没有合适的资源?快使用搜索试试~ 我知道了~
首页Linux与UNIX系统编程手册
Linux与UNIX系统编程手册

"《Linux编程接口》是一本深入讲解Linux和UNIX系统编程的手册,作者是Michael Kerrisk。这本书在行业内获得了高度评价,被赞誉为Linux编程领域的重要参考资料。"
《Linux编程接口》这本书详细阐述了Linux及UNIX系统编程的核心概念和实践技巧,是开发者必备的参考书籍之一。书中涵盖了各种低级编程API的细节和微妙之处,无论读者的水平如何,都能从中学习到有价值的知识。
书中的内容包括但不限于:
1. 进程管理:讨论了进程的创建、终止、信号处理、进程间通信(IPC)以及线程的概念与实现。
2. 文件系统接口:详述了打开、关闭、读写文件等基本操作,以及更复杂的文件控制和磁盘I/O操作。
3. 系统调用与库函数:解释了如socket编程、网络协议、套接字选项和并发服务等网络编程相关内容。
4. 内存管理:涵盖了内存分配、释放、映射和共享内存等主题,对Linux虚拟内存管理有深入探讨。
5. 错误处理和调试:指导如何有效地处理和报告程序错误,以及使用调试工具进行问题定位。
6. 标准I/O库:详细解析了标准输入/输出流,缓冲和文件描述符的使用。
7. 权限和安全:讲解了用户和组权限、访问控制列表(ACL)、文件系统安全以及capabilities机制。
8. 时间和定时器:涵盖时间处理函数,定时器,以及实时性编程。
9. 符号链接和硬链接:解释了这两种链接的区别和用法。
10. 符号表和动态链接:探讨了程序加载、动态链接库以及符号解析过程。
本书的作者Michael Kerrisk以其对Linux编程的深入理解和对技术的严谨态度,不仅提供了丰富的实践示例,还积极参与Linux内核的改进工作,确保书中介绍的知识与最新的Linux版本保持同步。他的贡献使得Linux编程变得更加易懂和高效,无论是新手还是经验丰富的开发者,都能从中受益。
《Linux编程接口》是一部全面而权威的Linux系统编程指南,对于想要深入了解Linux内核机制和提升编程技能的人来说,这是一本不可或缺的书籍。通过阅读本书,读者将能够更好地理解并利用Linux的底层功能,从而编写出更高效、更可靠的程序。
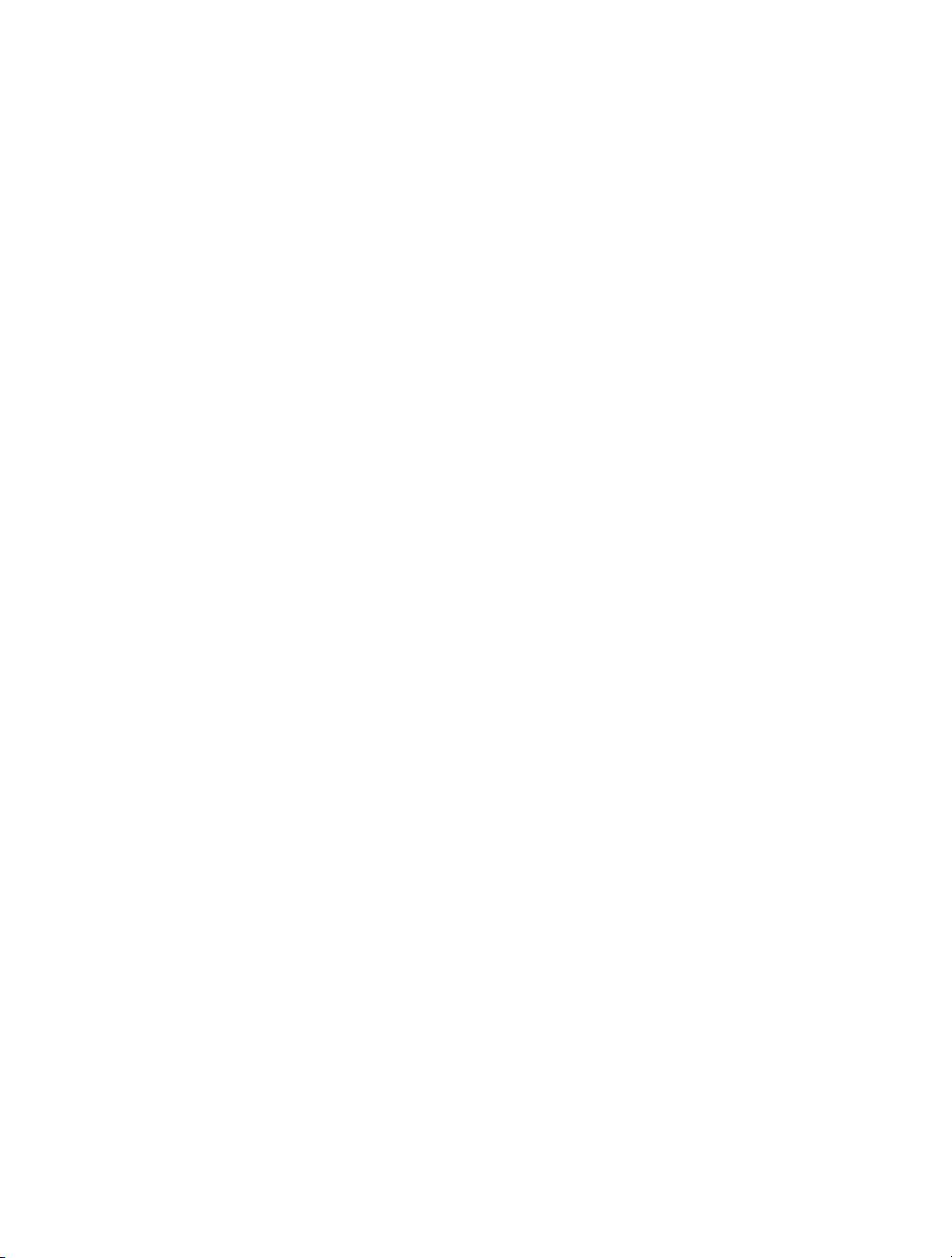
CONTENTS IN DETAIL
PREFACE xxxi
1 HISTORY AND STANDARDS 1
1.1 A Brief History of UNIX and C ........................................................................................2
1.2 A Brief History of Linux ...................................................................................................5
1.2.1 The GNU Project ......................................................................................5
1.2.2 The Linux Kernel .......................................................................................6
1.3 Standardization .......................................................................................................... 10
1.3.1 The C Programming Language ................................................................. 10
1.3.2 The First POSIX Standards........................................................................ 11
1.3.3 X/Open Company and The Open Group .................................................. 13
1.3.4 SUSv3 and POSIX.1-2001....................................................................... 13
1.3.5 SUSv4 and POSIX.1-2008....................................................................... 15
1.3.6 UNIX Standards Timeline ......................................................................... 16
1.3.7 Implementation Standards........................................................................ 17
1.3.8 Linux, Standards, and the Linux Standard Base........................................... 18
1.4 Summary.................................................................................................................... 19
2 FUNDAMENTAL CONCEPTS 21
2.1 The Core Operating System: The Kernel......................................................................... 21
2.2 The Shell .................................................................................................................... 24
2.3 Users and Groups ....................................................................................................... 26
2.4 Single Directory Hierarchy, Directories, Links, and Files ................................................... 27
2.5 File I/O Model ........................................................................................................... 29
2.6 Programs.................................................................................................................... 30
2.7 Processes ................................................................................................................... 31
