#include<stdio.h> #include<stdlib.h> #include<string.h> #define MAXSIZE 100 #define OK 1 #define ERROR 0 #define OVERFLOW -2 #define INFEASIBLE -1 typedef struct {/* 栈类定义 */ char data[MAXSIZE]; int top; }SqStack; typedef struct { /* 队列类定义 */ char data[MAXSIZE]; int front;/* 队首指针 */ int rear;/* 队尾指针 */ }SqQueue; void InitSqStack(SqStack *s) { /* 初始化栈,将栈置空 */ s->top=0; /* 令top为0表示栈为空 */ } int InitSqQueue(SqQueue *q) {/* 初始化循环队列,将队列置为空 */ q=(SqQueue *)malloc(sizeof(SqQueue));/* 分配队列的存储空间 */ if(q==NULL){ return 0; } (q)->front=(q)->rear=0;/* 令front为0 */ return 1; } int PushStack(SqStack *s,char e) { /* 将元素e压入到栈S中 */ if(s->top==MAXSIZE)/* 栈满则操作失败 */ return 0; s->data[s->top]=e; s->top++; return 1; } int PushSqQueue(SqQueue *q,char e) {/* 将元素e压入到队列Q中 */ if(q->front==(q->rear+1)%MAXSIZE) /* 队列满则操作失败 */ return 0; q->data[q->rear]=e; q->rear=(q->rear+1)%MAXSIZE; return 1; } int PopStack(SqStack *s,char *e) {/* 将栈S中的栈顶元素出栈 */ if(s->top==0) /* 栈空则操作失败 */ return 0; s->top--; *e=s->data[s->top]; return 1; } int PopQueue(SqQueue *q,char *e) { /* 将队列Q中的队首元素删除 */ if(q->front==q->rear) /* 队列空则操作失败 */ return 0; *e=q->data[q->front]; q->front=(q->front+1)%MAXSIZE; return 1; } void Print(SqStack *s){ while(s->top!=0){ char x; PopStack(s,&x); printf("%c",x); } } void EditString(){ SqStack s; InitSqStack(&s); char c; while((c=getchar())!='\n'){ if(c=='#'){ char x; PopStack(&s,&x); } else if(c=='@'){ InitSqStack(&s); } else{ PushStack(&s,c); } } Print(&s); } void ReadString() { SqQueue q; InitSqQueue(&q); char c; while((c=getchar())!='\n'){ PushSqQueue(&q,c); } while(q.front!=q.rear){ char x; PopQueue(&q,&x); printf("%c",x); } } int main() { SqQueue q; if(InitSqQueue(&q) == 0){return 0;} EditString(); ReadString(); free(q); return 0; }free处的错误是什么,怎么改正
时间: 2024-02-23 17:00:22 浏览: 53
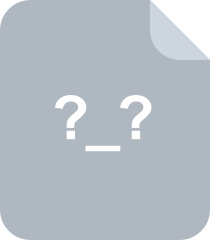
StudentInformation
在main函数中,使用了free(q)来释放动态分配的SqQueue空间,但这里应该使用free(&q),即应该释放指向SqQueue的指针q的空间。修改后代码如下:
```
int main() {
SqQueue *q; // 声明SqQueue类型的指针
if(InitSqQueue(q) == 0){return 0;}
EditString();
ReadString();
free(q); // 释放指针q指向的空间
return 0;
}
```
阅读全文
相关推荐
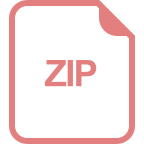
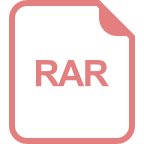
















