C语言编写一个复数支持++
时间: 2023-05-23 12:06:21 浏览: 120
A:
在C语言中,复数可以用结构体来表示。这个结构体中包含两个浮点数,一个表示实部,一个表示虚部。
首先,定义一个复数结构体:
```c
struct complex {
float real;
float imag;
};
```
接下来,我们可以为这个结构体定义一些常用的操作函数,比如加法、减法、乘法、除法等:
```c
// 加法
struct complex add(struct complex x, struct complex y) {
struct complex z;
z.real = x.real + y.real;
z.imag = x.imag + y.imag;
return z;
}
// 减法
struct complex sub(struct complex x, struct complex y) {
struct complex z;
z.real = x.real - y.real;
z.imag = x.imag - y.imag;
return z;
}
// 乘法
struct complex mul(struct complex x, struct complex y) {
struct complex z;
z.real = x.real * y.real - x.imag * y.imag;
z.imag = x.real * y.imag + x.imag * y.real;
return z;
}
// 除法
struct complex div(struct complex x, struct complex y) {
struct complex z;
float s = y.real * y.real + y.imag * y.imag;
z.real = (x.real * y.real + x.imag * y.imag) / s;
z.imag = (x.imag * y.real - x.real * y.imag) / s;
return z;
}
```
这样,我们就可以使用这些操作函数进行复数的加减乘除操作了:
```c
int main() {
struct complex a = {1.0, 2.0};
struct complex b = {3.0, 4.0};
struct complex c = add(a, b);
printf("a + b = %f + %fi\n", c.real, c.imag);
struct complex d = sub(a, b);
printf("a - b = %f + %fi\n", d.real, d.imag);
struct complex e = mul(a, b);
printf("a * b = %f + %fi\n", e.real, e.imag);
struct complex f = div(a, b);
printf("a / b = %f + %fi\n", f.real, f.imag);
return 0;
}
```
输出结果:
```
a + b = 4.000000 + 6.000000i
a - b = -2.000000 - 2.000000i
a * b = -5.000000 + 10.000000i
a / b = 0.440000 + 0.080000i
```
阅读全文
相关推荐
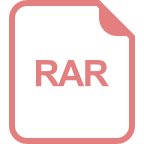

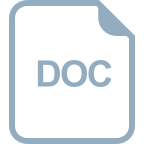
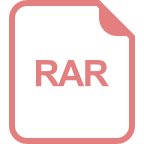
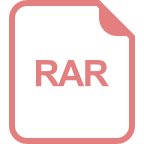