2.8 Memory Mappings...................................................................................................... 35
2.9 Static and Shared Libraries........................................................................................... 35
2.10 Interprocess Communication and Synchronization ........................................................... 36
2.11 Signals....................................................................................................................... 37
2.12 Threads...................................................................................................................... 38
2.13 Process Groups and Shell Job Control............................................................................ 38
2.14 Sessions, Controlling Terminals, and Controlling Processes............................................... 39
2.15 Pseudoterminals .......................................................................................................... 39
2.16 Date and Time ............................................................................................................ 40
2.17 Client-Server Architecture ............................................................................................. 40
2.18 Realtime..................................................................................................................... 41
2.19 The
/proc File System................................................................................................... 42
2.20 Summary.................................................................................................................... 42
3 SYSTEM PROGRAMMING CONCEPTS 43
3.1 System Calls ............................................................................................................... 43
3.2 Library Functions ......................................................................................................... 46
3.3 The Standard C Library; The GNU C Library (
glibc) ......................................................... 47
3.4 Handling Errors from System Calls and Library Functions.................................................. 48
3.5 Notes on the Example Programs in This Book.................................................................. 50
3.5.1 Command-Line Options and Arguments ..................................................... 50
3.5.2 Common Functions and Header Files......................................................... 51
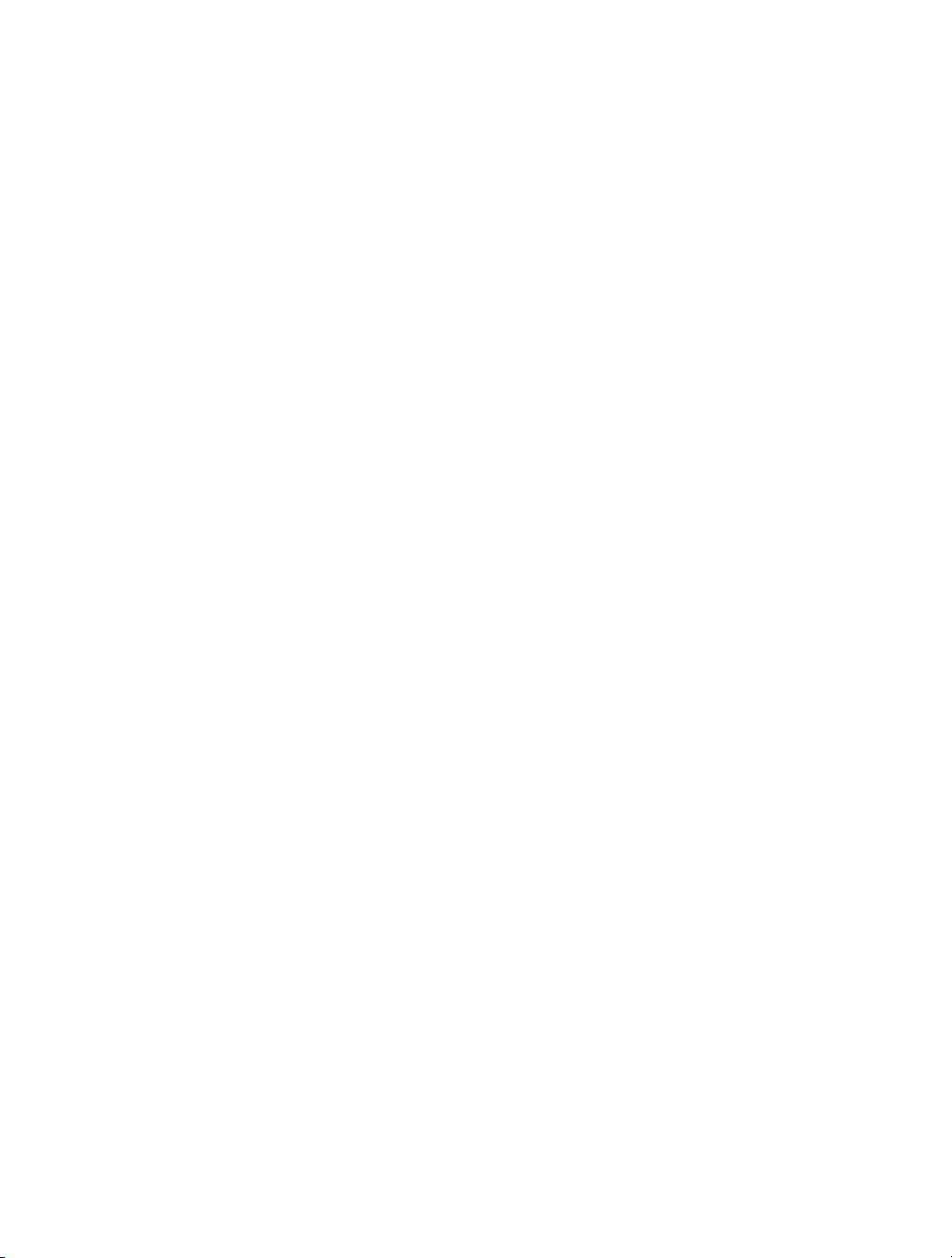
xii Contents in Detail
3.6 Portability Issues.......................................................................................................... 61
3.6.1 Feature Test Macros ................................................................................ 61
3.6.2 System Data Types.................................................................................. 63
3.6.3 Miscellaneous Portability Issues................................................................. 66
3.7 Summary.................................................................................................................... 68
3.8 Exercise ..................................................................................................................... 68
4 FILE I/O: THE UNIVERSAL I/O MODEL 69
4.1 Overview ................................................................................................................... 69
