScript: The Good Parts by Douglas Crockford
Table Of Contents
Introduction
What is a Paern?
"Paern"-ity Testing, Proto-Paerns & The Rule Of Three
The Structure Of A Design Paern
Writing Design Paerns
Anti-Paerns
Categories Of Design Paern
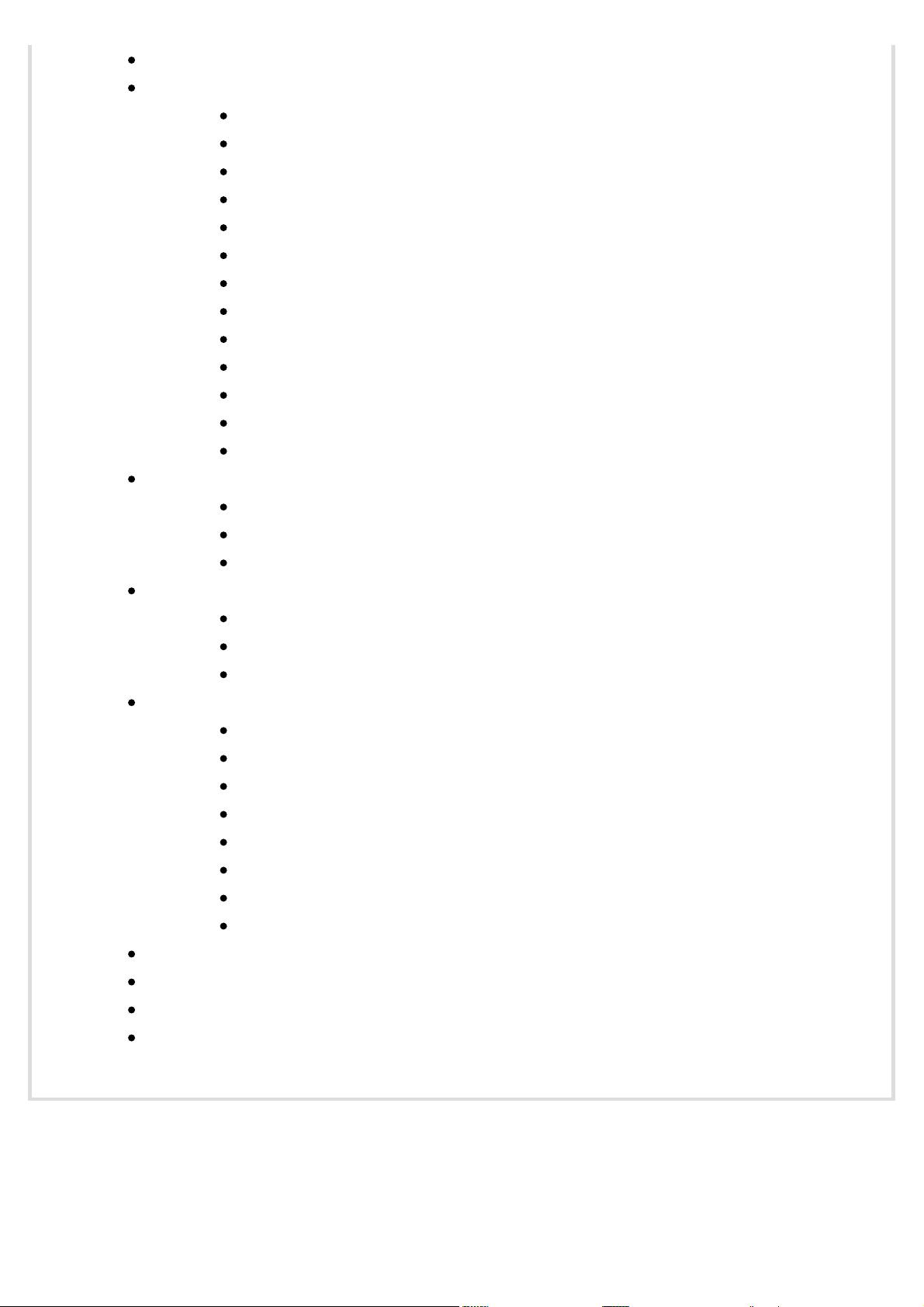
2018/6/1 Learning JavaScript Design Patterns
https://addyosmani.com/resources/essentialjsdesignpatterns/book/ 4/202
Summary Table Of Design Paern Categorization
JavaScript Design Paerns
Constructor Paern
Module Paern
Revealing Module Paern
Singleton Paern
Observer Paern
Mediator Paern
Prototype Paern
Command Paern
Facade Paern
Factory Paern
Mixin Paern
Decorator Paern
Flyweight Paern
JavaScript MV* Paerns
MVC Paern
