用结构体编写一程序,允许从键盘输入任意多个英语单词(单词可以重复),中间用空格分开,输入0表示输入结束。该程序可以统计同一个英语单词被输入几次,最后对英文单词按字典顺序输出,后面跟上该单词被输入的次数。
时间: 2023-05-19 20:04:16 浏览: 186
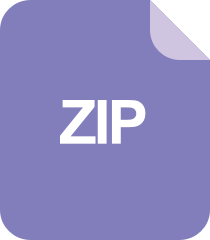
vc使用程序模拟键盘输入
以下是用 C 语言编写的程序,可以实现您的要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORDS 1000
#define MAX_WORD_LEN 20
struct word_count {
char word[MAX_WORD_LEN];
int count;
};
int cmp_word(const void *a, const void *b) {
return strcmp(((struct word_count *)a)->word, ((struct word_count *)b)->word);
}
int main() {
struct word_count words[MAX_WORDS];
int n = 0;
printf("请输入英语单词,以空格分隔,输入0表示结束:\n");
while (1) {
char word[MAX_WORD_LEN];
scanf("%s", word);
if (strcmp(word, "0") == 0) {
break;
}
int found = 0;
for (int i = 0; i < n; i++) {
if (strcmp(words[i].word, word) == 0) {
words[i].count++;
found = 1;
break;
}
}
if (!found) {
if (n >= MAX_WORDS) {
printf("输入的单词太多了,最多只能输入 %d 个单词\n", MAX_WORDS);
exit(1);
}
strcpy(words[n].word, word);
words[n].count = 1;
n++;
}
}
qsort(words, n, sizeof(struct word_count), cmp_word);
printf("按字典顺序输出单词及其出现次数:\n");
for (int i = 0; i < n; i++) {
printf("%s: %d\n", words[i].word, words[i].count);
}
return 0;
}
```
您可以将以上代码保存为一个名为 `word_count.c` 的文件,然后使用 C 语言编译器编译并运行它。例如,在 Linux 系统下,您可以使用以下命令编译:
```
gcc -o word_count word_count.c
```
然后运行:
```
./word_count
```
程序会提示您输入英语单词,您可以按照要求输入,然后按下回车键,程序会输出按字典顺序排序后的单词及其出现次数。
阅读全文
相关推荐
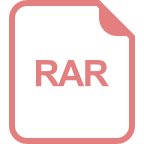
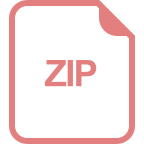








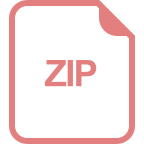
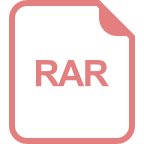
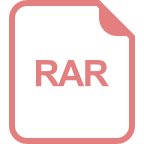
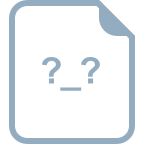
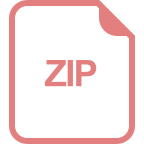
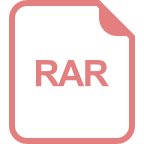
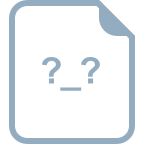
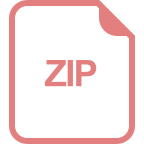