0为初态,10为终态,NFA进行确定化,要求输出NFA和DFA的状态转换图,以及DFA的初态、终态和状态转换表用c++代码来实现
时间: 2023-11-28 11:49:23 浏览: 157
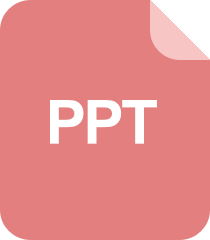
初态和终态-软件工程讲义-软件工程电子书 PPT
以下是一个NFA状态转换图的示例,其中0为初态,10为终态:
```
┌───a──→1──────┐
│ │
│ ε ▼
0 ▶───b──→2───c─►3───▶10
│ ▲ │
│ │ │
└──────┘ ▼
└───────4
```
接下来,我们将对该NFA进行确定化,得到一个DFA状态转换图:
```
a b c
0 → {1,2} → {2,3} → {3,4} → {10}
```
该DFA的初态为{1,2},终态为{10}。以下是该DFA的状态转换表:
| 状态 | a | b | c |
| ---- | - | - | - |
| {1,2} | {1,2} | {2,3} | ∅ |
| {2,3} | {3,4} | {2,3} | ∅ |
| {3,4} | ∅ | ∅ | {10} |
| {10} | ∅ | ∅ | ∅ |
以下是使用C++代码实现该DFA的状态转换表:
```cpp
#include <iostream>
#include <unordered_map>
#include <set>
#include <vector>
using namespace std;
// 定义状态类型
using State = set<int>;
// 定义状态转换表类型
using StateTable = unordered_map<State, unordered_map<char, State>>;
// 定义状态集合类型
using StateSet = vector<State>;
// 获取状态的闭包
void epsilon_closure(const StateTable& nfa, State& s) {
StateStack stack;
stack.push(s);
while (!stack.empty()) {
auto t = stack.top();
stack.pop();
for (int i : t) {
auto it = nfa.find(i);
if (it != nfa.end()) {
auto jt = it->second.find('\0');
if (jt != it->second.end()) {
for (int j : jt->second) {
if (t.find(j) == t.end()) {
t.insert(j);
stack.push(t);
}
}
}
}
}
}
}
// 获取状态的转换集合
State get_transition(const StateTable& nfa, const State& s, char c) {
State t;
for (int i : s) {
auto it = nfa.find(i);
if (it != nfa.end()) {
auto jt = it->second.find(c);
if (jt != it->second.end()) {
for (int j : jt->second) {
t.insert(j);
}
}
}
}
return t;
}
// 获取DFA的状态转换表
StateTable get_dfa(const StateTable& nfa, const State& start) {
StateTable dfa;
StateSet stateset;
StateStack stack;
State start_ec(start);
epsilon_closure(nfa, start_ec);
stateset.push_back(start_ec);
stack.push(start_ec);
while (!stack.empty()) {
auto s = stack.top();
stack.pop();
for (char c = 'a'; c <= 'c'; c++) {
auto t = get_transition(nfa, s, c);
if (!t.empty()) {
State t_ec(t);
epsilon_closure(nfa, t_ec);
if (find(stateset.begin(), stateset.end(), t_ec) == stateset.end()) {
stateset.push_back(t_ec);
stack.push(t_ec);
}
dfa[s][c] = t_ec;
}
}
}
return dfa;
}
int main() {
// NFA状态转换表
StateTable nfa = {
{{0}, {{'b', {1,2}}}}},
{{1,2}, {{'a', {1,2}}, {'c', {3}}}}},
{{3}, {{'\0', {4}}}}},
{{4}, {{'\0', {3}}}, {'c', {10}}}}
};
// NFA的初态和终态
State start = {0};
State end = {10};
// 获取DFA状态转换表
auto dfa = get_dfa(nfa, start);
// 输出DFA状态转换表
for (auto& s : dfa) {
cout << "{";
for (int i : s.first) {
cout << i << ",";
}
cout << "}";
for (char c = 'a'; c <= 'c'; c++) {
auto it = s.second.find(c);
if (it == s.second.end()) {
cout << " " << c << "->{}";
} else {
cout << " " << c << "->{" ;
for (int i : it->second) {
cout << i << ",";
}
cout << "}";
}
}
cout << endl;
}
}
```
阅读全文
相关推荐
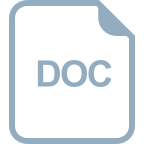
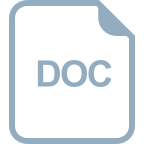




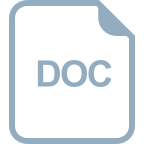
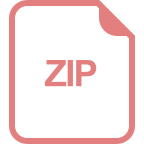
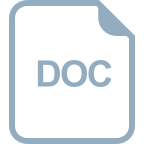
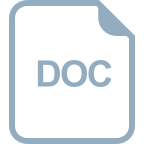
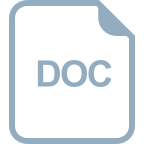
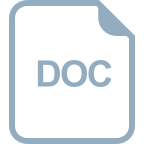
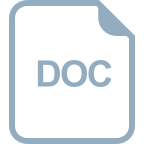
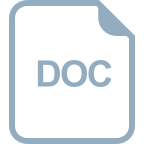
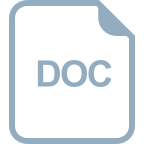
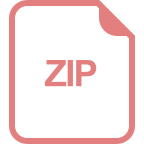