4.2 Universality of I/O ...................................................................................................... 72
4.3 Opening a File:
open()................................................................................................. 72
4.3.1 The
open() flags Argument........................................................................ 74
4.3.2 Errors from
open() ................................................................................... 77
4.3.3 The
creat() System Call ............................................................................ 78
4.4 Reading from a File:
read()........................................................................................... 79
4.5 Writing to a File:
write()............................................................................................... 80
4.6 Closing a File:
close() ................................................................................................... 80
4.7 Changing the File Offset:
lseek().................................................................................... 81
4.8 Operations Outside the Universal I/O Model:
ioctl() ....................................................... 86
4.9 Summary.................................................................................................................... 86
4.10 Exercises .................................................................................................................... 87
5 FILE I/O: FURTHER DETAILS 89
5.1 Atomicity and Race Conditions ..................................................................................... 90
5.2 File Control Operations:
fcntl() ..................................................................................... 92
5.3 Open File Status Flags ................................................................................................. 93
5.4 Relationship Between File Descriptors and Open Files ...................................................... 94
5.5 Duplicating File Descriptors .......................................................................................... 96
5.6 File I/O at a Specified Offset:
pread() and pwrite() ......................................................... 98
5.7 Scatter-Gather I/O:
readv() and writev() ........................................................................ 99
5.8 Truncating a File:
truncate() and ftruncate() ................................................................. 103
5.9 Nonblocking I/O ...................................................................................................... 103
5.10 I/O on Large Files..................................................................................................... 104
5.11 The
/dev/fd Directory ................................................................................................ 107
5.12 Creating Temporary Files ........................................................................................... 108
5.13 Summary.................................................................................................................. 109
5.14 Exercises .................................................................................................................. 110
6 PROCESSES 113
6.1 Processes and Programs............................................................................................. 113
6.2 Process ID and Parent Process ID................................................................................. 114