MVP Paern
MVVM Paern
Modern Modular JavaScript Design Paerns
AMD
CommonJS
ES Harmony
Design Paerns In jQuery
Composite Paern
Adapter Paern
Facade Paern
Observer Paern
Iterator Paern
Lazy Initialization Paern
Proxy Paern
Builder Paern
jQuery Plugin Design Paerns
JavaScript Namespacing Paerns
Conclusions
References
Introduction
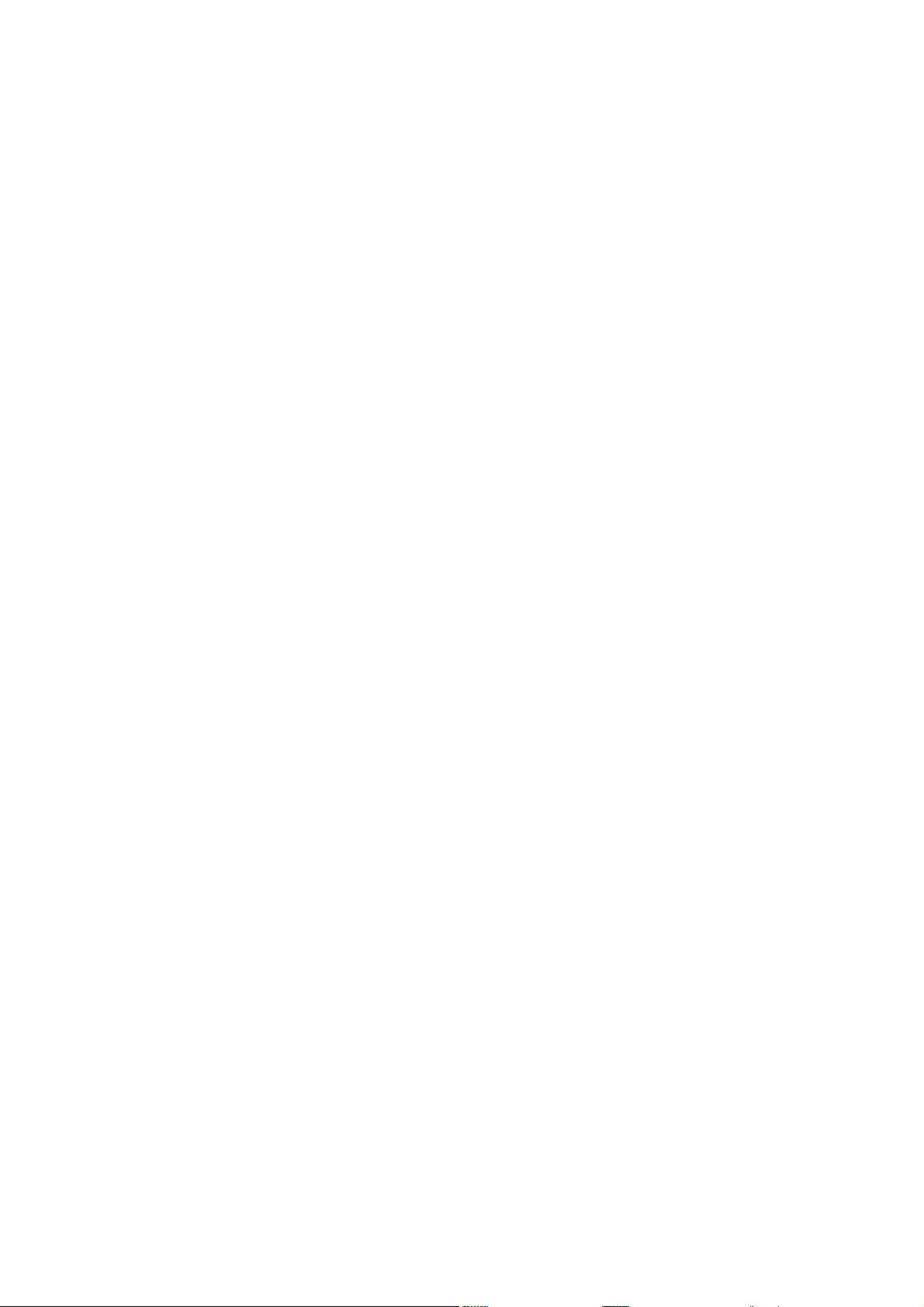
2018/6/1 Learning JavaScript Design Patterns
https://addyosmani.com/resources/essentialjsdesignpatterns/book/ 5/202
One of the most important aspects of writing maintainable code is being able to notice the recurring
themes in that code and optimize them. This is an area where knowledge of design paerns can prove
invaluable.
