帮我详细讲解这段Java代码public class Product { private int id; private String name; private double price; public Product(int id, String name, double price) { this.id = id; this.name = name; this.price = price; } public int getId() { return id; } public String getName() { return name; } public double getPrice() { return price; } public void setName(String name) { this.name = name; } public void setPrice(double price) { this.price = price; }
时间: 2024-01-11 18:02:18 浏览: 28
这段代码定义了一个Java类Product,包含了三个属性id、name和price以及相应的getter和setter方法。
1. id、name和price属性:
- id:产品的唯一标识符,使用int类型表示。
- name:产品的名称,使用String类型表示。
- price:产品的价格,使用double类型表示。
2. 构造函数:
在Java中,构造函数是一个特殊的方法,用于初始化类的对象。在这个Product类中,构造函数被定义为:
```java
public Product(int id, String name, double price) {
this.id = id;
this.name = name;
this.price = price;
}
```
这个构造函数接收三个参数:id、name和price,并将它们分别赋值给类的属性。关键字 `this` 代表当前对象,用于区分属性和参数名相同的情况。例如,`this.id` 表示当前对象的id属性,而 `id` 则表示传入的参数。
3. getter和setter方法:
getter方法用于获取对象的属性值,setter方法用于设置属性值。在这个Product类中,定义了三个getter方法和两个setter方法:
```java
public int getId() {
return id;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
public void setName(String name) {
this.name = name;
}
public void setPrice(double price) {
this.price = price;
}
```
这些方法都是公共的(使用 public 关键字),因此可以被其他类访问。例如,如果要获取一个Product对象的价格,可以调用 `getPrice()` 方法。如果要修改这个对象的名称,可以调用 `setName(String name)` 方法。
综上所述,这个Product类定义了一个产品对象的基本属性和行为,并提供了访问和修改这些属性的方法。它可以作为其他Java类的一个成员变量或返回值,用于描述和处理产品相关的数据。
相关推荐
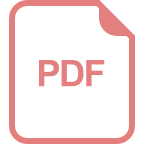
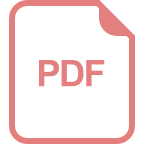
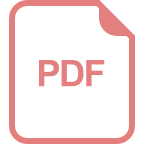