6.3 Memory Layout of a Process ....................................................................................... 115
6.4 Virtual Memory Management ..................................................................................... 118
6.5 The Stack and Stack Frames ....................................................................................... 121
6.6 Command-Line Arguments (
argc, argv) ......................................................................... 122
6.7 Environment List ........................................................................................................ 125
6.8 Performing a Nonlocal Goto:
setjmp() and longjmp() .................................................... 131
6.9 Summary.................................................................................................................. 138
6.10 Exercises .................................................................................................................. 138
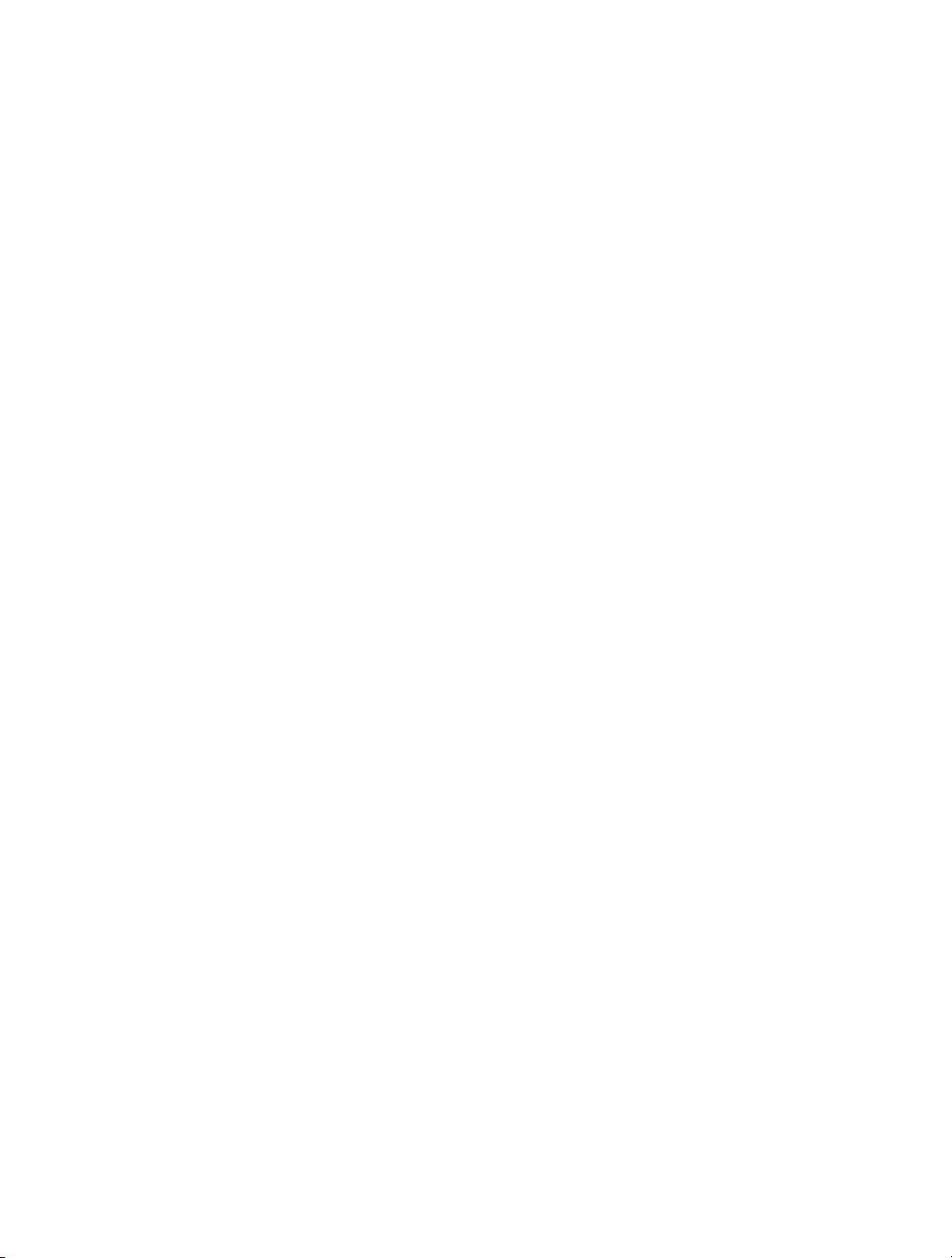
Contents in Detail xiii
7 MEMORY ALLOCATION 139
7.1 Allocating Memory on the Heap ................................................................................. 139
7.1.1 Adjusting the Program Break:
brk() and sbrk() .......................................... 139
7.1.2 Allocating Memory on the Heap:
malloc() and free() ................................. 140
7.1.3 Implementation of
malloc() and free() ...................................................... 144
7.1.4 Other Methods of Allocating Memory on the Heap ................................... 147
7.2 Allocating Memory on the Stack:
alloca() ..................................................................... 150
7.3 Summary.................................................................................................................. 151
7.4 Exercises .................................................................................................................. 152
8USERS AND GROUPS 153
8.1 The Password File: /etc/passwd ................................................................................... 153
8.2 The Shadow Password File:
/etc/shadow ...................................................................... 155
8.3 The Group File:
/etc/group......................................................................................... 155
8.4 Retrieving User and Group Information ........................................................................ 157
8.5 Password Encryption and User Authentication............................................................... 162
8.6 Summary.................................................................................................................. 166
8.7 Exercises .................................................................................................................. 166
9 PROCESS CREDENTIALS 167
9.1 Real User ID and Real Group ID.................................................................................. 167
9.2 Effective User ID and Effective Group ID....................................................................... 168
9.3 Set-User-ID and Set-Group-ID Programs ........................................................................ 168
9.4 Saved Set-User-ID and Saved Set-Group-ID ................................................................... 170
9.5 File-System User ID and File-System Group ID................................................................ 171
9.6 Supplementary Group IDs .......................................................................................... 172
