7-2 Summation from 1 to n using recursion. 分数 10 全屏浏览题目 作者 Jinlan Xu 单位 杭州电子科技大学 Write a program in C to calculate the sum of numbers from 1 to n using recursion. Input: Input a positve integer n. Output : Compute the summation of 1+2+...+n. Sample of Input: 5 Sample of Output: 15
时间: 2024-02-25 07:57:46 浏览: 19
好的,我明白了你的问题。以下是一个使用递归计算从1到n的总和的C程序:
```c
#include <stdio.h>
int sum(int n);
int main() {
int n, result;
printf("Input a positive integer n: ");
scanf("%d", &n);
result = sum(n);
printf("Summation from 1 to %d is %d\n", n, result);
return 0;
}
int sum(int n) {
if (n == 0) {
return 0;
} else {
return n + sum(n - 1);
}
}
```
这个程序中,我们定义了一个名为“sum”的递归函数,它接受一个整数n作为参数,并返回从1到n的总和。在函数中,我们首先检查n是否为0,如果是,我们返回0作为基本情况。否则,我们通过递归调用sum函数来计算n-1的总和,并将结果与n相加,以获得n的总和。最后,在主函数中,我们获取用户输入的整数n,并将其作为参数传递给sum函数,以计算从1到n的总和,并将结果打印到控制台上。
希望这个程序能够帮助您解决问题!
相关问题
Write a program in C to calculate the sum of numbers from 1 to n using recursion. Input: Input a positve integer n. Output : Compute the summation of 1+2+...+n. Sample of Input: 5 Sample of Output: 15
Sure, I can help you with that. Here's the C program to calculate the sum of numbers from 1 to n using recursion:
```c
#include <stdio.h>
int sum(int n);
int main() {
int n, result;
printf("Enter a positive integer: ");
scanf("%d", &n);
result = sum(n);
printf("The sum of numbers from 1 to %d is %d.\n", n, result);
return 0;
}
int sum(int n) {
if (n == 0) {
return 0;
} else {
return n + sum(n-1);
}
}
```
Explanation:
The program takes a positive integer `n` as input from the user and calls the `sum()` function with `n` as argument.
The `sum()` function is a recursive function that takes an integer `n` as input and returns the sum of numbers from 1 to `n`.
If `n` is 0, the function returns 0. Otherwise, it adds `n` to the sum of numbers from 1 to `n-1` by calling itself recursively with `n-1` as argument.
Finally, the program outputs the result, which is the sum of numbers from 1 to `n`.
详细解释一下这段话The direct summation causes that the response maximum of the feature group is positively related to the number of fea- tures in the group. Next, the summation is normalized to relieve this relativity
这段话是在讨论特征组的响应最大值与组中特征数量之间的关系。其中,“direct summation”指的是直接将特征组中各个特征的值相加得到的结果,这种方法会导致特征组的响应最大值与组中特征数量呈正相关关系,也就是说,特征数量越多,响应最大值就越大。
为了消除这种相关性,需要对求和结果进行归一化处理。归一化可以将求和结果映射到一个固定的范围内,比如[0,1],这样就可以消除特征数量对响应最大值的影响。具体而言,归一化可以通过Z-score标准化、最小-最大规范化等方法实现,其中最小-最大规范化是将所有特征值线性映射到[0,1]区间内,公式为:(x-min)/(max-min)。
因此,这段话的意思是:通过直接求和得到的特征组响应最大值与组中特征数量呈正相关关系,为了消除这种相关性,需要对求和结果进行归一化处理。
相关推荐
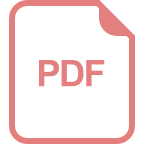
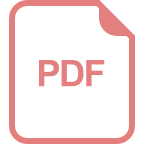
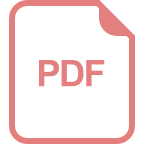













