二叉树先序中序后序
时间: 2023-07-21 09:15:46 浏览: 110
二叉树有三种遍历方式:先序遍历、中序遍历和后序遍历。
先序遍历:先遍历根节点,然后遍历左子树,最后遍历右子树。
中序遍历:先遍历左子树,然后遍历根节点,最后遍历右子树。
后序遍历:先遍历左子树,然后遍历右子树,最后遍历根节点。
例如,对于下面这棵二叉树:
```
A
/ \
B C
/ \ \
D E F
```
其先序遍历结果为:A B D E C F
其中序遍历结果为:D B E A C F
其后序遍历结果为:D E B F C A
相关问题
二叉树先序中序后序递归遍历
二叉树的遍历方式有三种:前序遍历、中序遍历和后序遍历。递归是实现这三种遍历方式的常用方法。
1. 前序遍历:先访问根节点,再访问左子树,最后访问右子树。
```python
def preorderTraversal(root):
if not root:
return []
res = []
res.append(root.val)
res += self.preorderTraversal(root.left)
res += self.preorderTraversal(root.right)
return res
```
2. 中序遍历:先访问左子树,再访问根节点,最后访问右子树。
```python
def inorderTraversal(root):
if not root:
return []
res = []
res += self.inorderTraversal(root.left)
res.append(root.val)
res += self.inorderTraversal(root.right)
return res
```
3. 后序遍历:先访问左子树,再访问右子树,最后访问根节点。
```python
def postorderTraversal(root):
if not root:
return []
res = []
res += self.postorderTraversal(root.left)
res += self.postorderTraversal(root.right)
res.append(root.val)
return res
```
二叉树先序中序后序遍历代码
以下是二叉树先序、中序、后序遍历的代码:
```python
# 先序遍历
def preorderTraversal(root):
if not root:
return []
res = []
stack = [root]
while stack:
node = stack.pop()
res.append(node.val)
if node.right:
stack.append(node.right)
if node.left:
stack.append(node.left)
return res
# 中序遍历
def inorderTraversal(root):
if not root:
return []
res = []
stack = []
while stack or root:
if root:
stack.append(root)
root = root.left
else:
node = stack.pop()
res.append(node.val)
root = node.right
return res
# 后序遍历
def postorderTraversal(root):
if not root:
return []
res = []
stack = [root]
while stack:
node = stack.pop()
res.append(node.val)
if node.left:
stack.append(node.left)
if node.right:
stack.append(node.right)
return res[::-1]
```
阅读全文
相关推荐
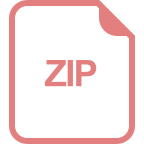
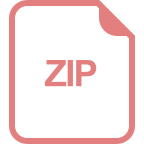
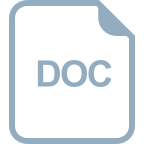
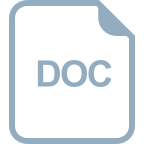
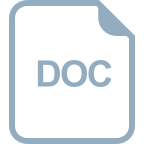






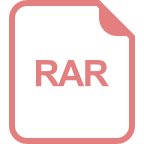
