python推箱子
时间: 2023-06-22 07:25:21 浏览: 102
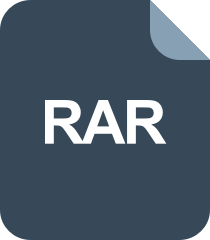
使用python语言制作的推箱子游戏,运行简单,可作毕业设计,下载即可运行

推箱子是一种常见的益智游戏,使用Python实现也是比较容易的。以下是一个简单的推箱子游戏实现:
1. 首先,我们需要定义地图和人物、箱子的位置,比如使用二维列表表示地图,用元组表示人物和箱子的位置:
```
map = [
['#', '#', '#', '#', '#', '#', '#', '#', '#', '#'],
['#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'],
['#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'],
['#', ' ', ' ', ' ', ' ', ' ', '#', '#', '#', '#'],
['#', ' ', ' ', ' ', ' ', ' ', '#', ' ', ' ', '#'],
['#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'],
['#', '#', '#', '#', '#', '#', '#', '#', '#', '#']
]
player_pos = (1, 1) # 人物初始位置
box_pos = [(3, 6), (4, 6)] # 箱子初始位置
```
2. 然后,我们需要定义游戏的操作,比如上下左右移动,判断是否胜利等:
```
def move(dx, dy):
global player_pos, box_pos
new_player_pos = (player_pos[0] + dx, player_pos[1] + dy)
if map[new_player_pos[0]][new_player_pos[1]] == '#':
return
if new_player_pos in box_pos:
new_box_pos = (new_player_pos[0] + dx, new_player_pos[1] + dy)
if map[new_box_pos[0]][new_box_pos[1]] == '#':
return
if new_box_pos in box_pos:
return
box_pos.remove(new_player_pos)
box_pos.append(new_box_pos)
player_pos = new_player_pos
def is_win():
for pos in box_pos:
if map[pos[0]][pos[1]] != 'X':
return False
return True
```
3. 最后,我们可以使用Pygame库来实现图形化界面,并在界面中处理游戏操作和状态变化:
```
import pygame
pygame.init()
# 设置窗口大小
screen_size = (800, 600)
screen = pygame.display.set_mode(screen_size)
pygame.display.set_caption('Push Box')
# 加载图片
wall_image = pygame.image.load('wall.png')
floor_image = pygame.image.load('floor.png')
player_image = pygame.image.load('player.png')
box_image = pygame.image.load('box.png')
win_image = pygame.image.load('win.png')
# 主循环
while True:
# 绘制地图
for y in range(len(map)):
for x in range(len(map[y])):
if map[y][x] == '#':
screen.blit(wall_image, (x * 50, y * 50))
else:
screen.blit(floor_image, (x * 50, y * 50))
if (x, y) == player_pos:
screen.blit(player_image, (x * 50, y * 50))
if (x, y) in box_pos:
screen.blit(box_image, (x * 50, y * 50))
# 检查事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
move(0, -1)
elif event.key == pygame.K_DOWN:
move(0, 1)
elif event.key == pygame.K_LEFT:
move(-1, 0)
elif event.key == pygame.K_RIGHT:
move(1, 0)
# 检查是否胜利
if is_win():
screen.blit(win_image, (0, 0))
# 更新界面
pygame.display.flip()
```
希望这个简单的例子可以帮助你了解如何使用Python实现推箱子游戏。
阅读全文
相关推荐


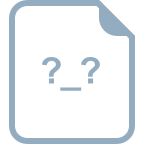











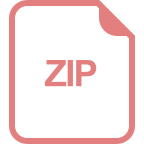
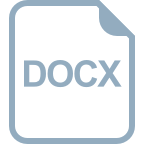