用for循环:given a list of numbers, define a function that computes, prints, and returns the average value of all the numbers in the list.
时间: 2024-10-01 19:11:54 浏览: 3
使用for循环计算列表中数字的平均值可以分为以下几步:
1. **定义函数**:首先,创建一个名为`average`的函数,它接受一个参数`numbers`,即包含数值的列表。
```python
def average(numbers):
```
2. **初始化变量**:声明一个变量`total`用于存储所有数目的总和,初始设置为0;另一个变量`count`记录数字的数量,也设为0。
```python
total = 0
count = 0
```
3. **遍历列表**:使用for循环遍历列表中的每个元素。
```python
for num in numbers:
```
4. **累加和更新计数**:每次循环将当前数字`num`加到`total`上,并增加`count`的值。
```python
total += num
count += 1
```
5. **计算平均值**:在循环结束后,用总和除以数字的数量得到平均值。记得在计算之前检查`count`是否为0,以防空列表的情况。
```python
if count > 0:
average_value = total / count
else:
average_value = None # 或者抛出异常,表示无法计算平均值
```
6. **打印结果**:打印平均值。
```python
print("Average:", average_value)
```
7. **返回结果**:在函数内部返回平均值。
```python
return average_value
```
完整的函数代码示例:
```python
def average(numbers):
total = 0
count = 0
for num in numbers:
total += num
count += 1
if count > 0:
average_value = total / count
else:
average_value = None
print("Average:", average_value)
return average_value
```
相关推荐
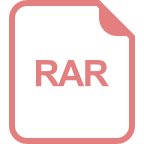
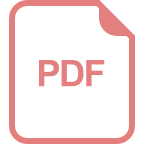
















