怎么吧结构体传入dll中,代码示例
时间: 2023-11-08 12:17:27 浏览: 39
下面是一个简单的示例代码,演示如何将结构体传递给dll并在dll中使用它:
假设我们有以下结构体定义:
```c
typedef struct MyStruct {
int intValue;
double doubleValue;
char stringValue[100];
} MyStruct;
```
然后,我们在dll中定义一个函数,该函数接受指向结构体的指针,并对其进行操作:
```c
__declspec(dllexport) void myFunction(MyStruct* myStruct) {
// 在这里执行对结构体的操作
printf("intValue: %d, doubleValue: %f, stringValue: %s\n", myStruct->intValue, myStruct->doubleValue, myStruct->stringValue);
}
```
最后,在应用程序中调用dll中的函数,并将指向结构体的指针传递给它:
```c
#include <windows.h>
#include <stdio.h>
typedef struct MyStruct {
int intValue;
double doubleValue;
char stringValue[100];
} MyStruct;
typedef void (__stdcall *MyFunctionPtr)(MyStruct*);
int main() {
HMODULE hDll = LoadLibrary("mydll.dll");
if (hDll == NULL) {
printf("Failed to load DLL\n");
return 1;
}
MyFunctionPtr myFunction = (MyFunctionPtr)GetProcAddress(hDll, "myFunction");
if (myFunction == NULL) {
printf("Failed to get function pointer\n");
return 1;
}
MyStruct myStruct = { 123, 3.14, "Hello World" };
myFunction(&myStruct);
FreeLibrary(hDll);
return 0;
}
```
在这个示例中,我们使用 `LoadLibrary` 和 `GetProcAddress` 函数动态加载并调用dll中的函数。我们还定义了一个指向函数的指针类型 `MyFunctionPtr`,以便在应用程序中调用函数。最后,我们创建了一个结构体 `MyStruct` 的实例,并将其传递给dll中的函数。
相关推荐
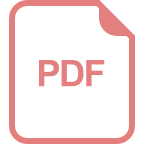
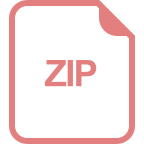














