32用库函数实现串口接受数据
时间: 2024-04-29 15:27:07 浏览: 44
很高兴回答你的问题。以下是使用库函数在C语言中实现串口接收数据的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#include <string.h>
int main(){
int fd;
struct termios opt;
/* 打开串口设备 */
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if(fd == -1){
perror("Open Error");
return -1;
}
/* 将终端包括输入、输出波特率设为9600 */
bzero(&opt, sizeof(opt));
cfsetispeed(&opt, B9600);
cfsetospeed(&opt, B9600);
/* 设置为本地连接模式,不接收软件流控制 */
opt.c_cflag |= CLOCAL | CREAD;
opt.c_cflag &=~CSIZE;
opt.c_cflag |= CS8;
/* 设置为无奇偶校验 */
opt.c_cflag &=~PARENB;
opt.c_cflag &=~CSTOPB;
/* 设置超时时间 */
opt.c_cc[VTIME]=0;
opt.c_cc[VMIN]=1;
/* 刷新输入输出缓冲区 */
tcflush(fd,TCIFLUSH);
tcflush(fd,TCOFLUSH);
/* 应用新的配置 */
if(tcsetattr(fd, TCSANOW, &opt)<0){
perror("Set Error");
return -1;
}
while(1){
char buf[256];
memset(buf, 0, sizeof(buf));
/* 等待数据接收 */
int len = read(fd, buf, sizeof(buf));
if(len > 0){
/* 接收到数据,进行处理 */
printf("Received data: %s\n", buf);
}
sleep(1);
}
/* 关闭串口设备 */
close(fd);
return 0;
}
```
该代码将终端波特率设为9600,无奇偶校验,不使用硬件流控制。然后,通过read()函数接收串口传输的数据,然后进行处理。注意,在使用该代码时,需要确认串口设备名称是否正确,以及是否有足够的权限打开该设备。
阅读全文
相关推荐
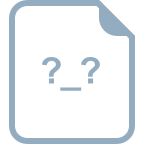


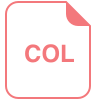




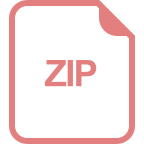