9.7 Retrieving and Modifying Process Credentials............................................................... 172
9.7.1 Retrieving and Modifying Real, Effective, and Saved Set IDs ...................... 172
9.7.2 Retrieving and Modifying File-System IDs ................................................. 178
9.7.3 Retrieving and Modifying Supplementary Group IDs ................................. 178
9.7.4 Summary of Calls for Modifying Process Credentials ................................. 180
9.7.5 Example: Displaying Process Credentials ................................................. 182
9.8 Summary.................................................................................................................. 183
9.9 Exercises .................................................................................................................. 184
10 TIME 185
10.1 Calendar Time .......................................................................................................... 186
10.2 Time-Conversion Functions.......................................................................................... 187
10.2.1 Converting
time_t to Printable Form ........................................................ 188
10.2.2 Converting Between
time_t and Broken-Down Time................................... 189
10.2.3 Converting Between Broken-Down Time and Printable Form ....................... 191
10.3 Timezones ................................................................................................................ 197
10.4 Locales..................................................................................................................... 200
10.5 Updating the System Clock......................................................................................... 204
10.6 The Software Clock (Jiffies) ......................................................................................... 205
10.7 Process Time............................................................................................................. 206
10.8 Summary.................................................................................................................. 209
10.9 Exercise ................................................................................................................... 210
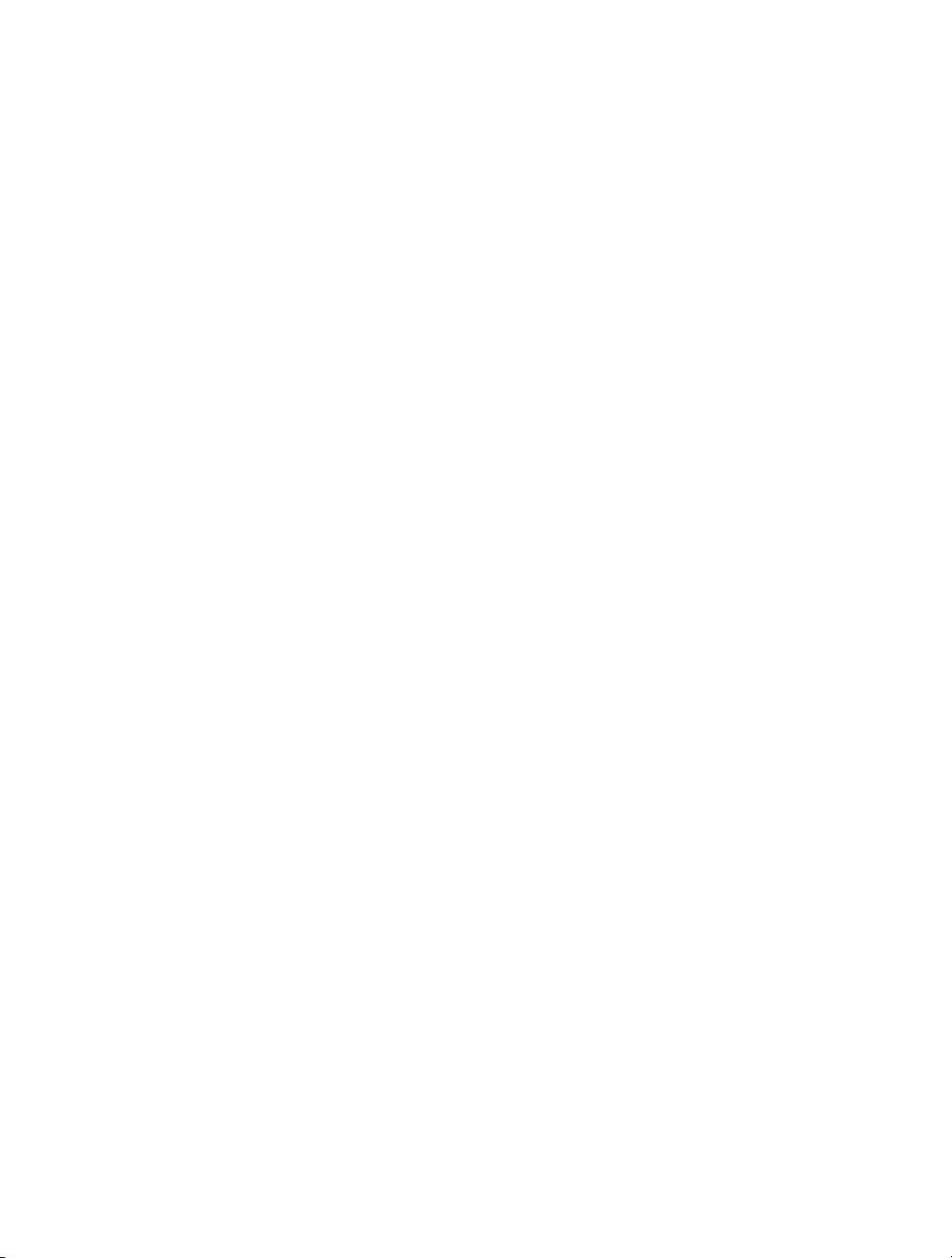
xiv Contents in Detail
11 SYSTEM LIMITS AND OPTIONS 211
11.1 System Limits............................................................................................................. 212
11.2 Retrieving System Limits (and Options) at Run Time ........................................................ 215
11.3 Retrieving File-Related Limits (and Options) at Run Time.................................................. 217
11.4 Indeterminate Limits ................................................................................................... 219
11.5 System Options......................................................................................................... 219
11.6 Summary.................................................................................................................. 221
11.7 Exercises .................................................................................................................. 222
12 SYSTEM AND PROCESS INFORMATION 223
12.1 The /proc File System................................................................................................. 223
