声明教师类,成员函数有:构造函数,输入成员函数,输出数据信息函数。建立教师对象数组,内放3个教师的数据信息(姓名name、月薪salary),在主函数中将月薪最高的教师信息输出。
时间: 2024-03-17 19:41:05 浏览: 39
好的,我可以帮您写出这个程序。以下是教师类的代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Teacher {
private:
string name;
double salary;
public:
Teacher() : name(""), salary(0.0) {}
Teacher(string nm, double sal) : name(nm), salary(sal) {}
void input() {
cout << "请输入教师姓名:";
getline(cin, name);
cout << "请输入教师月薪:";
cin >> salary;
cin.ignore(); // 忽略输入缓冲区中的回车符
}
void output() {
cout << "教师姓名:" << name << endl;
cout << "教师月薪:" << salary << endl;
}
double getSalary() const {
return salary;
}
};
```
在主函数中,我们可以定义一个教师对象数组,然后循环输入每个教师的信息,同时记录月薪最高的教师的下标。最后再输出月薪最高的教师信息。下面是完整的程序代码:
```c++
#include <iostream>
#include <string>
using namespace std;
class Teacher {
private:
string name;
double salary;
public:
Teacher() : name(""), salary(0.0) {}
Teacher(string nm, double sal) : name(nm), salary(sal) {}
void input() {
cout << "请输入教师姓名:";
getline(cin, name);
cout << "请输入教师月薪:";
cin >> salary;
cin.ignore(); // 忽略输入缓冲区中的回车符
}
void output() {
cout << "教师姓名:" << name << endl;
cout << "教师月薪:" << salary << endl;
}
double getSalary() const {
return salary;
}
};
int main() {
const int MAX_TEACHERS = 3;
Teacher teachers[MAX_TEACHERS];
int maxIndex = 0;
// 输入教师信息
for (int i = 0; i < MAX_TEACHERS; i++) {
cout << "请输入第" << i+1 << "个教师的信息:" << endl;
teachers[i].input();
if (teachers[i].getSalary() > teachers[maxIndex].getSalary()) {
maxIndex = i;
}
}
// 输出月薪最高的教师信息
cout << "月薪最高的教师是:" << endl;
teachers[maxIndex].output();
return 0;
}
```
希望对您有所帮助!
相关推荐
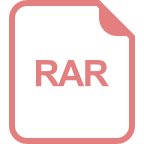
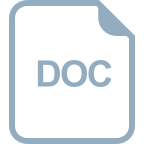
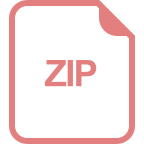







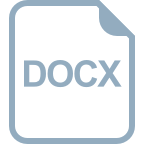
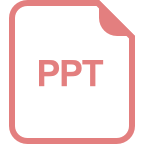
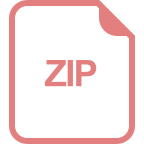
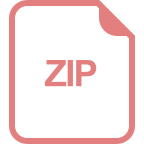
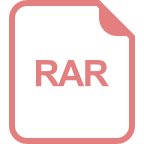
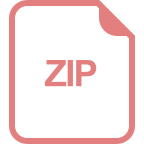
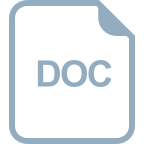
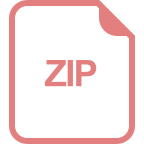