数据结构C语言在建立一个一元多项式程序的基础上,建立一个函数,计算并返回一元多项式中一共有多少项数据
时间: 2023-05-24 18:03:48 浏览: 131
A:可以使用链表来实现一元多项式,每个节点存储多项式中一项的系数和指数。在计算项数时,遍历整个链表并计数即可,最后返回计数结果。示例代码如下:
```
typedef struct PolyNode *Polynomial;
struct PolyNode {
int coef; // 系数
int expon; // 指数
Polynomial next; // 下一个节点
};
int CountTerms(Polynomial P) {
int count = 0;
while (P) {
count++;
P = P->next;
}
return count;
}
```
其中,P为链表头节点,count为计数器。遍历链表时,每遇到一个节点就将计数器加1。最后返回计数器的值即可。
相关问题
数据结构c语言建立一个一元二次多项式计算并返回多项式中一共有多少项数据
以下是一个基于链表的一元二次多项式的C语言实现,包括了计算和返回多项式中项数的函数:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义一元二次多项式的结构体
typedef struct term {
float coefficient; // 系数
int exponent; // 指数
struct term *next; // 指向下一项的指针
} Term;
// 创建一元二次多项式
Term *createPolynomial() {
Term *head = (Term*) malloc(sizeof(Term)); // 创建头节点
head->next = NULL; // 头节点不存储数据
Term *current = head; // current指向当前节点
int n; // 多项式的项数
printf("请输入一元二次多项式的项数:");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
Term *newTerm = (Term*) malloc(sizeof(Term)); // 创建新节点
printf("请输入第%d项的系数和指数:", i + 1);
scanf("%f %d", &newTerm->coefficient, &newTerm->exponent);
current->next = newTerm; // 当前节点的next指向新节点
current = newTerm; // current指向新节点
}
current->next = NULL; // 最后一个节点的next指向NULL
return head;
}
// 计算一元二次多项式的值
float evaluatePolynomial(Term *head, float x) {
float result = 0;
Term *current = head->next; // 从第一个节点开始遍历
while (current != NULL) {
result += current->coefficient * pow(x, current->exponent);
current = current->next; // 遍历下一个节点
}
return result;
}
// 返回一元二次多项式中项的个数
int countTerms(Term *head) {
int count = 0;
Term *current = head->next; // 从第一个节点开始遍历
while (current != NULL) {
count++;
current = current->next; // 遍历下一个节点
}
return count;
}
int main() {
Term *head = createPolynomial();
printf("一元二次多项式的项数为:%d\n", countTerms(head));
float x;
printf("请输入x的值:");
scanf("%f", &x);
printf("一元二次多项式在x=%.2f处的值为:%.2f\n", x, evaluatePolynomial(head, x));
return 0;
}
```
数据结构c语言,在建立一个一元二次多项式程序的基础上,建立一个函数,可以得到一元多项式中指数最大元素的系数并打出来
以下是一个示例程序,可以用于获取一元多项式中指数最大元素的系数并打印出来。
```c
#include <stdio.h>
#define MAX_TERMS 100 // 多项式项数最大值
typedef struct {
float coef; // 系数
int expon; // 指数
} term;
term terms[MAX_TERMS]; // 多项式数组
int n; // 多项式中的项数
// 添加一个项到多项式中
void attach(float coef, int expon) {
terms[n].coef = coef;
terms[n].expon = expon;
n++;
}
// 获取一元多项式中指数最大元素的系数
float get_max_coef() {
float max_coef = 0.0;
int i;
for (i = 0; i < n; i++) {
if (terms[i].expon > terms[(i+1)%n].expon) {
max_coef = terms[i].coef;
}
}
return max_coef;
}
int main() {
int i;
float coef;
int expon;
// 读取多项式的每一项,添加到数组中
printf("请输入多项式的每一项(按照指数从大到小的顺序输入,以0 0结束):\n");
do {
scanf("%f %d", &coef, &expon);
attach(coef, expon);
} while (expon != 0);
// 获取一元多项式中指数最大元素的系数
float max_coef = get_max_coef();
// 打印出最大系数
printf("一元多项式中指数最大元素的系数为:%.2f\n", max_coef);
return 0;
}
```
运行该程序后,可以根据提示输入一元多项式的每一项,程序将会计算出一元多项式中指数最大元素的系数并打印出来。
注意:上述程序简化了很多情况,比如没有进行错误处理等,仅供参考。
阅读全文
相关推荐
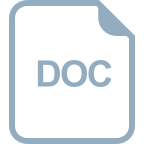