12.1.1 Obtaining Information About a Process:
/proc/PID................................... 224
12.1.2 System Information Under
/proc.............................................................. 226
12.1.3 Accessing
/proc Files ............................................................................ 226
12.2 System Identification:
uname() .................................................................................... 229
12.3 Summary.................................................................................................................. 231
12.4 Exercises .................................................................................................................. 231
13 FILE I/O BUFFERING 233
13.1 Kernel Buffering of File I/O: The Buffer Cache .............................................................. 233
13.2 Buffering in the
stdio Library ....................................................................................... 237
13.3 Controlling Kernel Buffering of File I/O........................................................................ 239
13.4 Summary of I/O Buffering .......................................................................................... 243
13.5 Advising the Kernel About I/O Patterns........................................................................ 244
13.6 Bypassing the Buffer Cache: Direct I/O........................................................................ 246
13.7 Mixing Library Functions and System Calls for File I/O .................................................. 248
13.8 Summary.................................................................................................................. 249
13.9 Exercises .................................................................................................................. 250
14 FILE SYSTEMS 251
14.1 Device Special Files (Devices) ..................................................................................... 252
14.2 Disks and Partitions ................................................................................................... 253
14.3 File Systems.............................................................................................................. 254
14.4 I-nodes..................................................................................................................... 256
14.5 The Virtual File System (VFS) ....................................................................................... 259
14.6 Journaling File Systems............................................................................................... 260
14.7 Single Directory Hierarchy and Mount Points ................................................................ 261
14.8 Mounting and Unmounting File Systems ....................................................................... 262
14.8.1 Mounting a File System:
mount() ............................................................ 264
14.8.2 Unmounting a File System:
umount() and umount2() ................................ 269
14.9 Advanced Mount Features.......................................................................................... 271
14.9.1 Mounting a File System at Multiple Mount Points....................................... 271
14.9.2 Stacking Multiple Mounts on the Same Mount Point................................... 271
14.9.3 Mount Flags That Are Per-Mount Options ................................................. 272
