在c++中定义Polyhedron类,Polyhedron类只有一个构造函数Polyhedron(const char *path),参数是const char *类型,传递的是目标OFF文件的地址。在这个构造函数中实现对OFF文件的读取。每读一个顶点就new一个Point然后保存到对应的顶点vector数组里面。所有顶点读完之后再读取面片信息,根据每个面片包含哪些顶点的信息以及上面读取顶点得到的vector数组,就可以得到对应的Point指针,然后加入到Facet的顶点列表中。
时间: 2024-02-11 16:06:02 浏览: 22
可以如下实现 `Polyhedron` 类:
```c++
#include <fstream>
#include <vector>
class Point {
public:
Point(double x, double y, double z) : x_(x), y_(y), z_(z) {}
double getX() const {
return x_;
}
double getY() const {
return y_;
}
double getZ() const {
return z_;
}
private:
double x_;
double y_;
double z_;
};
class Facet {
public:
void addVertex(Point* vertex) {
vertices_.push_back(vertex);
}
private:
std::vector<Point*> vertices_;
};
class Polyhedron {
public:
Polyhedron(const char* path) {
std::ifstream infile(path);
std::string line;
// 读取顶点信息
while (getline(infile, line)) {
if (line == "OFF") {
continue;
}
std::istringstream iss(line);
double x, y, z;
iss >> x >> y >> z;
vertices_.push_back(new Point(x, y, z));
}
// 读取面片信息
int num_facets, num_vertices_per_facet, vertex_index;
infile >> num_facets >> num_vertices_per_facet;
for (int i = 0; i < num_facets; ++i) {
Facet facet;
for (int j = 0; j < num_vertices_per_facet; ++j) {
infile >> vertex_index;
facet.addVertex(vertices_[vertex_index]);
}
facets_.push_back(facet);
}
infile.close();
}
~Polyhedron() {
for (auto vertex : vertices_) {
delete vertex;
}
}
private:
std::vector<Point*> vertices_;
std::vector<Facet> facets_;
};
```
在 `Polyhedron` 的构造函数中,首先使用 `ifstream` 读取目标OFF文件,然后逐行读取文件内容。如果读取到的行是"OFF",则跳过。否则,使用 `istringstream` 解析该行内容,获取顶点的坐标信息,并使用 `new` 运算符动态创建一个 `Point` 对象,并将其加入到顶点列表中。
接着,读取面片信息。首先读取面片个数和每个面片包含的顶点数,然后逐个读取面片的顶点索引,并根据索引获取对应的 `Point` 指针,并将其加入到面片的顶点列表中。最后将面片加入到面片列表中。
需要注意的是,在 `Polyhedron` 的析构函数中,需要手动释放动态分配的 `Point` 对象,防止内存泄漏。
使用 `Polyhedron` 类读取OFF文件的示例代码如下:
```c++
int main() {
Polyhedron polyhedron("example.off");
return 0;
}
```
在示例代码中,首先创建一个 `Polyhedron` 类的对象,并传递目标OFF文件的地址作为构造函数的参数。然后程序会自动读取OFF文件,并将顶点和面片信息存储在类中。
相关推荐
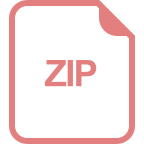







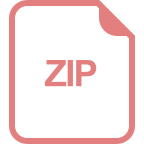
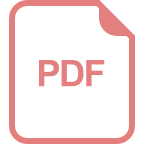
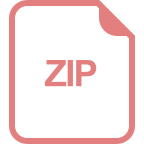