for root, _, fnames in sorted(os.walk(dir)): for fname in fnames: if is_image_file(fname): path = os.path.join(root, fname) images.append(path) return images[:min(max_dataset_size, len(images))]中目录不正确该如何修改
时间: 2024-02-21 15:57:29 浏览: 136
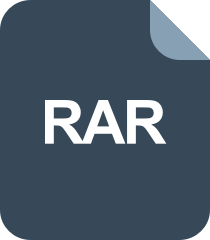
Linked_list_in_Linux.rar_DEMO_linux 链表
如果目录不正确,你需要将该函数中的 `dir` 参数修改为正确的目录路径。假设你希望这个函数搜索的目录是 `/path/to/images`,那么你需要将这个参数修改为 `/path/to/images`,例如:
```
def make_dataset(dir, max_dataset_size=float("inf")):
images = []
for root, _, fnames in sorted(os.walk('/path/to/images')):
for fname in fnames:
if is_image_file(fname):
path = os.path.join(root, fname)
images.append(path)
return images[:min(max_dataset_size, len(images))]
```
这样函数就会在 `/path/to/images` 目录下搜索图片文件并返回一个文件路径列表。
阅读全文
相关推荐
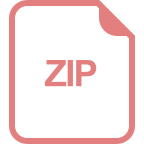
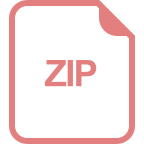

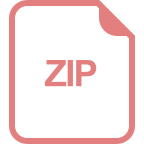
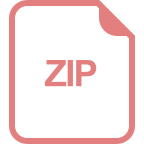
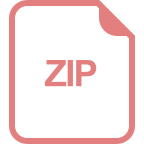
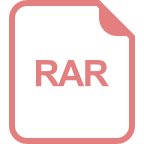
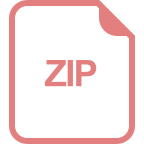
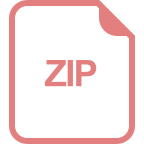
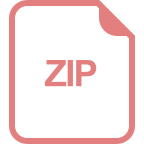
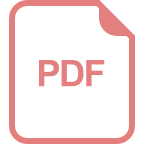
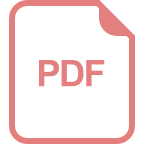
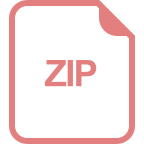
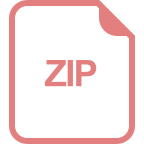
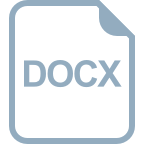
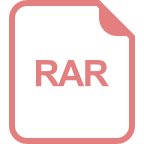
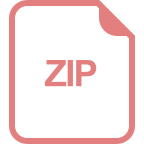