14.9.4 Bind Mounts......................................................................................... 272
14.9.5 Recursive Bind Mounts........................................................................... 273
14.10 A Virtual Memory File System:
tmpfs............................................................................ 274
14.11 Obtaining Information About a File System:
statvfs()...................................................... 276
14.12 Summary.................................................................................................................. 277
14.13 Exercise ................................................................................................................... 278
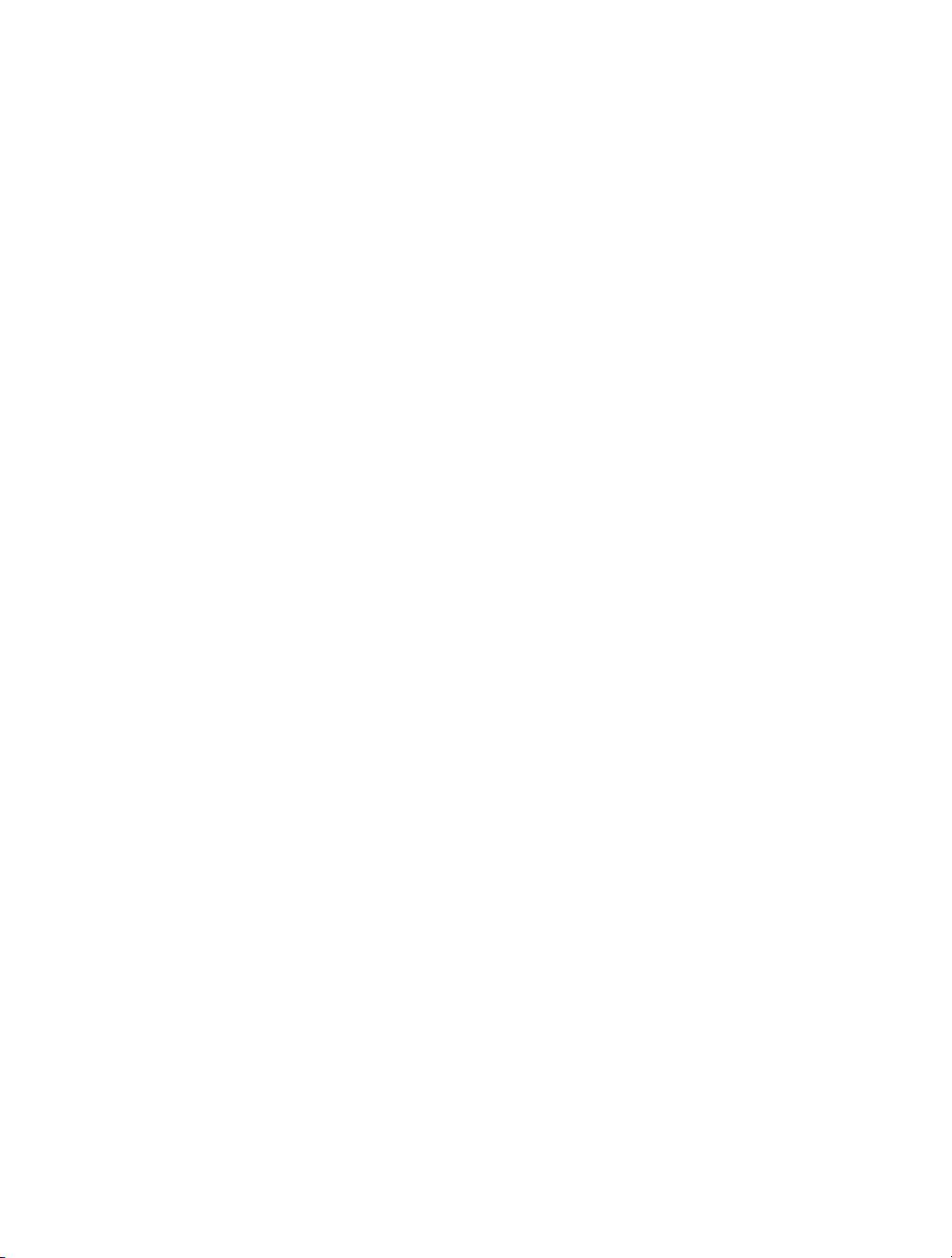
Contents in Detail xv
15 FILE ATTRIBUTES 279
15.1 Retrieving File Information: stat() ................................................................................. 279
15.2 File Timestamps......................................................................................................... 285
15.2.1 Changing File Timestamps with
utime() and utimes()................................. 287
15.2.2 Changing File Timestamps with
utimensat() and futimens() ........................ 289
15.3 File Ownership ......................................................................................................... 291
15.3.1 Ownership of New Files ........................................................................ 291
15.3.2 Changing File Ownership:
chown(), fchown(), and lchown()....................... 291
15.4 File Permissions......................................................................................................... 294
15.4.1 Permissions on Regular Files................................................................... 294
15.4.2 Permissions on Directories...................................................................... 297
15.4.3 Permission-Checking Algorithm ............................................................... 297
15.4.4 Checking File Accessibility:
access()......................................................... 299
15.4.5 Set-User-ID, Set-Group-ID, and Sticky Bits ................................................. 300
15.4.6 The Process File Mode Creation Mask:
umask()........................................ 301
15.4.7 Changing File Permissions:
chmod() and fchmod()..................................... 303
15.5 I-node Flags (
ext2 Extended File Attributes) ................................................................... 304
15.6 Summary.................................................................................................................. 308
15.7 Exercises .................................................................................................................. 309
16 EXTENDED ATTRIBUTES 311
16.1 Overview ................................................................................................................. 311
16.2 Extended Attribute Implementation Details .................................................................... 313
16.3 System Calls for Manipulating Extended Attributes......................................................... 314
16.4 Summary.................................................................................................................. 318
16.5 Exercise ................................................................................................................... 318
17 ACCESS CONTROL LISTS 319
