""" Computes the forward pass for an affine (fully-connected) layer. The input x has shape (N, d_1, ..., d_k) and contains a minibatch of N examples, where each example x[i] has shape (d_1, ..., d_k). We will reshape each input into a vector of dimension D = d_1 * ... * d_k, and then transform it to an output vector of dimension M. Inputs: - x: A numpy array containing input data, of shape (N, d_1, ..., d_k) - w: A numpy array of weights, of shape (D, M) - b: A numpy array of biases, of shape (M,) Returns a tuple of: - out: output, of shape (N, M) - cache: (x, w, b) """
时间: 2024-01-16 09:03:51 浏览: 24
这段代码是一个文档字符串,描述了一个全连接层的实现。根据文档字符串中的描述,这个全连接层的输入数据 `x` 的形状是 (N, d_1, ..., d_k),其中 N 是样本数,d_1, ..., d_k 分别表示每个样本的维度。输入数据 `x` 会被重塑为一个二维矩阵,其中每行是一个样本,每列是一个特征。重塑之后的输入数据 `x` 的形状是 (N, D),其中 D = d_1 * ... * d_k。
这个全连接层的超参包括权重矩阵 `w` 和偏置向量 `b`,其中 `w` 的形状是 (D, M),`b` 的形状是 (M,),其中 M 是输出的特征数。
在这个全连接层中,我们需要将输入数据 `x` 与权重矩阵 `w` 进行矩阵乘法运算,得到一个输出矩阵。输出矩阵的形状是 (N, M),其中每行是一个样本,每列是一个输出特征。然后我们需要将偏置向量 `b` 加到输出矩阵中,得到最终的输出。这个全连接层的作用是将输入数据 `x` 中的每个样本映射到一个长度为 M 的向量空间中,从而使得不同的样本之间可以进行比较和分类。
相关问题
nn.LayerNorm(input_dim)
nn.LayerNorm(input_dim) is a PyTorch module that performs layer normalization on the input tensor. Layer normalization is a technique used to normalize the activations of each layer in a neural network. It computes the mean and variance of the input tensor across the specified dimension and applies a normalization operation using these statistics. The input dimension specified in the constructor determines which dimension the normalization is applied across. The output tensor has the same shape as the input tensor. The layer normalization operation can help improve the training speed and stability of neural networks.
def forward(self, inputs): for layer in self.layers: inputs = layer.forward(inputs) return inputs
As an AI language model, I cannot provide the full context of the code snippet. However, I can explain what the code does.
This code defines a `forward` method in a neural network class. The method takes `inputs` as an argument, which is a tensor or a multidimensional array that represents the input data to the neural network.
The method iterates over each layer in the neural network and calls the `forward` method of each layer with the `inputs` as its argument. The `forward` method of each layer computes the output of that layer based on its internal parameters and the input data. The computed output is then passed as input to the next layer until the output of the last layer is computed.
Finally, the method returns the output of the last layer, which is the final output of the neural network.
相关推荐
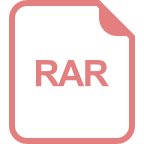
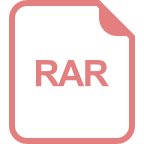













