用c++Polyhedron类只有一个构造函数Polyhedron(const char *path),参数是const char *类型,传递的是目标OFF文件的地址。在这个构造函数中实现对OFF文件的读取。每读一个顶点就new一个Point然后保存到对应的顶点vector数组里面
时间: 2024-02-11 22:05:34 浏览: 101
好的,你的第一个问题是如何使用C++的Polyhedron类构造一个多面体对象?
首先,你需要在你的代码中包含Polyhedron类的头文件:
```c++
#include <CGAL/Polyhedron_3.h>
```
然后,你可以创建一个Polyhedron对象并将其初始化为一个OFF文件中的多面体,方法是调用Polyhedron类的构造函数,并将目标OFF文件的地址作为参数传递:
```c++
CGAL::Polyhedron_3<> polyhedron("path/to/off/file.off");
```
在这个构造函数中,Polyhedron类会读取OFF文件并将其转换为一个多面体对象。在读取过程中,对于每个顶点,Polyhedron类会新建一个Point对象并将其保存到对应的顶点vector数组中。
需要注意的是,这里的Polyhedron类是CGAL库中的类,不同于其他可能存在的同名类。如果你想要进一步操作这个多面体对象,可以查看CGAL的文档或者参考相关的示例代码。
相关问题
Polyhedron类只有一个构造函数Polyhedron(const char *path),参数是const char *类型,传递的是目标OFF文件的地址。在这个构造函数中实现对OFF文件的读取
好的,你的问题是如何在Polyhedron类的构造函数中实现对OFF文件的读取。我可以为你解答。
在Polyhedron类的构造函数中,需要使用C++的文件流(fstream)来读取OFF文件。具体步骤如下:
1. 打开OFF文件:可以使用fstream类的open函数打开OFF文件,可以使用ifstream类打开文件以进行读取。
2. 读取OFF文件的顶点数、面数、边数等信息:OFF文件的第一行包含了模型的基本信息,需要使用getline函数读取该行信息,并解析出模型的顶点数、面数、边数等信息。
3. 读取OFF文件中的顶点信息:OFF文件的第二行到第n+1行包含了模型的顶点坐标信息,需要使用循环结构读取每一个顶点的坐标信息,并将其存储到Polyhedron类的顶点数组中。
4. 读取OFF文件中的面信息:OFF文件的第n+2行到文件结束位置包含了模型的面信息,需要使用循环结构读取每一个面的顶点索引信息,并将其存储到Polyhedron类的面数组中。
5. 关闭OFF文件:使用fstream类的close函数关闭OFF文件。
下面是一个简单的代码示例,可以帮助你更好地理解:
```
#include <fstream>
#include <iostream>
#include <string>
class Polyhedron {
public:
Polyhedron(const char *path) {
// 打开OFF文件
std::ifstream infile;
infile.open(path, std::ios::in);
// 读取OFF文件的基本信息
std::string line;
std::getline(infile, line);
std::istringstream iss(line);
iss >> m_numVertices >> m_numFaces >> m_numEdges;
// 读取OFF文件中的顶点信息
m_vertices.resize(m_numVertices);
for (int i = 0; i < m_numVertices; ++i) {
std::getline(infile, line);
std::istringstream iss(line);
iss >> m_vertices[i].x >> m_vertices[i].y >> m_vertices[i].z;
}
// 读取OFF文件中的面信息
m_faces.resize(m_numFaces);
for (int i = 0; i < m_numFaces; ++i) {
std::getline(infile, line);
std::istringstream iss(line);
int numVertices;
iss >> numVertices;
m_faces[i].resize(numVertices);
for (int j = 0; j < numVertices; ++j) {
iss >> m_faces[i][j];
}
}
// 关闭OFF文件
infile.close();
}
private:
int m_numVertices; // 顶点数
int m_numFaces; // 面数
int m_numEdges; // 边数
std::vector<Point3D> m_vertices; // 顶点坐标
std::vector<std::vector<int>> m_faces; // 面的顶点索引
};
int main() {
Polyhedron p("example.off");
return 0;
}
```
以上是一个简单的示例,你可以根据你的实际需求进行修改。希望能够对你有所帮助!
在c++中,定义Polyhedron类,Polyhedron类只有一个构造函数Polyhedron(const char *path),参数是const char *类型,传递的是目标OFF文件的地址。在这个构造函数中实现对OFF文件的读取。每读一个顶点就new一个Point然后保存到对应的顶点vector数组里面。
好的,你的要求是在C++中定义Polyhedron类,该类具有一个构造函数Polyhedron(const char *path),参数是const char *类型,传递目标OFF文件的地址。在这个构造函数中,我们需要实现对OFF文件的读取,并且每读取一个顶点就new一个Point并将其保存到对应的顶点vector数组中。
首先,我们需要在代码中引入CGAL库的头文件:
```c++
#include <CGAL/Exact_predicates_inexact_constructions_kernel.h>
#include <CGAL/Polyhedron_3.h>
```
然后,我们定义Polyhedron类,其构造函数如下:
```c++
class Polyhedron {
public:
Polyhedron(const char *path) {
CGAL::Polyhedron_3<Kernel> polyhedron;
std::ifstream input(path);
input >> polyhedron;
input.close();
// 遍历顶点,新建Point并保存到对应的顶点vector数组中
for(auto vertex = polyhedron.vertices_begin(); vertex != polyhedron.vertices_end(); ++vertex) {
Point_3 point(vertex->point().x(), vertex->point().y(), vertex->point().z());
vertices.push_back(point);
}
}
private:
typedef CGAL::Exact_predicates_inexact_constructions_kernel Kernel;
typedef CGAL::Polyhedron_3<Kernel> Polyhedron;
typedef Kernel::Point_3 Point_3;
std::vector<Point_3> vertices;
};
```
在这个构造函数中,我们首先创建一个Polyhedron对象,然后打开目标OFF文件并将其读入到这个对象中。在读取过程中,Polyhedron类会自动为每个顶点创建一个Point对象并将其保存到对应的顶点vector数组中。
需要注意的是,我们在Polyhedron类中定义了一个typedef来简化CGAL库中的类型,这样可以更容易地使用这些类型。同时,我们在构造函数中也使用了这些类型来定义Polyhedron对象、Point对象和vertices数组。
最后,我们可以使用如下代码来创建一个Polyhedron对象并传入目标OFF文件的地址:
```c++
Polyhedron polyhedron("path/to/off/file.off");
```
这样,我们就实现了一个Polyhedron类,可以读取OFF文件并保存顶点信息。
阅读全文
相关推荐
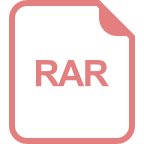




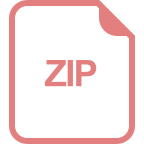
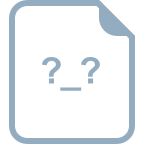
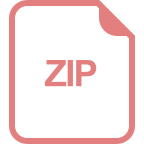
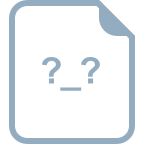
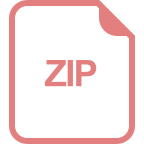