In the first part of this book, we will explore the history and importance of design paerns which can
really be applied to any programming language. If you're already sold on or are familiar with this
history, feel free to skip to the chapter "What is a Paern?" to continue reading.
Design paerns can be traced back to the early work of an architect named Christopher Alexander. He
would often write publications about his experience in solving design issues and how they related to
buildings and towns. One day, it occurred to Alexander that when used time and time again, certain
design constructs lead to a desired optimal effect.
In collaboration with Sara Ishikawa and Murray Silverstein, Alexander produced a paern language that
would help empower anyone wishing to design and build at any scale. This was published back in 1977
in a paper titled "A Paern Language", which was later released as a complete hardcover book.
Some 30 years ago, software engineers began to incorporate the principles Alexander had wrien about
into the first documentation about design paerns, which was to be a guide for novice developers
looking to improve their coding skills. It's important to note that the concepts behind design paerns
have actually been around in the programming industry since its inception, albeit in a less formalized
form.
One of the first and arguably most iconic formal works published on design paerns in software
engineering was a book in 1995 called Design Paerns: Elements Of Reusable Object-Oriented Software. This
was wrien by Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides - a group that became
known as the Gang of Four (or GoF for short).
The GoF's publication is considered quite instrumental to pushing the concept of design paerns further
in our field as it describes a number of development techniques and pitfalls as well as providing twenty-
three core Object-Oriented design paerns frequently used around the world today. We will be covering
these paerns in more detail in the section "Categories of Design Paerns".
In this book, we will take a look at a number of popular JavaScript design paerns and explore why
certain paerns may be more suitable for your projects than others. Remember that paerns can be
applied not just to vanilla JavaScript (i.e standard JavaScript code), but also to abstracted libraries such as
jQuery or dojo as well. Before we begin, let’s look at the exact definition of a "paern" in software design.
剩余201页未读,继续阅读
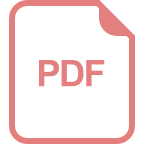
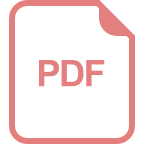


















haiyang5210
- 粉丝: 3
- 资源: 2
上传资源 快速赚钱
我的内容管理 收起
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

会员权益专享
最新资源
- RTL8188FU-Linux-v5.7.4.2-36687.20200602.tar(20765).gz
- c++校园超市商品信息管理系统课程设计说明书(含源代码) (2).pdf
- 建筑供配电系统相关课件.pptx
- 企业管理规章制度及管理模式.doc
- vb打开摄像头.doc
- 云计算-可信计算中认证协议改进方案.pdf
- [详细完整版]单片机编程4.ppt
- c语言常用算法.pdf
- c++经典程序代码大全.pdf
- 单片机数字时钟资料.doc
- 11项目管理前沿1.0.pptx
- 基于ssm的“魅力”繁峙宣传网站的设计与实现论文.doc
- 智慧交通综合解决方案.pptx
- 建筑防潮设计-PowerPointPresentati.pptx
- SPC统计过程控制程序.pptx
- SPC统计方法基础知识.pptx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


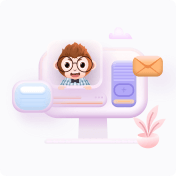
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0