17.1 Overview ................................................................................................................. 320
17.2 ACL Permission-Checking Algorithm............................................................................. 321
17.3 Long and Short Text Forms for ACLs............................................................................. 323
17.4 The
ACL_MASK Entry and the ACL Group Class................................................................ 324
17.5 The
getfacl and setfacl Commands ............................................................................... 325
17.6 Default ACLs and File Creation ................................................................................... 327
17.7 ACL Implementation Limits .......................................................................................... 328
17.8 The ACL API ............................................................................................................. 329
17.9 Summary.................................................................................................................. 337
17.10 Exercise ................................................................................................................... 337
18 DIRECTORIES AND LINKS 339
18.1 Directories and (Hard) Links........................................................................................ 339
18.2 Symbolic (Soft) Links .................................................................................................. 342
18.3 Creating and Removing (Hard) Links:
link() and unlink()............................................... 344
18.4 Changing the Name of a File:
rename() ....................................................................... 348
18.5 Working with Symbolic Links:
symlink() and readlink().................................................. 349
18.6 Creating and Removing Directories:
mkdir() and rmdir() ............................................... 350
18.7 Removing a File or Directory:
remove()......................................................................... 352
18.8 Reading Directories:
opendir() and readdir() ................................................................ 352
18.9 File Tree Walking:
nftw() ........................................................................................... 358
18.10 The Current Working Directory of a Process ................................................................. 363
18.11 Operating Relative to a Directory File Descriptor ........................................................... 365
18.12 Changing the Root Directory of a Process:
chroot() ........................................................ 367
18.13 Resolving a Pathname:
realpath() ................................................................................ 369
剩余1558页未读,继续阅读
2018-12-27 上传
2021-03-08 上传
2018-08-23 上传
2023-07-27 上传
2023-10-05 上传
2023-07-30 上传
2023-03-30 上传
2023-06-10 上传
2023-08-13 上传

csprimer
- 粉丝: 80
- 资源: 51
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- Angular实现MarcHayek简历展示应用教程
- Crossbow Spot最新更新 - 获取Chrome扩展新闻
- 量子管道网络优化与Python实现
- Debian系统中APT缓存维护工具的使用方法与实践
- Python模块AccessControl的Windows64位安装文件介绍
- 掌握最新*** Fisher资讯,使用Google Chrome扩展
- Ember应用程序开发流程与环境配置指南
- EZPCOpenSDK_v5.1.2_build***版本更新详情
- Postcode-Finder:利用JavaScript和Google Geocode API实现
- AWS商业交易监控器:航线行为分析与营销策略制定
- AccessControl-4.0b6压缩包详细使用教程
- Python编程实践与技巧汇总
- 使用Sikuli和Python打造颜色求解器项目
- .Net基础视频教程:掌握GDI绘图技术
- 深入理解数据结构与JavaScript实践项目
- 双子座在线裁判系统:提高编程竞赛效率
安全验证
文档复制为VIP权益,开通VIP直接复制
