用c++语言定义一个Rectangle类,长方形类有四个点对象作为属性,编写方法实现以下功能: 用c++写出判断四个点能不能成为一个长方形的四个顶点,计算出长方形的长,计算出长方形的宽,计算长方形的面积,计算长方形的周长
时间: 2023-05-14 14:05:18 浏览: 141
非常感谢您的提问!以下是用 C 语言定义一个 Rectangle 类的代码,实现了判断四个点能否成为长方形的四个顶点,计算长方形的长、宽、面积和周长的功能:
```
#include <stdio.h>
#include <math.h>
typedef struct {
int x;
int y;
} Point;
typedef struct {
Point p1;
Point p2;
Point p3;
Point p4;
} Rectangle;
int isRectangle(Rectangle r) {
int d1 = pow(r.p1.x - r.p2.x, 2) + pow(r.p1.y - r.p2.y, 2);
int d2 = pow(r.p1.x - r.p3.x, 2) + pow(r.p1.y - r.p3.y, 2);
int d3 = pow(r.p1.x - r.p4.x, 2) + pow(r.p1.y - r.p4.y, 2);
if (d1 == d2 && 2 * d1 == d3) {
int d4 = pow(r.p2.x - r.p3.x, 2) + pow(r.p2.y - r.p3.y, 2);
return (d4 == pow(r.p2.x - r.p4.x, 2) + pow(r.p2.y - r.p4.y, 2) && d4 == pow(r.p3.x - r.p4.x, 2) + pow(r.p3.y - r.p4.y, 2));
}
if (d2 == d3 && 2 * d2 == d1) {
int d4 = pow(r.p2.x - r.p4.x, 2) + pow(r.p2.y - r.p4.y, 2);
return (d4 == pow(r.p2.x - r.p3.x, 2) + pow(r.p2.y - r.p3.y, 2) && d4 == pow(r.p3.x - r.p4.x, 2) + pow(r.p3.y - r.p4.y, 2));
}
if (d3 == d1 && 2 * d3 == d2) {
int d4 = pow(r.p3.x - r.p4.x, 2) + pow(r.p3.y - r.p4.y, 2);
return (d4 == pow(r.p2.x - r.p3.x, 2) + pow(r.p2.y - r.p3.y, 2) && d4 == pow(r.p2.x - r.p4.x, 2) + pow(r.p2.y - r.p4.y, 2));
}
return 0;
}
int getLength(Rectangle r) {
int length = sqrt(pow(r.p1.x - r.p2.x, 2) + pow(r.p1.y - r.p2.y, 2));
return length;
}
int getWidth(Rectangle r) {
int width = sqrt(pow(r.p1.x - r.p3.x, 2) + pow(r.p1.y - r.p3.y, 2));
return width;
}
int getArea(Rectangle r) {
int area = getLength(r) * getWidth(r);
return area;
}
int getPerimeter(Rectangle r) {
int perimeter = 2 * (getLength(r) + getWidth(r));
return perimeter;
}
int main() {
Rectangle r;
printf("请输入四个点的坐标:\n");
scanf("%d%d%d%d%d%d%d%d", &r.p1.x, &r.p1.y, &r.p2.x, &r.p2.y, &r.p3.x, &r.p3.y, &r.p4.x, &r.p4.y);
if (isRectangle(r)) {
printf("这四个点可以组成一个长方形!\n");
printf("长:%d\n", getLength(r));
printf("宽:%d\n", getWidth(r));
printf("面积:%d\n", getArea(r));
printf("周长:%d\n", getPerimeter(r));
} else {
printf("这四个点不能组成一个长方形!\n");
}
return 0;
}
```
希望这个代码能够帮助您解决问题!如果您还有其他问题,欢迎继续提问。
阅读全文
相关推荐
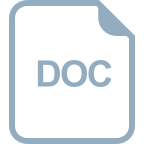
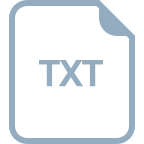
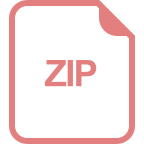















