C++ 获取扩展屏窗口句柄
时间: 2024-01-11 09:51:42 浏览: 400
获取扩展屏幕窗口句柄的方法与获取主屏幕窗口句柄的方法是类似的,只需要在获取窗口句柄之前指定窗口所在的屏幕即可。
以下是一个获取扩展屏幕窗口句柄的示例代码:
```cpp
#include <Windows.h>
HWND GetSecondaryMonitorHWND()
{
HWND hwnd = NULL;
HMONITOR hMonitor = NULL;
// 获取第一个副屏幕的句柄
hMonitor = MonitorFromPoint({0, 0}, MONITOR_DEFAULTTOPRIMARY);
// 如果存在副屏幕,则获取其句柄
if (hMonitor != NULL)
{
MONITORINFOEX monitorInfo = {0};
monitorInfo.cbSize = sizeof(MONITORINFOEX);
GetMonitorInfo(hMonitor, &monitorInfo);
hwnd = FindWindow(NULL, monitorInfo.szDevice);
}
return hwnd;
}
```
该函数通过 `MonitorFromPoint` 函数获取第一个副屏幕的句柄,然后通过 `GetMonitorInfo` 函数获取该屏幕的信息,包括窗口名称等。最后,通过 `FindWindow` 函数查找具有该名称的窗口,并返回其句柄。
相关问题
c++ 创建一个子窗口并根据主窗口句柄在主窗口中居中显示
下面是一个基本的C++程序,它使用Win32 API创建一个子窗口并将其居中放置在主窗口中。这个程序假设你已经有了一个主窗口的句柄(在代码中称为hParent)和一个子窗口的类名(在代码中称为szChildClass)。
```c++
#include <windows.h>
LRESULT CALLBACK ChildWndProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
// Register the child window class
WNDCLASS wc = { 0 };
wc.lpfnWndProc = ChildWndProc;
wc.hInstance = hInstance;
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszClassName = "MyChildClass";
RegisterClass(&wc);
// Create the child window
HWND hChild = CreateWindow("MyChildClass", "My Child Window", WS_VISIBLE | WS_BORDER | WS_CHILD, 0, 0, 200, 200, hParent, NULL, hInstance, NULL);
// Center the child window in the parent window
RECT rcParent, rcChild;
GetWindowRect(hParent, &rcParent);
GetWindowRect(hChild, &rcChild);
int cxParent = rcParent.right - rcParent.left;
int cyParent = rcParent.bottom - rcParent.top;
int cxChild = rcChild.right - rcChild.left;
int cyChild = rcChild.bottom - rcChild.top;
int x = (cxParent - cxChild) / 2 + rcParent.left;
int y = (cyParent - cyChild) / 2 + rcParent.top;
SetWindowPos(hChild, NULL, x, y, 0, 0, SWP_NOSIZE | SWP_NOZORDER);
// Enter the message loop
MSG msg;
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return (int)msg.wParam;
}
LRESULT CALLBACK ChildWndProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam)
{
switch (uMsg)
{
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, uMsg, wParam, lParam);
}
return 0;
}
```
请注意,此代码只是基本的示例。如果你想要更多的控制,你可能需要在创建子窗口之前设置更多的窗口样式和扩展样式,或者在子窗口过程中处理更多的消息。
阅读全文
相关推荐

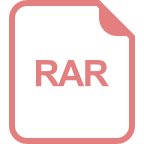













