写一个32位的branch target buffer的verilog代码
时间: 2023-11-27 07:55:00 浏览: 76
以下是一个简单的32位分支目标缓冲器的 Verilog 代码:
```
module branch_target_buffer (
input clk,
input rst,
input [31:0] pc,
input [31:0] target,
input valid,
output [31:0] branch_target
);
parameter BTB_SIZE = 16; // BTB 大小为 16 条记录
parameter INDEX_WIDTH = $clog2(BTB_SIZE); // 索引位宽为 4
reg [31:0] btb_tags [0:BTB_SIZE-1]; // BTB 标记数组
reg [31:0] btb_targets [0:BTB_SIZE-1]; // BTB 目标地址数组
reg [INDEX_WIDTH-1:0] btb_indices [0:BTB_SIZE-1]; // BTB 索引数组
reg [INDEX_WIDTH-1:0] btb_lru [0:BTB_SIZE-1]; // BTB 最近最少使用数组
reg [3:0] btb_hits; // BTB 命中数
// 初始化 BTB
initial begin
for (int i = 0; i < BTB_SIZE; i = i + 1) begin
btb_tags[i] = 32'hffffffff;
btb_targets[i] = 32'hffffffff;
btb_indices[i] = INDEX_WIDTH'hffffffff;
btb_lru[i] = 4'h0;
end
btb_hits = 4'h0;
end
// 更新 BTB
always @(posedge clk) begin
if (rst) begin
for (int i = 0; i < BTB_SIZE; i = i + 1) begin
btb_tags[i] <= 32'hffffffff;
btb_targets[i] <= 32'hffffffff;
btb_indices[i] <= INDEX_WIDTH'hffffffff;
btb_lru[i] <= 4'h0;
end
btb_hits <= 4'h0;
end else begin
integer match_index = -1;
for (int i = 0; i < BTB_SIZE; i = i + 1) begin
if (btb_tags[i] == pc[31:INDEX_WIDTH]) begin // 标记匹配
match_index = i;
break;
end
end
if (valid && match_index != -1) begin // 命中
btb_hits <= btb_hits + 1;
btb_targets[match_index] <= target; // 更新目标地址
btb_lru[match_index] <= 4'hf; // 最近使用
end else begin // 未命中
integer lru_index = 0;
for (int i = 1; i < BTB_SIZE; i = i + 1) begin
if (btb_lru[i] < btb_lru[lru_index]) begin // 找到最近最少使用的索引
lru_index = i;
end
end
btb_tags[lru_index] <= pc[31:INDEX_WIDTH]; // 更新标记
btb_targets[lru_index] <= target; // 更新目标地址
btb_lru[lru_index] <= 4'hf; // 最近使用
end
end
end
// 输出分支目标地址
assign branch_target = btb_hits == 4'h0 ? 32'hffffffff : btb_targets[match_index];
endmodule
```
此代码中使用了一个大小为 16 的 BTB,每个 BTB 条目包含标记、目标地址、索引和最近最少使用信息。当给定一个 PC 地址和一个分支目标地址时,BTB 将检查是否有与给定标记匹配的条目。如果有,则 BTB 命中并返回相应的目标地址;否则,BTB 插入一个新的条目,将其标记设置为给定标记,将其目标地址设置为给定目标地址,并更新最近最少使用信息。当 BTB 满时,将使用最近最少使用信息来确定要替换的条目。
阅读全文
相关推荐
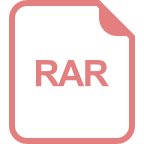
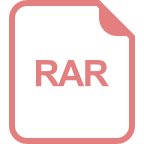




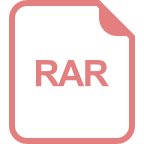
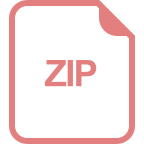
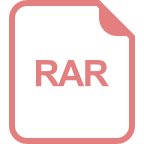
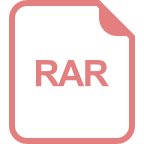
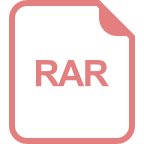




